mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-25 08:05:51 +00:00
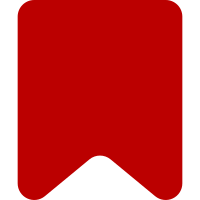
Uses all of the changes from Jon's patch in 866503 to move all of the sidebar menus after and including the tools menu into the page tools component. The rest of the menus remain in the main menu. Additionally: * Per T317898#8468437, the "Tools" label should be "General". It was also assumed from the prototype that the "More" label should become "Actions" Bug: T317898 Change-Id: Ic9c1586febd8ebfff4a17285e6bd59cee509bd34
94 lines
2 KiB
PHP
94 lines
2 KiB
PHP
<?php
|
|
namespace MediaWiki\Skins\Vector\Components;
|
|
|
|
use MessageLocalizer;
|
|
use User;
|
|
|
|
/**
|
|
* VectorComponentMainMenu component
|
|
*/
|
|
class VectorComponentPageTools implements VectorComponent {
|
|
|
|
/** @var array */
|
|
private $menus;
|
|
|
|
/** @var bool */
|
|
private $isPinned;
|
|
|
|
/** @var VectorComponentPinnableHeader|null */
|
|
private $pinnableHeader;
|
|
|
|
/** @var string */
|
|
public const ID = 'vector-page-tools';
|
|
|
|
/** @var string */
|
|
public const TOOLBOX_ID = 'p-tb';
|
|
|
|
/** @var string */
|
|
private const ACTIONS_ID = 'p-cactions';
|
|
|
|
/** @var MessageLocalizer */
|
|
private $localizer;
|
|
|
|
/**
|
|
* @param array $menus
|
|
* @param bool $isPinned
|
|
* @param MessageLocalizer $localizer
|
|
* @param User $user
|
|
*/
|
|
public function __construct(
|
|
array $menus,
|
|
bool $isPinned,
|
|
MessageLocalizer $localizer,
|
|
User $user
|
|
) {
|
|
$this->menus = $menus;
|
|
$this->isPinned = $isPinned;
|
|
$this->localizer = $localizer;
|
|
$this->pinnableHeader = $user->isRegistered() ? new VectorComponentPinnableHeader(
|
|
$localizer,
|
|
$isPinned,
|
|
// Name
|
|
'vector-page-tools',
|
|
// Feature name
|
|
'page-tools-pinned'
|
|
) : null;
|
|
}
|
|
|
|
/**
|
|
* Revises the labels of the p-tb and p-cactions menus.
|
|
*
|
|
* @return array
|
|
*/
|
|
private function getMenus(): array {
|
|
return array_map( function ( $menu ) {
|
|
switch ( $menu['id'] ?? '' ) {
|
|
case self::TOOLBOX_ID:
|
|
$menu['label'] = $this->localizer->msg( 'vector-page-tools-general-label' );
|
|
break;
|
|
case self::ACTIONS_ID:
|
|
$menu['label'] = $this->localizer->msg( 'vector-page-tools-actions-label' );
|
|
break;
|
|
}
|
|
|
|
return $menu;
|
|
}, $this->menus );
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getTemplateData(): array {
|
|
$pinnedContainer = new VectorComponentPinnedContainer( self::ID, $this->isPinned );
|
|
$pinnableElement = new VectorComponentPinnableElement( self::ID );
|
|
|
|
$data = $pinnableElement->getTemplateData() +
|
|
$pinnedContainer->getTemplateData();
|
|
|
|
return $data + [
|
|
'data-pinnable-header' => $this->pinnableHeader ? $this->pinnableHeader->getTemplateData() : null,
|
|
'data-menus' => $this->getMenus()
|
|
];
|
|
}
|
|
}
|