mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-12 09:21:11 +00:00
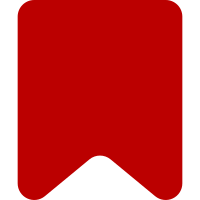
If the wgVectorSearchClient supports it (the default implementation doesn’t), add a visible-item-limit to the cdx-typeahead-search, and wire up the resulting load-more event (new in Codex v0.3) to load additional search results on scroll. The hard-coded visibleItemLimit (7) is chosen to match the default limit of the wbsearchentities API, but ultimately arbitrary; we can look into how to make this number configurable later, if necessary. This increases the module size enough that we need to bump the bundle size a bit more. Bug: T322333 Change-Id: Iadade9cbf48457cfeabc78439624602ec3f98782 Co-Authored-By: Jon Robson <jdlrobson@gmail.com> Needed-By: I67fac3b209d6a1ab2661e1e1c0681edd8472ac6c
122 lines
3 KiB
JavaScript
122 lines
3 KiB
JavaScript
/* global RestResult, SearchResult */
|
|
/** @module restSearchClient */
|
|
|
|
const fetchJson = require( './fetch.js' );
|
|
const urlGenerator = require( './urlGenerator.js' );
|
|
|
|
/**
|
|
* @typedef {Object} RestResponse
|
|
* @property {RestResult[]} pages
|
|
*/
|
|
|
|
/**
|
|
* @typedef {Object} SearchResponse
|
|
* @property {string} query
|
|
* @property {SearchResult[]} results
|
|
*/
|
|
|
|
/**
|
|
* Nullish coalescing operator (??) helper
|
|
*
|
|
* @param {any} a
|
|
* @param {any} b
|
|
* @return {any}
|
|
*/
|
|
function nullish( a, b ) {
|
|
return ( a !== null && a !== undefined ) ? a : b;
|
|
}
|
|
|
|
/**
|
|
* @param {MwMap} config
|
|
* @param {string} query
|
|
* @param {RestResponse} restResponse
|
|
* @param {boolean} showDescription
|
|
* @return {SearchResponse}
|
|
*/
|
|
function adaptApiResponse( config, query, restResponse, showDescription ) {
|
|
const urlGeneratorInstance = urlGenerator( config );
|
|
return {
|
|
query,
|
|
results: restResponse.pages.map( ( page, index ) => {
|
|
const thumbnail = page.thumbnail;
|
|
return {
|
|
id: page.id,
|
|
value: page.id || -( index + 1 ),
|
|
label: page.title,
|
|
key: page.key,
|
|
title: page.title,
|
|
description: showDescription ? page.description : undefined,
|
|
url: urlGeneratorInstance.generateUrl( page ),
|
|
thumbnail: thumbnail ? {
|
|
url: thumbnail.url,
|
|
width: nullish( thumbnail.width, undefined ),
|
|
height: nullish( thumbnail.height, undefined )
|
|
} : undefined
|
|
};
|
|
} )
|
|
};
|
|
}
|
|
|
|
/**
|
|
* @typedef {Object} AbortableSearchFetch
|
|
* @property {Promise<SearchResponse>} fetch
|
|
* @property {Function} abort
|
|
*/
|
|
|
|
/**
|
|
* @callback fetchByTitle
|
|
* @param {string} query The search term.
|
|
* @param {number} [limit] Maximum number of results.
|
|
* @param {boolean} [showDescription] Whether descriptions should be added to the results.
|
|
* @return {AbortableSearchFetch}
|
|
*/
|
|
|
|
/**
|
|
* @callback loadMore
|
|
* @param {string} query The search term.
|
|
* @param {number} offset The number of search results that were already loaded.
|
|
* @param {number} [limit] How many further search results to load (at most).
|
|
* @param {boolean} [showDescription] Whether descriptions should be added to the results.
|
|
* @return {AbortableSearchFetch}
|
|
*/
|
|
|
|
/**
|
|
* @typedef {Object} SearchClient
|
|
* @property {fetchByTitle} fetchByTitle
|
|
* @property {loadMore} [loadMore]
|
|
*/
|
|
|
|
/**
|
|
* @param {MwMap} config
|
|
* @return {SearchClient}
|
|
*/
|
|
function restSearchClient( config ) {
|
|
return config.get( 'wgVectorSearchClient', {
|
|
/**
|
|
* @type {fetchByTitle}
|
|
*/
|
|
fetchByTitle: ( q, limit = 10, showDescription = true ) => {
|
|
const searchApiUrl = config.get( 'wgVectorSearchApiUrl',
|
|
config.get( 'wgScriptPath' ) + '/rest.php'
|
|
);
|
|
const params = { q, limit };
|
|
const url = searchApiUrl + '/v1/search/title?' + $.param( params );
|
|
const result = fetchJson( url, {
|
|
headers: {
|
|
accept: 'application/json'
|
|
}
|
|
} );
|
|
const searchResponsePromise = result.fetch
|
|
.then( ( /** @type {RestResponse} */ res ) => {
|
|
return adaptApiResponse( config, q, res, showDescription );
|
|
} );
|
|
return {
|
|
abort: result.abort,
|
|
fetch: searchResponsePromise
|
|
};
|
|
}
|
|
} );
|
|
}
|
|
|
|
module.exports = restSearchClient;
|