mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-09-25 03:08:16 +00:00
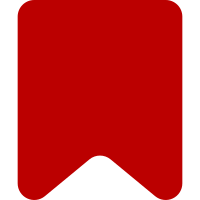
The variable was declared in the "if" branch but also used in the "else" one. This caused the rules textarea to not have the readonly attribute if the user wasn't allowed and CodeEditor wasn't installed. Change-Id: I2bf69dc0f2d24efac41d1ac6100ed7e286e3afa4
256 lines
6.5 KiB
PHP
256 lines
6.5 KiB
PHP
<?php
|
|
|
|
use Wikimedia\Rdbms\IDatabase;
|
|
|
|
abstract class AbuseFilterView extends ContextSource {
|
|
public $mFilter, $mHistoryID, $mSubmit, $mPage, $mParams;
|
|
|
|
/**
|
|
* @var \MediaWiki\Linker\LinkRenderer
|
|
*/
|
|
protected $linkRenderer;
|
|
|
|
/**
|
|
* @param SpecialAbuseFilter $page
|
|
* @param array $params
|
|
*/
|
|
public function __construct( $page, $params ) {
|
|
$this->mPage = $page;
|
|
$this->mParams = $params;
|
|
$this->setContext( $this->mPage->getContext() );
|
|
$this->linkRenderer = $page->getLinkRenderer();
|
|
}
|
|
|
|
/**
|
|
* @param string $subpage
|
|
* @return Title
|
|
*/
|
|
public function getTitle( $subpage = '' ) {
|
|
return $this->mPage->getPageTitle( $subpage );
|
|
}
|
|
|
|
/**
|
|
* Function to show the page
|
|
*/
|
|
abstract public function show();
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function canEdit() {
|
|
return (
|
|
!$this->getUser()->isBlocked() &&
|
|
$this->getUser()->isAllowed( 'abusefilter-modify' )
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function canEditGlobal() {
|
|
return $this->getUser()->isAllowed( 'abusefilter-modify-global' );
|
|
}
|
|
|
|
/**
|
|
* Whether the user can edit the given filter.
|
|
*
|
|
* @param object $row Filter row
|
|
*
|
|
* @return bool
|
|
*/
|
|
public function canEditFilter( $row ) {
|
|
return (
|
|
$this->canEdit() &&
|
|
!( isset( $row->af_global ) && $row->af_global == 1 && !$this->canEditGlobal() )
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @param string $rules
|
|
* @param string $textName
|
|
* @param bool $addResultDiv
|
|
* @param bool $externalForm
|
|
* @return string
|
|
*/
|
|
public function buildEditBox(
|
|
$rules,
|
|
$textName = 'wpFilterRules',
|
|
$addResultDiv = true,
|
|
$externalForm = false
|
|
) {
|
|
$this->getOutput()->enableOOUI();
|
|
|
|
// Rules are in English
|
|
$editorAttrib = [ 'dir' => 'ltr' ];
|
|
|
|
$noTestAttrib = [];
|
|
if ( !$this->getUser()->isAllowed( 'abusefilter-modify' ) ) {
|
|
$noTestAttrib['disabled'] = 'disabled';
|
|
$addResultDiv = false;
|
|
}
|
|
|
|
$rules = rtrim( $rules ) . "\n";
|
|
$canEdit = $this->canEdit();
|
|
|
|
if ( ExtensionRegistry::getInstance()->isLoaded( 'CodeEditor' ) ) {
|
|
$editorAttrib['name'] = 'wpAceFilterEditor';
|
|
$editorAttrib['id'] = 'wpAceFilterEditor';
|
|
$editorAttrib['class'] = 'mw-abusefilter-editor';
|
|
|
|
$switchEditor =
|
|
new OOUI\ButtonWidget(
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-switch-editor' )->text(),
|
|
'id' => 'mw-abusefilter-switcheditor'
|
|
] + $noTestAttrib
|
|
);
|
|
|
|
$rulesContainer = Xml::element( 'div', $editorAttrib, $rules );
|
|
|
|
// Dummy textarea for submitting form and to use in case JS is disabled
|
|
$textareaAttribs = [
|
|
'style' => 'display: none'
|
|
];
|
|
if ( $externalForm ) {
|
|
$textareaAttribs['form'] = 'wpFilterForm';
|
|
}
|
|
$rulesContainer .= Xml::textarea( $textName, $rules, 40, 15, $textareaAttribs );
|
|
|
|
$editorConfig = AbuseFilter::getAceConfig( $canEdit );
|
|
|
|
// Add Ace configuration variable
|
|
$this->getOutput()->addJsConfigVars( 'aceConfig', $editorConfig );
|
|
} else {
|
|
if ( !$canEdit ) {
|
|
$editorAttrib['readonly'] = 'readonly';
|
|
}
|
|
if ( $externalForm ) {
|
|
$editorAttrib['form'] = 'wpFilterForm';
|
|
}
|
|
$rulesContainer = Xml::textarea( $textName, $rules, 40, 15, $editorAttrib );
|
|
}
|
|
|
|
if ( $canEdit ) {
|
|
// Generate builder drop-down
|
|
$dropDown = AbuseFilter::getBuilderValues();
|
|
|
|
// The array needs to be rearranged to be understood by OOUI
|
|
foreach ( $dropDown as $group => $values ) {
|
|
// Give grep a chance to find the usages:
|
|
// abusefilter-edit-builder-group-op-arithmetic, abusefilter-edit-builder-group-op-comparison,
|
|
// abusefilter-edit-builder-group-op-bool, abusefilter-edit-builder-group-misc,
|
|
// abusefilter-edit-builder-group-funcs, abusefilter-edit-builder-group-vars
|
|
$localisedLabel = $this->msg( "abusefilter-edit-builder-group-$group" )->text();
|
|
$dropDown[ $localisedLabel ] = $dropDown[ $group ];
|
|
unset( $dropDown[ $group ] );
|
|
$dropDown[ $localisedLabel ] = array_flip( $dropDown[ $localisedLabel ] );
|
|
foreach ( $values as $content => $name ) {
|
|
$localisedInnerLabel = $this->msg( "abusefilter-edit-builder-$group-$name" )->text();
|
|
$dropDown[ $localisedLabel ][ $localisedInnerLabel ] = $dropDown[ $localisedLabel ][ $name ];
|
|
unset( $dropDown[ $localisedLabel ][ $name ] );
|
|
}
|
|
}
|
|
|
|
$dropDown = [ $this->msg( 'abusefilter-edit-builder-select' )->text() => 'other' ] + $dropDown;
|
|
$dropDown = Xml::listDropDownOptionsOoui( $dropDown );
|
|
$dropDown = new OOUI\DropdownInputWidget( [
|
|
'name' => 'wpFilterBuilder',
|
|
'inputId' => 'wpFilterBuilder',
|
|
'options' => $dropDown
|
|
] );
|
|
|
|
$dropDown = new OOUI\FieldLayout( $dropDown );
|
|
$formElements = [ $dropDown ];
|
|
|
|
// Button for syntax check
|
|
$syntaxCheck =
|
|
new OOUI\ButtonWidget(
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-check' )->text(),
|
|
'id' => 'mw-abusefilter-syntaxcheck'
|
|
] + $noTestAttrib
|
|
);
|
|
$group = $syntaxCheck;
|
|
|
|
// Button for switching editor (if Ace is used)
|
|
if ( isset( $switchEditor ) ) {
|
|
$group =
|
|
new OOUI\Widget( [
|
|
'content' => new OOUI\HorizontalLayout( [
|
|
'items' => [ $switchEditor, $syntaxCheck ]
|
|
] )
|
|
] );
|
|
}
|
|
$group = new OOUI\FieldLayout( $group );
|
|
$formElements[] = $group;
|
|
|
|
$fieldSet = new OOUI\FieldsetLayout( [
|
|
'items' => $formElements,
|
|
'classes' => [ 'mw-abusefilter-edit-buttons' ]
|
|
] );
|
|
|
|
$rulesContainer .= $fieldSet;
|
|
}
|
|
|
|
if ( $addResultDiv ) {
|
|
$rulesContainer .= Xml::element( 'div',
|
|
[ 'id' => 'mw-abusefilter-syntaxresult', 'style' => 'display: none;' ],
|
|
' ' );
|
|
}
|
|
|
|
// Add script
|
|
$this->getOutput()->addModules( 'ext.abuseFilter.edit' );
|
|
AbuseFilter::$editboxName = $textName;
|
|
|
|
return $rulesContainer;
|
|
}
|
|
|
|
/**
|
|
* @param IDatabase $db
|
|
* @return string
|
|
*/
|
|
public function buildTestConditions( IDatabase $db ) {
|
|
// If one of these is true, we're abusefilter compatible.
|
|
return $db->makeList( [
|
|
'rc_source' => [
|
|
RecentChange::SRC_EDIT,
|
|
RecentChange::SRC_NEW,
|
|
],
|
|
$db->makeList( [
|
|
'rc_source' => RecentChange::SRC_LOG,
|
|
$db->makeList( [
|
|
$db->makeList( [
|
|
'rc_log_type' => 'move',
|
|
'rc_log_action' => 'move'
|
|
], LIST_AND ),
|
|
$db->makeList( [
|
|
'rc_log_type' => 'newusers',
|
|
'rc_log_action' => 'create'
|
|
], LIST_AND ),
|
|
$db->makeList( [
|
|
'rc_log_type' => 'delete',
|
|
'rc_log_action' => 'delete'
|
|
], LIST_AND ),
|
|
// @todo: add upload
|
|
], LIST_OR ),
|
|
], LIST_AND ),
|
|
], LIST_OR );
|
|
}
|
|
|
|
/**
|
|
* @static
|
|
* @return bool
|
|
*/
|
|
public static function canViewPrivate() {
|
|
global $wgUser;
|
|
static $canView = null;
|
|
|
|
if ( is_null( $canView ) ) {
|
|
$canView = $wgUser->isAllowedAny( 'abusefilter-modify', 'abusefilter-view-private' );
|
|
}
|
|
|
|
return $canView;
|
|
}
|
|
|
|
}
|