mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-13 17:57:06 +00:00
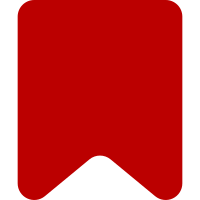
Rather than test for fetch, limit the code to ES6 browsers. Depends-On: I96a03796628a74ace93579d45a582711400c09c1 Change-Id: I4ca10182491118e61e155f99c713d4cb1b4fc7f0
53 lines
1.5 KiB
JavaScript
53 lines
1.5 KiB
JavaScript
/** @module search */
|
|
|
|
const
|
|
Vue = require( 'vue' ).default || require( 'vue' ),
|
|
App = require( './App.vue' ),
|
|
config = require( './config.json' );
|
|
|
|
/**
|
|
* @param {Element} searchBox
|
|
* @return {void}
|
|
*/
|
|
function initApp( searchBox ) {
|
|
const searchForm = searchBox.querySelector( '.vector-search-box-form' ),
|
|
titleInput = /** @type {HTMLInputElement|null} */ (
|
|
searchBox.querySelector( 'input[name=title]' )
|
|
),
|
|
search = /** @type {HTMLInputElement|null} */ ( searchBox.querySelector( 'input[name="search"]' ) ),
|
|
searchPageTitle = titleInput && titleInput.value;
|
|
|
|
if ( !searchForm || !search || !titleInput ) {
|
|
throw new Error( 'Attempted to create Vue search element from an incompatible element.' );
|
|
}
|
|
|
|
// @ts-ignore
|
|
Vue.createMwApp(
|
|
App, $.extend( {
|
|
id: searchForm.id,
|
|
autofocusInput: search === document.activeElement,
|
|
action: searchForm.getAttribute( 'action' ),
|
|
searchAccessKey: search.getAttribute( 'accessKey' ),
|
|
searchPageTitle: searchPageTitle,
|
|
searchTitle: search.getAttribute( 'title' ),
|
|
searchPlaceholder: search.getAttribute( 'placeholder' ),
|
|
searchQuery: search.value,
|
|
autoExpandWidth: searchBox ? searchBox.classList.contains( 'vector-search-box-auto-expand-width' ) : false
|
|
// Pass additional config from server.
|
|
}, config )
|
|
)
|
|
.mount( searchForm.parentNode );
|
|
}
|
|
/**
|
|
* @param {Document} document
|
|
* @return {void}
|
|
*/
|
|
function main( document ) {
|
|
const searchBoxes = document.querySelectorAll( '.vector-search-box' );
|
|
|
|
searchBoxes.forEach( ( searchBox ) => {
|
|
initApp( searchBox );
|
|
} );
|
|
}
|
|
main( document );
|