mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-12-18 10:51:10 +00:00
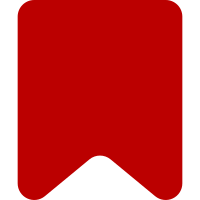
While our implementation of night mode is in beta, we want to respect the existing night mode gadget and disable night mode in favor of the gadget, providing a notice with an option to disable the gadget and reload the page Additionally, raise the max bundle size to account for the additional code added Note: the tests still aren't exactly where I'd like them to be, but hopefully they raise confidence a little bit with reviewing this patch Additional changes: * Upgrade to latest version of TypeScript types and remove several @ts-ignore statements Bug: T365083 Change-Id: I9583ee7ebf8c810ddd504193d568034c954d28f2
132 lines
3.5 KiB
JavaScript
132 lines
3.5 KiB
JavaScript
const {
|
|
isNightModeGadgetEnabled,
|
|
disableNightModeForGadget,
|
|
alterDisableLink,
|
|
alterExclusionMessage
|
|
} = require( '../../../resources/skins.vector.js/disableNightModeIfGadget.js' );
|
|
|
|
describe( 'isNightModeGadgetEnabled', () => {
|
|
beforeEach( () => {
|
|
// https://github.com/wikimedia/mw-node-qunit/pull/38
|
|
mw.loader.getState = () => null;
|
|
} );
|
|
|
|
it( 'should return false if no gadgets are installed', () => {
|
|
expect( isNightModeGadgetEnabled() ).toBeFalsy();
|
|
} );
|
|
|
|
it( 'should return false if the gadgets are installed but not enabled', () => {
|
|
// https://github.com/wikimedia/mw-node-qunit/pull/38
|
|
mw.loader.getState = () => 'registered';
|
|
|
|
expect( isNightModeGadgetEnabled() ).toBeFalsy();
|
|
} );
|
|
|
|
it( 'should return true if the gadgets are enabled', () => {
|
|
// https://github.com/wikimedia/mw-node-qunit/pull/38
|
|
mw.loader.getState = () => 'ready';
|
|
|
|
expect( isNightModeGadgetEnabled() ).toBeTruthy();
|
|
} );
|
|
} );
|
|
|
|
describe( 'disableNightModeForGadget', () => {
|
|
beforeEach( () => {
|
|
document.documentElement.classList.remove( 'skin-theme-clientpref--excluded' );
|
|
document.documentElement.classList.remove( 'skin-theme-clientpref-night' );
|
|
document.documentElement.classList.remove( 'skin-theme-clientpref-os' );
|
|
} );
|
|
|
|
it( 'should disable night mode', () => {
|
|
document.documentElement.classList.add( 'skin-theme-clientpref-night' );
|
|
|
|
disableNightModeForGadget();
|
|
|
|
expect( document.documentElement.classList.contains( 'skin-theme-clientpref-night' ) ).toBeFalsy();
|
|
} );
|
|
|
|
it( 'should disable automatic mode', () => {
|
|
document.documentElement.classList.add( 'skin-theme-clientpref-os' );
|
|
|
|
disableNightModeForGadget();
|
|
|
|
expect( document.documentElement.classList.contains( 'skin-theme-clientpref-os' ) ).toBeFalsy();
|
|
} );
|
|
|
|
it( 'should add the excluded class', () => {
|
|
disableNightModeForGadget();
|
|
|
|
expect( document.documentElement.classList.contains( 'skin-theme-clientpref--excluded' ) ).toBeTruthy();
|
|
} );
|
|
} );
|
|
|
|
describe( 'alterDisableLink', () => {
|
|
it( 'should leave the surrounding element unaltered', () => {
|
|
const p = document.createElement( 'p' );
|
|
const a = document.createElement( 'a' );
|
|
p.appendChild( a );
|
|
|
|
p.textContent = 'test';
|
|
|
|
alterDisableLink( p );
|
|
|
|
expect( p.textContent ).toBe( 'test' );
|
|
} );
|
|
|
|
it( 'should strip the title and href attributes', () => {
|
|
const p = document.createElement( 'p' );
|
|
const a = document.createElement( 'a' );
|
|
p.appendChild( a );
|
|
|
|
a.href = 'test.com';
|
|
a.title = 'test';
|
|
|
|
alterDisableLink( p );
|
|
|
|
expect( a.href ).toBe( '' );
|
|
expect( a.title ).toBe( '' );
|
|
} );
|
|
|
|
it( 'should make the link display inline', () => {
|
|
const p = document.createElement( 'p' );
|
|
const a = document.createElement( 'a' );
|
|
p.appendChild( a );
|
|
|
|
alterDisableLink( p );
|
|
|
|
expect( a.style.display ).toBe( 'inline' );
|
|
} );
|
|
|
|
// actual click test to be added after https://github.com/wikimedia/mw-node-qunit/pull/39
|
|
} );
|
|
|
|
describe( 'alterExclusionMessage', () => {
|
|
beforeEach( () => {
|
|
jest.spyOn( mw.loader, 'using' ).mockImplementation( () => ( {
|
|
then: ( fn ) => fn()
|
|
} ) );
|
|
|
|
// https://github.com/wikimedia/mw-node-qunit/pull/40
|
|
jest.spyOn( mw, 'message' ).mockImplementation( () => ( {
|
|
parseDom: () => ( {
|
|
appendTo: () => {}
|
|
} )
|
|
} ) );
|
|
} );
|
|
|
|
afterEach( () => {
|
|
jest.restoreAllMocks();
|
|
} );
|
|
|
|
it( 'should remove the existing text from the notice', () => {
|
|
const p = document.createElement( 'p' );
|
|
document.documentElement.appendChild( p );
|
|
p.className = 'exclusion-notice';
|
|
p.textContent = 'test';
|
|
|
|
alterExclusionMessage();
|
|
|
|
expect( p.textContent ).toBe( '' );
|
|
} );
|
|
} );
|