mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-12-24 13:43:16 +00:00
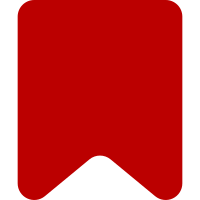
* Using the new ConfigHelper, support disabling night mode on certain pages via configuration options * In addition, adds test coverage for this case and the query parameter case (in a new integration test file as we now require accessing the service container for request context) * Finally, supply a default configuration in skin.json with all the values set to null For the easiest possible approach while we evaulate our general feature management system, this logic is handled inside of getFeatureBodyClass using the new shouldDisable general function. In the future, it may make sense to break this out into its own requirement class, but for now that feels premature Bug: T359606 Change-Id: I3e7a4720ec3cc2afd9777e36f59aa56b682258f0
148 lines
4.4 KiB
PHP
148 lines
4.4 KiB
PHP
<?php
|
|
/**
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*
|
|
* @file
|
|
* @since 1.35
|
|
*/
|
|
|
|
namespace MediaWiki\Skins\Vector\Tests\Unit\FeatureManagement;
|
|
|
|
use MediaWiki\Skins\Vector\FeatureManagement\FeatureManager;
|
|
|
|
/**
|
|
* @group Vector
|
|
* @group FeatureManagement
|
|
* @coversDefaultClass \MediaWiki\Skins\Vector\FeatureManagement\FeatureManager
|
|
*/
|
|
class FeatureManagerTest extends \MediaWikiUnitTestCase {
|
|
|
|
/**
|
|
* @covers ::registerSimpleRequirement
|
|
* @covers ::registerRequirement
|
|
*/
|
|
public function testRegisterSimpleRequirementThrowsWhenRequirementIsRegisteredTwice() {
|
|
$this->expectException( \LogicException::class );
|
|
|
|
$featureManager = new FeatureManager();
|
|
$featureManager->registerSimpleRequirement( 'requirementA', true );
|
|
$featureManager->registerSimpleRequirement( 'requirementA', true );
|
|
}
|
|
|
|
public static function provideInvalidFeatureConfig() {
|
|
return [
|
|
|
|
// ::registerFeature( string, int[] ) will throw an exception.
|
|
[
|
|
\Wikimedia\Assert\ParameterAssertionException::class,
|
|
[ 1 ],
|
|
],
|
|
|
|
// The "bar" requirement hasn't been registered.
|
|
[
|
|
\InvalidArgumentException::class,
|
|
[
|
|
'bar',
|
|
],
|
|
],
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @dataProvider provideInvalidFeatureConfig
|
|
* @covers ::registerFeature
|
|
*/
|
|
public function testRegisterFeatureValidatesConfig( $expectedExceptionType, $config ) {
|
|
$this->expectException( $expectedExceptionType );
|
|
|
|
$featureManager = new FeatureManager();
|
|
$featureManager->registerSimpleRequirement( 'requirement', true );
|
|
$featureManager->registerFeature( 'feature', $config );
|
|
}
|
|
|
|
/**
|
|
* @covers ::isRequirementMet
|
|
*/
|
|
public function testIsRequirementMet() {
|
|
$featureManager = new FeatureManager();
|
|
$featureManager->registerSimpleRequirement( 'enabled', true );
|
|
$featureManager->registerSimpleRequirement( 'disabled', false );
|
|
|
|
$this->assertTrue( $featureManager->isRequirementMet( 'enabled' ) );
|
|
$this->assertFalse( $featureManager->isRequirementMet( 'disabled' ) );
|
|
}
|
|
|
|
/**
|
|
* @covers ::isRequirementMet
|
|
*/
|
|
public function testIsRequirementMetThrowsExceptionWhenRequirementIsntRegistered() {
|
|
$this->expectException( \InvalidArgumentException::class );
|
|
|
|
$featureManager = new FeatureManager();
|
|
$featureManager->isRequirementMet( 'foo' );
|
|
}
|
|
|
|
/**
|
|
* @covers ::registerFeature
|
|
*/
|
|
public function testRegisterFeatureThrowsExceptionWhenFeatureIsRegisteredTwice() {
|
|
$this->expectException( \LogicException::class );
|
|
|
|
$featureManager = new FeatureManager();
|
|
$featureManager->registerFeature( 'featureA', [] );
|
|
$featureManager->registerFeature( 'featureA', [] );
|
|
}
|
|
|
|
/**
|
|
* @covers ::isFeatureEnabled
|
|
*/
|
|
public function testIsFeatureEnabled() {
|
|
$featureManager = new FeatureManager();
|
|
$featureManager->registerSimpleRequirement( 'foo', false );
|
|
$featureManager->registerFeature( 'requiresFoo', 'foo' );
|
|
|
|
$this->assertFalse(
|
|
$featureManager->isFeatureEnabled( 'requiresFoo' ),
|
|
'A feature is disabled when the requirement that it requires is disabled.'
|
|
);
|
|
|
|
// ---
|
|
|
|
$featureManager->registerSimpleRequirement( 'bar', true );
|
|
$featureManager->registerSimpleRequirement( 'baz', true );
|
|
|
|
$featureManager->registerFeature( 'requiresFooBar', [ 'foo', 'bar' ] );
|
|
$featureManager->registerFeature( 'requiresBarBaz', [ 'bar', 'baz' ] );
|
|
|
|
$this->assertFalse(
|
|
$featureManager->isFeatureEnabled( 'requiresFooBar' ),
|
|
'A feature is disabled when at least one requirement that it requires is disabled.'
|
|
);
|
|
|
|
$this->assertTrue( $featureManager->isFeatureEnabled( 'requiresBarBaz' ) );
|
|
}
|
|
|
|
/**
|
|
* @covers ::isFeatureEnabled
|
|
*/
|
|
public function testIsFeatureEnabledThrowsExceptionWhenFeatureIsntRegistered() {
|
|
$this->expectException( \InvalidArgumentException::class );
|
|
|
|
$featureManager = new FeatureManager();
|
|
$featureManager->isFeatureEnabled( 'foo' );
|
|
}
|
|
}
|