mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-14 10:04:52 +00:00
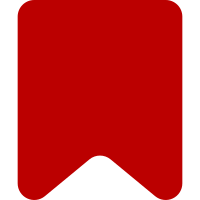
After catching the REST exception, just throw an API exception. Remove the step inbetween where we built a response array. Bonus: * Remove tests that only tested this array building. * Remove the unused 'code' from successful responses, and update documentation of return value shapes. * Remove impossible check for `$ex instanceof LocalizedException`. After moving the code into one place, it's obvious that it can't happen, since LocalizedException doesn't inherit from HttpException. Bug: T341613 Change-Id: I31370efeed9d5283b53eafca102b2f1efc811d27
63 lines
2.1 KiB
PHP
63 lines
2.1 KiB
PHP
<?php
|
|
namespace MediaWiki\Extension\VisualEditor;
|
|
|
|
use MediaWiki\Page\PageIdentity;
|
|
use MediaWiki\Revision\RevisionRecord;
|
|
use Wikimedia\Bcp47Code\Bcp47Code;
|
|
|
|
interface ParsoidClient {
|
|
|
|
/**
|
|
* Request page HTML
|
|
*
|
|
* @param RevisionRecord $revision Page revision
|
|
* @param Bcp47Code|null $targetLanguage Desired output language
|
|
*
|
|
* @return array An array mimicking a RESTbase server's response, with keys: 'headers' and 'body'
|
|
* @phan-return array{body:string,headers:array<string,string>}
|
|
*/
|
|
public function getPageHtml( RevisionRecord $revision, ?Bcp47Code $targetLanguage ): array;
|
|
|
|
/**
|
|
* Transform HTML to wikitext via Parsoid
|
|
*
|
|
* @param PageIdentity $page The page the content belongs to
|
|
* @param Bcp47Code $targetLanguage The desired output language
|
|
* @param string $html The HTML of the page to be transformed
|
|
* @param ?int $oldid What oldid revision, if any, to base the request from (default: `null`)
|
|
* @param ?string $etag The ETag to set in the HTTP request header
|
|
*
|
|
* @return array An array mimicking a RESTbase server's response, with keys: 'headers' and 'body'
|
|
* @phan-return array{body:string,headers:array<string,string>}
|
|
*/
|
|
public function transformHTML(
|
|
PageIdentity $page,
|
|
Bcp47Code $targetLanguage,
|
|
string $html,
|
|
?int $oldid,
|
|
?string $etag
|
|
): array;
|
|
|
|
/**
|
|
* Transform wikitext to HTML via Parsoid.
|
|
*
|
|
* @param PageIdentity $page The page the content belongs to
|
|
* @param Bcp47Code $targetLanguage The desired output language
|
|
* @param string $wikitext The wikitext fragment to parse
|
|
* @param bool $bodyOnly Whether to provide only the contents of the `<body>` tag
|
|
* @param ?int $oldid What oldid revision, if any, to base the request from (default: `null`)
|
|
* @param bool $stash Whether to stash the result in the server-side cache (default: `false`)
|
|
*
|
|
* @return array An array mimicking a RESTbase server's response, with keys: 'headers' and 'body'
|
|
* @phan-return array{body:string,headers:array<string,string>}
|
|
*/
|
|
public function transformWikitext(
|
|
PageIdentity $page,
|
|
Bcp47Code $targetLanguage,
|
|
string $wikitext,
|
|
bool $bodyOnly,
|
|
?int $oldid,
|
|
bool $stash
|
|
): array;
|
|
}
|