mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/Vector.git
synced 2024-11-13 17:57:06 +00:00
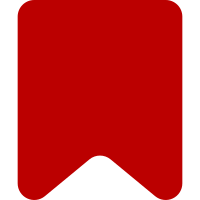
The article ID shouldn't matter for display of table of contents unless the A/B test is enabled. This also has the added benefit of making the content provider work with table of contents on articles again (content provider articles always have a page id of 0) Change-Id: I6af5e1476827828d0974ecbb110476ac1e5dc0c9
151 lines
3.8 KiB
PHP
151 lines
3.8 KiB
PHP
<?php
|
|
|
|
namespace Vector;
|
|
|
|
use MediaWiki\MediaWikiServices;
|
|
|
|
/**
|
|
* @ingroup Skins
|
|
* @package Vector
|
|
* @internal
|
|
*/
|
|
class SkinVector22 extends SkinVector {
|
|
private const TOC_AB_TEST_NAME = 'skin-vector-toc-experiment';
|
|
|
|
/**
|
|
* Updates the constructor to conditionally disable table of contents in article
|
|
* body. Note, the constructor can only check feature flags that do not vary on
|
|
* whether the user is logged in e.g. features with the 'default' key set.
|
|
* @inheritDoc
|
|
*/
|
|
public function __construct( array $options ) {
|
|
if ( !$this->isTOCABTestEnabled() ) {
|
|
$options['toc'] = !$this->isTableOfContentsVisibleInSidebar();
|
|
}
|
|
|
|
parent::__construct( $options );
|
|
}
|
|
|
|
/**
|
|
* @internal
|
|
* @return bool
|
|
*/
|
|
public function isTOCABTestEnabled(): bool {
|
|
$experimentConfig = $this->getConfig()->get( Constants::CONFIG_WEB_AB_TEST_ENROLLMENT );
|
|
|
|
return $experimentConfig['name'] === self::TOC_AB_TEST_NAME &&
|
|
$experimentConfig['enabled'] &&
|
|
MediaWikiServices::getInstance()->hasService( Constants::WEB_AB_TEST_ARTICLE_ID_FACTORY_SERVICE );
|
|
}
|
|
|
|
/**
|
|
* Returns whether or not the table of contents is enabled through
|
|
* FeatureManager.
|
|
*
|
|
* @internal
|
|
* @return bool
|
|
*/
|
|
public function isTOCEnabled() {
|
|
$featureManager = VectorServices::getFeatureManager();
|
|
|
|
return $featureManager->isFeatureEnabled( Constants::FEATURE_TABLE_OF_CONTENTS );
|
|
}
|
|
|
|
/**
|
|
* Determines if the Table of Contents should be visible.
|
|
* TOC is visible on main namespaces except for the Main Page
|
|
* when the feature flag is on.
|
|
*
|
|
* @internal
|
|
* @return bool
|
|
*/
|
|
public function isTableOfContentsVisibleInSidebar(): bool {
|
|
$title = $this->getTitle();
|
|
|
|
if (
|
|
!$title ||
|
|
$title->isMainPage()
|
|
) {
|
|
return false;
|
|
}
|
|
|
|
if ( $this->isTOCABTestEnabled() ) {
|
|
return $title->getArticleID() !== 0;
|
|
}
|
|
|
|
return $this->isTOCEnabled();
|
|
}
|
|
|
|
/**
|
|
* Annotates table of contents data with Vector-specific information.
|
|
*
|
|
* @param array $tocData
|
|
* @return array
|
|
*/
|
|
private function getTocData( array $tocData ): array {
|
|
// If the table of contents has no items, we won't output it.
|
|
// empty array is interpreted by Mustache as falsey.
|
|
if ( empty( $tocData ) || empty( $tocData[ 'array-sections' ] ) ) {
|
|
return [];
|
|
}
|
|
|
|
// Populate button labels for collapsible TOC sections
|
|
foreach ( $tocData[ 'array-sections' ] as &$section ) {
|
|
if ( $section['is-top-level-section'] && $section['is-parent-section'] ) {
|
|
$section['vector-button-label'] =
|
|
$this->msg( 'vector-toc-toggle-button-label', $section['line'] )->text();
|
|
}
|
|
}
|
|
|
|
return array_merge( $tocData, [
|
|
'is-vector-toc-beginning-enabled' => $this->getConfig()->get(
|
|
'VectorTableOfContentsBeginning'
|
|
),
|
|
'vector-is-collapse-sections-enabled' =>
|
|
$tocData[ 'number-section-count'] >= $this->getConfig()->get(
|
|
'VectorTableOfContentsCollapseAtCount'
|
|
)
|
|
] );
|
|
}
|
|
|
|
/**
|
|
* Temporary function while we deprecate SkinVector class.
|
|
*
|
|
* @return bool
|
|
*/
|
|
protected function isLegacy(): bool {
|
|
return false;
|
|
}
|
|
|
|
/**
|
|
* @return array
|
|
*/
|
|
public function getTemplateData(): array {
|
|
$featureManager = VectorServices::getFeatureManager();
|
|
$parentData = parent::getTemplateData();
|
|
|
|
$parentData['data-toc'] = $this->getTocData( $parentData['data-toc'] ?? [] );
|
|
|
|
if ( !$this->isTableOfContentsVisibleInSidebar() ) {
|
|
unset( $parentData['data-toc'] );
|
|
}
|
|
return $parentData + [
|
|
'data-vector-sticky-header' => $featureManager->isFeatureEnabled(
|
|
Constants::FEATURE_STICKY_HEADER
|
|
) ? $this->getStickyHeaderData(
|
|
$this->getSearchData(
|
|
$parentData['data-search-box'],
|
|
// Collapse inside search box is disabled.
|
|
false,
|
|
false,
|
|
'vector-sticky-search-form',
|
|
false
|
|
),
|
|
$featureManager->isFeatureEnabled(
|
|
Constants::FEATURE_STICKY_HEADER_EDIT
|
|
)
|
|
) : false,
|
|
];
|
|
}
|
|
}
|