mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-13 17:57:06 +00:00
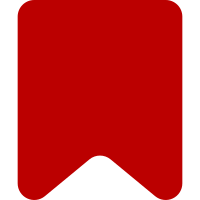
To aid moving towards a MainMenu component we must first make subcomponents for the language alert and opt-out link (which share code which I've captured in VectorComponentMainMenuAction) Code is lifted into SkinVector22 to allow us to easily make the template change without any breaking visual changes Bug: T319349 Bug: T322089 Change-Id: Ibb69d029a9fb6cee3482e15a60a7358361bd2405
56 lines
1.5 KiB
PHP
56 lines
1.5 KiB
PHP
<?php
|
|
namespace MediaWiki\Skins\Vector\Components;
|
|
|
|
use Skin;
|
|
|
|
/**
|
|
* VectorComponentMainMenuAction component
|
|
*/
|
|
class VectorComponentMainMenuAction implements VectorComponent {
|
|
/** @var Skin */
|
|
private $skin;
|
|
/** @var array */
|
|
private $htmlData;
|
|
/** @var array */
|
|
private $headingOptions;
|
|
|
|
/**
|
|
* @param Skin $skin
|
|
* @param array $htmlData data to make a link or raw html
|
|
* @param array $headingOptions optional heading for the html
|
|
*/
|
|
public function __construct( Skin $skin, array $htmlData, array $headingOptions ) {
|
|
$this->skin = $skin;
|
|
$this->htmlData = $htmlData;
|
|
$this->headingOptions = $headingOptions;
|
|
}
|
|
|
|
/**
|
|
* Generate data needed to create MainMenuAction item.
|
|
* @param array $htmlData data to make a link or raw html
|
|
* @param array $headingOptions optional heading for the html
|
|
* @return array keyed data for the MainMenuAction template
|
|
*/
|
|
private function makeMainMenuActionData( array $htmlData = [], array $headingOptions = [] ): array {
|
|
$skin = $this->skin;
|
|
$htmlContent = '';
|
|
// Populates the main menu as a standalone link or custom html.
|
|
if ( array_key_exists( 'link', $htmlData ) ) {
|
|
$htmlContent = $skin->makeLink( 'link', $htmlData['link'] );
|
|
} elseif ( array_key_exists( 'html-content', $htmlData ) ) {
|
|
$htmlContent = $htmlData['html-content'];
|
|
}
|
|
|
|
return $headingOptions + [
|
|
'html-content' => $htmlContent,
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getTemplateData(): array {
|
|
return $this->makeMainMenuActionData( $this->htmlData, $this->headingOptions );
|
|
}
|
|
}
|