mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 11:46:55 +00:00
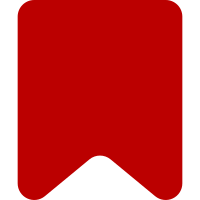
Why: Because they are the approved standard by TC39 and Ecma for JavaScript modules. Changes: * Wrap mw-node-qunit in run.js to register babel to transpile modules for node v6 * Change all sources in src/ to use ES modules * Change constants.js to be able to run without jQuery.bracketedDevicePixelRatio given ES modules are hoisted to the top by spec so we can't patch globals before importing it * Change all tests in tests/node-qunit/ to use ES modules * Drop usage of mock-require given ES modules are easy to stub with sinon Additional changes: * Rename tests/node-qunit/renderer.js to renderer.test.js to follow the convention of all the other files * Make npm run test:node run only .test.js test files so that it doesn't run the stubs.js or run.js file. Bug: T171951 Change-Id: I17a0b76041d5e2fd18e2d54950d9d7c0db99a941
245 lines
4.4 KiB
JavaScript
245 lines
4.4 KiB
JavaScript
import preview from '../../../src/reducers/preview';
|
|
|
|
QUnit.module( 'ext.popups/reducers#preview', {
|
|
beforeEach: function () {
|
|
this.el = 'active link';
|
|
}
|
|
} );
|
|
|
|
QUnit.test( '@@INIT', function ( assert ) {
|
|
var state = preview( undefined, { type: '@@INIT' } );
|
|
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual(
|
|
state,
|
|
{
|
|
enabled: undefined,
|
|
activeLink: undefined,
|
|
activeEvent: undefined,
|
|
activeToken: '',
|
|
shouldShow: false,
|
|
isUserDwelling: false
|
|
}
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'BOOT', function ( assert ) {
|
|
var action = {
|
|
type: 'BOOT',
|
|
isEnabled: true
|
|
};
|
|
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
enabled: true
|
|
},
|
|
'It should set whether or not previews are enabled.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'SETTINGS_CHANGE', function ( assert ) {
|
|
var action = {
|
|
type: 'SETTINGS_CHANGE',
|
|
enabled: true
|
|
};
|
|
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
enabled: true
|
|
},
|
|
'It should set whether or not previews are enabled when settings change.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'LINK_DWELL initializes the state for a new link', function ( assert ) {
|
|
var action = {
|
|
type: 'LINK_DWELL',
|
|
el: this.el,
|
|
event: {},
|
|
token: '1234567890'
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
activeLink: action.el,
|
|
activeEvent: action.event,
|
|
activeToken: action.token,
|
|
shouldShow: false,
|
|
isUserDwelling: true
|
|
},
|
|
'It should set active link and event as well as interaction info and hide the preview.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'LINK_DWELL on an active link only updates dwell state', function ( assert ) {
|
|
var action = {
|
|
type: 'LINK_DWELL',
|
|
el: this.el,
|
|
event: {},
|
|
token: '1234567890'
|
|
},
|
|
state = {
|
|
activeLink: this.el,
|
|
isUserDwelling: false
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
activeLink: this.el,
|
|
isUserDwelling: true
|
|
},
|
|
'It should only set isUserDwelling to true'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'ABANDON_END', function ( assert ) {
|
|
var action = {
|
|
type: 'ABANDON_END',
|
|
token: 'bananas'
|
|
},
|
|
state = {
|
|
activeToken: 'bananas',
|
|
isUserDwelling: false
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
activeLink: undefined,
|
|
activeToken: undefined,
|
|
activeEvent: undefined,
|
|
fetchResponse: undefined,
|
|
isUserDwelling: false,
|
|
shouldShow: false
|
|
},
|
|
'ABANDON_END should hide the preview and reset the interaction info.'
|
|
);
|
|
|
|
// ---
|
|
|
|
state = {
|
|
activeToken: 'apples',
|
|
isUserDwelling: true
|
|
};
|
|
|
|
assert.equal(
|
|
preview( state, action ),
|
|
state,
|
|
'ABANDON_END should NOOP if the current interaction has changed.'
|
|
);
|
|
|
|
// ---
|
|
|
|
state = {
|
|
activeToken: 'bananas',
|
|
isUserDwelling: true
|
|
};
|
|
|
|
assert.equal(
|
|
preview( state, action ),
|
|
state,
|
|
'ABANDON_END should NOOP if the user is dwelling on the preview.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'FETCH_COMPLETE', function ( assert ) {
|
|
var token = '1234567890',
|
|
state = {
|
|
activeToken: token,
|
|
isUserDwelling: true
|
|
},
|
|
action = {
|
|
type: 'FETCH_COMPLETE',
|
|
token: token,
|
|
result: {}
|
|
};
|
|
|
|
assert.expect( 3 );
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
// Previous state.
|
|
activeToken: state.activeToken,
|
|
isUserDwelling: true,
|
|
|
|
fetchResponse: action.result,
|
|
shouldShow: true
|
|
},
|
|
'It should store the result and signal that a preview should be rendered.'
|
|
);
|
|
|
|
// ---
|
|
|
|
state = preview( state, {
|
|
type: 'ABANDON_START',
|
|
token: token
|
|
} );
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
activeToken: token,
|
|
isUserDwelling: false, // Set when ABANDON_START is reduced.
|
|
|
|
fetchResponse: action.result,
|
|
shouldShow: false
|
|
},
|
|
'It shouldn\'t signal that a preview should be rendered if the user has abandoned the link since the gateway request was made.'
|
|
);
|
|
|
|
// ---
|
|
|
|
action = {
|
|
type: 'FETCH_COMPLETE',
|
|
token: 'banana',
|
|
result: {}
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
state,
|
|
'It should NOOP if the user has interacted with another link since the gateway request was made.'
|
|
);
|
|
|
|
} );
|
|
|
|
QUnit.test( 'PREVIEW_DWELL', function ( assert ) {
|
|
var action = {
|
|
type: 'PREVIEW_DWELL'
|
|
};
|
|
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
isUserDwelling: true
|
|
},
|
|
'It should mark the preview as being dwelled on.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'ABANDON_START', function ( assert ) {
|
|
var action = {
|
|
type: 'ABANDON_START'
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
isUserDwelling: false
|
|
},
|
|
'ABANDON_START should mark the preview having been abandoned.'
|
|
);
|
|
} );
|