mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-09-24 10:49:25 +00:00
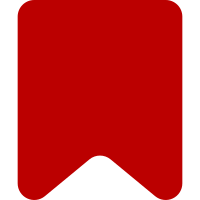
As described in the readme but not implemented until now, this patch enables the skin version to be specified as a URL query parameter. This is useful for testing both skin versions during development and on wiki, as well as enabling sharing URLs with a specific skin (Vector) and skin version (1 or 2). Obtaining the actual skin version requires tying together three input sources, WebRequest, User, and Config. It seems simple but it'd be easy to botch. For this reason, a helper class to correctly interrogate them and tests are provided. Bug: T244481 Change-Id: I52d80942b4270c008d4e45050589ed9220255a50
116 lines
3.2 KiB
PHP
116 lines
3.2 KiB
PHP
<?php
|
|
/**
|
|
* Vector - Modern version of MonoBook with fresh look and many usability
|
|
* improvements.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*
|
|
* @file
|
|
* @ingroup Skins
|
|
*/
|
|
use Vector\SkinVersionLookup;
|
|
|
|
/**
|
|
* Skin subclass for Vector
|
|
* @ingroup Skins
|
|
* @final skins extending SkinVector are not supported
|
|
* @unstable
|
|
*/
|
|
class SkinVector extends SkinTemplate {
|
|
public $skinname = 'vector';
|
|
public $stylename = 'Vector';
|
|
public $template = 'VectorTemplate';
|
|
|
|
private $responsiveMode = false;
|
|
|
|
/**
|
|
* @var SkinVersionLookup
|
|
*/
|
|
private $skinVersionLookup;
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function __construct( $skinname = null ) {
|
|
parent::__construct( $skinname );
|
|
|
|
$this->skinVersionLookup =
|
|
new SkinVersionLookup( $this->getRequest(), $this->getUser(), $this->getConfig() );
|
|
}
|
|
|
|
/**
|
|
* Enables the responsive mode
|
|
*/
|
|
public function enableResponsiveMode() {
|
|
if ( !$this->responsiveMode ) {
|
|
$out = $this->getOutput();
|
|
$out->addMeta( 'viewport', 'width=device-width, initial-scale=1' );
|
|
$out->addModuleStyles( 'skins.vector.styles.responsive' );
|
|
$this->responsiveMode = true;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Initializes output page and sets up skin-specific parameters
|
|
* @param OutputPage $out Object to initialize
|
|
*/
|
|
public function initPage( OutputPage $out ) {
|
|
parent::initPage( $out );
|
|
|
|
if ( $this->getConfig()->get( 'VectorResponsive' ) ) {
|
|
$this->enableResponsiveMode();
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @return array
|
|
*/
|
|
public function getDefaultModules() {
|
|
$modules = parent::getDefaultModules();
|
|
// add vector skin styles and vector module
|
|
$module = $this->skinVersionLookup->isLegacy()
|
|
? 'skins.vector.styles.legacy' : 'skins.vector.styles';
|
|
$modules['styles']['skin'][] = $module;
|
|
$modules['core'][] = 'skins.vector.js';
|
|
|
|
return $modules;
|
|
}
|
|
|
|
/**
|
|
* Set up the VectorTemplate. Overrides the default behaviour of SkinTemplate allowing
|
|
* the safe calling of constructor with additional arguments. If dropping this method
|
|
* please ensure that VectorTemplate constructor arguments match those in SkinTemplate.
|
|
*
|
|
* @internal
|
|
* @param string $classname
|
|
* @return VectorTemplate
|
|
*/
|
|
protected function setupTemplate( $classname ) {
|
|
$tp = new TemplateParser( __DIR__ . '/templates' );
|
|
return new VectorTemplate( $this->getConfig(), $tp, $this->skinVersionLookup->isLegacy() );
|
|
}
|
|
|
|
/**
|
|
* Whether the logo should be preloaded with an HTTP link header or not
|
|
* @since 1.29
|
|
* @return bool
|
|
*/
|
|
public function shouldPreloadLogo() {
|
|
return true;
|
|
}
|
|
}
|