mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-26 08:35:42 +00:00
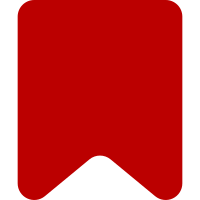
* Leverage the infrastructure around feature management to handle the page tools pinning and persistence * Make pinnableHeader.js leverage features.js if the data-feature-name attribute is set * Sets tests/.eslintrc.json ecmaVersion to 2018 to enable destructuring in test files. * Adds a isPinned helper method to pinnableElement * Add a logged in requirement so that the pinned feature is disabled for anon users. Bug: T322051 Change-Id: Ib86282216882fa94e37b7088a3f4bd0c1bcf6cd4
74 lines
1.5 KiB
PHP
74 lines
1.5 KiB
PHP
<?php
|
|
namespace MediaWiki\Skins\Vector\Components;
|
|
|
|
use Skin;
|
|
|
|
/**
|
|
* VectorComponentMainMenu component
|
|
*/
|
|
class VectorComponentPageTools implements VectorComponent {
|
|
|
|
/** @var array */
|
|
private $toolbox;
|
|
|
|
/** @var array */
|
|
private $actionsMenu;
|
|
|
|
/** @var bool */
|
|
private $isPinned;
|
|
|
|
/** @var Skin */
|
|
private $skin;
|
|
|
|
/** @var VectorComponentPinnableHeader|null */
|
|
private $pinnableHeader;
|
|
|
|
/** @var string */
|
|
public const TOOLBOX_ID = 'p-tb';
|
|
|
|
/**
|
|
* @param array $toolbox
|
|
* @param array $actionsMenu
|
|
* @param bool $isPinned
|
|
* @param Skin $skin
|
|
*/
|
|
public function __construct(
|
|
array $toolbox,
|
|
array $actionsMenu,
|
|
bool $isPinned,
|
|
Skin $skin
|
|
) {
|
|
$user = $skin->getUser();
|
|
$this->toolbox = $toolbox;
|
|
$this->actionsMenu = $actionsMenu;
|
|
$this->isPinned = $isPinned;
|
|
$this->pinnableHeader = $user->isRegistered() ? new VectorComponentPinnableHeader(
|
|
$skin->getContext(),
|
|
$isPinned,
|
|
// Name
|
|
'vector-page-tools',
|
|
// Feature name
|
|
'page-tools-pinned'
|
|
) : null;
|
|
$this->skin = $skin;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getTemplateData(): array {
|
|
$menusData = [ $this->toolbox, $this->actionsMenu ];
|
|
|
|
$pinnableDropdownData = [
|
|
'id' => 'vector-page-tools',
|
|
'class' => 'vector-page-tools',
|
|
'label' => $this->skin->msg( 'toolbox' ),
|
|
'is-pinned' => $this->isPinned,
|
|
'has-multiple-menus' => true,
|
|
'data-pinnable-header' => $this->pinnableHeader ? $this->pinnableHeader->getTemplateData() : null,
|
|
'data-menus' => $menusData
|
|
];
|
|
return $pinnableDropdownData;
|
|
}
|
|
}
|