mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-25 16:15:28 +00:00
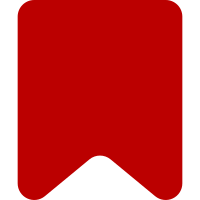
For idwiki/viwiki, we wish to run the sticky header edit button AB test so that treatment1 group sees the sticky header without edit buttons, treatment2 groups sees the sticky header with edit buttons, and the control/unsampled groups see no sticky header at all. This patch overrides the configuration to make the sticky header w/o edit buttons for treatment1, sticky header w/ edit buttons for treatment2, and hides sticky header for everyone else. This depends on a configuration with the treatment groups having "treatment1" and "treatment2" as substrings in their bucket names. The full configuration for idwiki/viwiki would be something like the following: ``` $wgVectorStickyHeader = [ "logged_in" => true, "logged_out" => false, ]; $wgVectorStickyHeaderEdit = [ "logged_in" => true, "logged_out" => false, ]; $wgVectorWebABTestEnrollment = [ "name" => "vector.sticky_header_edit", "enabled" => true, "buckets" => [ "unsampled" => [ "samplingRate" => 0 ], "noStickyHeaderControl" => [ "samplingRate" => 0.34 ], "stickyHeaderNoEditButtonTreatment1" => [ "samplingRate" => 0.33 ], "stickyHeaderEditButtonTreatment2" => [ "samplingRate" => 0.33 ] ], ]; ``` Bug: T312573 Change-Id: I15c360fdf5393f5594602acc33b5b916e904016d
162 lines
4.1 KiB
JavaScript
162 lines
4.1 KiB
JavaScript
const { test } = require( '../../../resources/skins.vector.es6/main.js' );
|
|
const {
|
|
STICKY_HEADER_EXPERIMENT_NAME,
|
|
STICKY_HEADER_EDIT_EXPERIMENT_NAME
|
|
} = require( '../../../resources/skins.vector.es6/stickyHeader.js' );
|
|
describe( 'main.js', () => {
|
|
it( 'getHeadingIntersectionHandler', () => {
|
|
const section = document.createElement( 'div' );
|
|
section.setAttribute( 'class', 'mw-body-content' );
|
|
section.setAttribute( 'id', 'mw-content-text' );
|
|
const heading = document.createElement( 'h2' );
|
|
const headline = document.createElement( 'span' );
|
|
headline.classList.add( 'mw-headline' );
|
|
headline.setAttribute( 'id', 'headline' );
|
|
heading.appendChild( headline );
|
|
section.appendChild(
|
|
heading
|
|
);
|
|
|
|
[
|
|
[ section, 'toc-mw-content-text' ],
|
|
[ heading, 'toc-headline' ]
|
|
].forEach( ( testCase ) => {
|
|
const node = /** @type {HTMLElement} */ ( testCase[ 0 ] );
|
|
const fn = jest.fn();
|
|
const handler = test.getHeadingIntersectionHandler( fn );
|
|
handler( node );
|
|
expect( fn ).toHaveBeenCalledWith( testCase[ 1 ] );
|
|
} );
|
|
} );
|
|
|
|
it( 'initStickyHeaderABTests', () => {
|
|
const STICKY_HEADER_AB = {
|
|
name: STICKY_HEADER_EXPERIMENT_NAME,
|
|
enabled: true
|
|
};
|
|
const STICKY_HEADER_AB_EDIT = {
|
|
name: STICKY_HEADER_EDIT_EXPERIMENT_NAME,
|
|
enabled: true
|
|
};
|
|
const DISABLED_STICKY_HEADER_AB_EDIT = {
|
|
name: STICKY_HEADER_EDIT_EXPERIMENT_NAME,
|
|
enabled: false
|
|
};
|
|
const TABLE_OF_CONTENTS_AB = {
|
|
name: 'skin-vector-toc-experiment',
|
|
enabled: true,
|
|
buckets: {}
|
|
};
|
|
[
|
|
{
|
|
abConfig: STICKY_HEADER_AB_EDIT,
|
|
isEnabled: false,
|
|
isUserInTreatmentBucket: false,
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB_EDIT,
|
|
isEnabled: true,
|
|
isUserInTreatmentBucket: false,
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB_EDIT,
|
|
isEnabled: true,
|
|
isUserInTreatmentBucket: true,
|
|
expectedResult: {
|
|
showStickyHeader: true,
|
|
disableEditIcons: false
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB,
|
|
isEnabled: false, // sticky header unavailable
|
|
isUserInTreatmentBucket: false, // not in treatment bucket
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB,
|
|
isEnabled: true, // sticky header available
|
|
isUserInTreatmentBucket: false, // not in treatment bucket
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB,
|
|
isEnabled: false, // sticky header is not available
|
|
isUserInTreatmentBucket: true, // but the user is in the treatment bucket
|
|
expectedResult: {
|
|
showStickyHeader: false,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: STICKY_HEADER_AB,
|
|
isEnabled: true,
|
|
isUserInTreatmentBucket: true,
|
|
expectedResult: {
|
|
showStickyHeader: true,
|
|
disableEditIcons: true
|
|
}
|
|
},
|
|
{
|
|
abConfig: TABLE_OF_CONTENTS_AB,
|
|
isEnabled: true,
|
|
isUserInTreatmentBucket: false,
|
|
expectedResult: {
|
|
showStickyHeader: true,
|
|
disableEditIcons: false
|
|
}
|
|
},
|
|
{
|
|
abConfig: DISABLED_STICKY_HEADER_AB_EDIT,
|
|
isEnabled: true,
|
|
isUserInTreatmentBucket: false,
|
|
expectedResult: {
|
|
showStickyHeader: true,
|
|
disableEditIcons: false
|
|
}
|
|
}
|
|
].forEach(
|
|
( {
|
|
abConfig,
|
|
isEnabled,
|
|
isUserInTreatmentBucket,
|
|
expectedResult
|
|
} ) => {
|
|
document.documentElement.classList.add( 'vector-sticky-header-enabled' );
|
|
const result = test.initStickyHeaderABTests(
|
|
abConfig,
|
|
// isStickyHeaderFeatureAllowed
|
|
isEnabled,
|
|
( experiment ) => ( {
|
|
name: experiment.name,
|
|
isInBucket: () => true,
|
|
isInSample: () => true,
|
|
getBucket: () => 'bucket',
|
|
isInTreatmentBucket: () => {
|
|
return isUserInTreatmentBucket;
|
|
}
|
|
} )
|
|
);
|
|
expect( result ).toMatchObject( expectedResult );
|
|
// Check that there are no side effects
|
|
expect(
|
|
document.documentElement.classList.contains( 'vector-sticky-header-enabled' )
|
|
).toBe( true );
|
|
} );
|
|
} );
|
|
} );
|