mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-14 19:26:42 +00:00
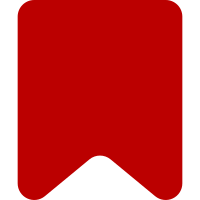
Simplify the user links component Introduce MenuListItem and IconLink components. HTML changes: * Logout, login and account links are now wrapped in a ul and li tag instead of a DIV for consistent with other menus (this occurs due to use of MenuContents). Code reviewer can see tests for an understanding of how the template data and markup has changed. VISUAL CHANGE: * Increased margins in user links menu relating to change from DIV to UL / LI tags. Bug: T320927 Change-Id: Ia24be48105e1ff85da227883abb5dddb3d54388d
67 lines
1.6 KiB
PHP
67 lines
1.6 KiB
PHP
<?php
|
|
namespace MediaWiki\Skins\Vector\Components;
|
|
|
|
use Html;
|
|
use Linker;
|
|
use MessageLocalizer;
|
|
|
|
/**
|
|
* VectorComponentIconLink component
|
|
*/
|
|
class VectorComponentIconLink implements VectorComponent {
|
|
/** @var MessageLocalizer */
|
|
private $localizer;
|
|
/** @var string */
|
|
private $icon;
|
|
/** @var string */
|
|
private $href;
|
|
/** @var string */
|
|
private $text;
|
|
/** @var string */
|
|
private $accessKeyHint;
|
|
|
|
/**
|
|
* @param string $href
|
|
* @param string $text
|
|
* @param null|string $icon
|
|
* @param null|MessageLocalizer $localizer for generation of tooltip and access keys
|
|
* @param null|string $accessKeyHint will be used to derive HTML attributes such as title, accesskey
|
|
* and aria-label ("$accessKeyHint-label")
|
|
*/
|
|
public function __construct( string $href, string $text, $icon = null, $localizer = null, $accessKeyHint = null ) {
|
|
$this->href = $href;
|
|
$this->text = $text;
|
|
$this->icon = $icon;
|
|
$this->localizer = $localizer;
|
|
$this->accessKeyHint = $accessKeyHint;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getTemplateData(): array {
|
|
$localizer = $this->localizer;
|
|
$accessKeyHint = $this->accessKeyHint;
|
|
$additionalAttributes = [];
|
|
if ( $localizer ) {
|
|
$msg = $localizer->msg( $accessKeyHint . '-label' );
|
|
if ( $msg->exists() ) {
|
|
$additionalAttributes[ 'aria-label' ] = $msg->text();
|
|
}
|
|
}
|
|
return [
|
|
'icon' => $this->icon,
|
|
'text' => $this->text,
|
|
'href' => $this->href,
|
|
'html-attributes' => $localizer && $accessKeyHint ? Html::expandAttributes(
|
|
Linker::tooltipAndAccesskeyAttribs(
|
|
$accessKeyHint,
|
|
[],
|
|
[],
|
|
$localizer
|
|
) + $additionalAttributes
|
|
) : '',
|
|
];
|
|
}
|
|
}
|