mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-12-03 19:57:19 +00:00
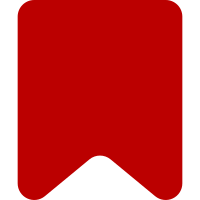
Move A/B test code to AB.js Consolidate the show/hide code spread across scrollObserver and stickyHeader by adding a show and hide function. This is needed to fix T296680 Change-Id: Ia2e0c50278df0dfc1600610f281be20f4cc755c2
66 lines
2 KiB
JavaScript
66 lines
2 KiB
JavaScript
// Enable Vector features limited to ES6 browse
|
|
const
|
|
searchToggle = require( './searchToggle.js' ),
|
|
stickyHeader = require( './stickyHeader.js' ),
|
|
scrollObserver = require( './scrollObserver.js' ),
|
|
AB = require( './AB.js' );
|
|
|
|
/**
|
|
* @return {void}
|
|
*/
|
|
const main = () => {
|
|
// Initialize the search toggle for the main header only. The sticky header
|
|
// toggle is initialized after wvui search loads.
|
|
const searchToggleElement = document.querySelector( '.mw-header .search-toggle' );
|
|
if ( searchToggleElement ) {
|
|
searchToggle( searchToggleElement );
|
|
}
|
|
|
|
// Get the A/B test config for sticky header if enabled.
|
|
const
|
|
FEATURE_TEST_GROUP = 'stickyHeaderEnabled',
|
|
testConfig = AB.getEnabledExperiment(),
|
|
stickyConfig = testConfig &&
|
|
// @ts-ignore
|
|
testConfig.experimentName === stickyHeader.STICKY_HEADER_EXPERIMENT_NAME ?
|
|
testConfig : null,
|
|
// Note that the default test group is set to experience the feature by default.
|
|
// @ts-ignore
|
|
testGroup = stickyConfig ? stickyConfig.group : FEATURE_TEST_GROUP,
|
|
targetElement = stickyHeader.header,
|
|
targetIntersection = stickyHeader.stickyIntersection,
|
|
isStickyHeaderAllowed = stickyHeader.isStickyHeaderAllowed() &&
|
|
testGroup !== 'unsampled' && AB.isInTestGroup( testGroup, FEATURE_TEST_GROUP );
|
|
|
|
// Fire the A/B test enrollment hook.
|
|
AB.initAB( testGroup );
|
|
|
|
// Set up intersection observer for sticky header functionality and firing scroll event hooks
|
|
// for event logging if AB test is enabled.
|
|
const observer = scrollObserver.initScrollObserver(
|
|
() => {
|
|
if ( targetElement && isStickyHeaderAllowed ) {
|
|
stickyHeader.show();
|
|
}
|
|
scrollObserver.fireScrollHook( 'down' );
|
|
},
|
|
() => {
|
|
if ( targetElement && isStickyHeaderAllowed ) {
|
|
stickyHeader.hide();
|
|
}
|
|
scrollObserver.fireScrollHook( 'up' );
|
|
}
|
|
|
|
);
|
|
|
|
if ( isStickyHeaderAllowed ) {
|
|
stickyHeader.initStickyHeader( observer );
|
|
} else if ( targetIntersection ) {
|
|
observer.observe( targetIntersection );
|
|
}
|
|
};
|
|
|
|
module.exports = {
|
|
main: main
|
|
};
|