mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-25 16:15:28 +00:00
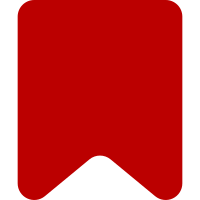
When the vector-sticky-header-enabled class is removed from the body it means the feature is not enabled, it doesn't mean the sticky header should be invisible. Call the hide method instead, and move it out of the function given its a side effect Bug: T308343 Change-Id: I4ecd6524146f203af926847812e20275c9573cab
128 lines
3.4 KiB
JavaScript
128 lines
3.4 KiB
JavaScript
const { test } = require( '../../../resources/skins.vector.es6/main.js' );
|
|
const {
|
|
STICKY_HEADER_EXPERIMENT_NAME,
|
|
STICKY_HEADER_EDIT_EXPERIMENT_NAME
|
|
} = require( '../../../resources/skins.vector.es6/stickyHeader.js' );
|
|
describe( 'main.js', () => {
|
|
it( 'getHeadingIntersectionHandler', () => {
|
|
const section = document.createElement( 'div' );
|
|
section.setAttribute( 'class', 'mw-body-content' );
|
|
section.setAttribute( 'id', 'mw-content-text' );
|
|
const heading = document.createElement( 'h2' );
|
|
const headline = document.createElement( 'span' );
|
|
headline.classList.add( 'mw-headline' );
|
|
headline.setAttribute( 'id', 'headline' );
|
|
heading.appendChild( headline );
|
|
section.appendChild(
|
|
heading
|
|
);
|
|
|
|
[
|
|
[ section, 'toc-mw-content-text' ],
|
|
[ heading, 'toc-headline' ]
|
|
].forEach( ( testCase ) => {
|
|
const node = /** @type {HTMLElement} */ ( testCase[ 0 ] );
|
|
const fn = jest.fn();
|
|
const handler = test.getHeadingIntersectionHandler( fn );
|
|
handler( node );
|
|
expect( fn ).toHaveBeenCalledWith( testCase[ 1 ] );
|
|
} );
|
|
} );
|
|
|
|
it( 'initStickyHeaderABTests', () => {
|
|
const STICKY_HEADER_AB = {
|
|
name: STICKY_HEADER_EXPERIMENT_NAME,
|
|
enabled: true
|
|
};
|
|
const STICKY_HEADER_AB_EDIT = {
|
|
name: STICKY_HEADER_EDIT_EXPERIMENT_NAME,
|
|
enabled: true
|
|
};
|
|
[
|
|
{
|
|
abConfig: STICKY_HEADER_AB_EDIT,
|
|
isEnabled: false,
|
|
isUserInTreatmentBucket: false,
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB_EDIT,
|
|
isEnabled: true,
|
|
isUserInTreatmentBucket: false,
|
|
expectedResult: {
|
|
showStickyHeader: true,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB_EDIT,
|
|
isEnabled: true,
|
|
isUserInTreatmentBucket: true,
|
|
expectedResult: {
|
|
showStickyHeader: true,
|
|
disableEditIcons: false
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB,
|
|
isEnabled: false, // sticky header unavailable
|
|
isUserInTreatmentBucket: false, // not in treament bucket
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB,
|
|
isEnabled: true, // sticky header available
|
|
isUserInTreatmentBucket: false, // not in treament bucket
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB,
|
|
isEnabled: false, // sticky header is not available
|
|
isUserInTreatmentBucket: true, // but the user is in the treament bucket
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB,
|
|
isEnabled: true,
|
|
isUserInTreatmentBucket: true,
|
|
expectedResult: {
|
|
showStickyHeader: true,
|
|
disableEditIcons: true
|
|
}
|
|
}
|
|
].forEach( ( { abConfig, isEnabled, isUserInTreatmentBucket, expectedResult } ) => {
|
|
document.documentElement.classList.add( 'vector-sticky-header-enabled' );
|
|
const result = test.initStickyHeaderABTests(
|
|
abConfig,
|
|
isEnabled,
|
|
( experiment ) => ( {
|
|
name: experiment.name,
|
|
isInBucket: () => true,
|
|
isInSample: () => true,
|
|
getBucket: () => 'bucket',
|
|
isInTreatmentBucket: () => {
|
|
return isUserInTreatmentBucket;
|
|
}
|
|
} )
|
|
);
|
|
expect( result ).toMatchObject( expectedResult );
|
|
// Check that there are no side effects
|
|
expect(
|
|
document.documentElement.classList.contains( 'vector-sticky-header-enabled' )
|
|
).toBe( true );
|
|
} );
|
|
} );
|
|
} );
|