mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-25 08:05:51 +00:00
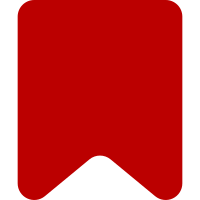
Previously for the table of contents A/B test we triggered scroll events when the table of contents was scrolled into view. Now this is never referenced, so the code is cleaned up to only apply to when the title is scrolled into view. Change-Id: Ie9a5c4b6d88068d914cbbcc3d046ac288d49172b
49 lines
1.4 KiB
JavaScript
49 lines
1.4 KiB
JavaScript
const
|
|
SCROLL_TITLE_HOOK = 'vector.page_title_scroll',
|
|
SCROLL_TITLE_CONTEXT_ABOVE = 'scrolled-above-page-title',
|
|
SCROLL_TITLE_CONTEXT_BELOW = 'scrolled-below-page-title',
|
|
SCROLL_TITLE_ACTION = 'scroll-to-top';
|
|
|
|
/**
|
|
* Fire a hook to be captured by WikimediaEvents for scroll event logging.
|
|
*
|
|
* @param {string} direction the scroll direction
|
|
*/
|
|
function firePageTitleScrollHook( direction ) {
|
|
if ( direction === 'down' ) {
|
|
mw.hook( SCROLL_TITLE_HOOK ).fire( {
|
|
context: SCROLL_TITLE_CONTEXT_BELOW
|
|
} );
|
|
} else {
|
|
mw.hook( SCROLL_TITLE_HOOK ).fire( {
|
|
context: SCROLL_TITLE_CONTEXT_ABOVE,
|
|
action: SCROLL_TITLE_ACTION
|
|
} );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Create an observer for showing/hiding feature and for firing scroll event hooks.
|
|
*
|
|
* @param {Function} show functionality for when feature is visible
|
|
* @param {Function} hide functionality for when feature is hidden
|
|
* @return {IntersectionObserver}
|
|
*/
|
|
function initScrollObserver( show, hide ) {
|
|
/* eslint-disable-next-line compat/compat */
|
|
return new IntersectionObserver( function ( entries ) {
|
|
if ( !entries[ 0 ].isIntersecting && entries[ 0 ].boundingClientRect.top < 0 ) {
|
|
// Viewport has crossed the bottom edge of the target element.
|
|
show();
|
|
} else {
|
|
// Viewport is above the bottom edge of the target element.
|
|
hide();
|
|
}
|
|
} );
|
|
}
|
|
|
|
module.exports = {
|
|
initScrollObserver,
|
|
firePageTitleScrollHook
|
|
};
|