mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/MinervaNeue
synced 2024-11-12 00:48:46 +00:00
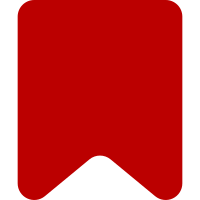
This is programmatic output from python3 scripts/migrate.py This will result in a Minerva skin dependent on MobileFrontend. Post merge we will rename message keys to have minerva- prefix Bug: T166748 Change-Id: Iff1f7e63e796cc5d4a6d2ab0370e0c33248d2fce
63 lines
1.1 KiB
PHP
63 lines
1.1 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Minerva;
|
|
|
|
use IContextSource;
|
|
use Title;
|
|
use User;
|
|
|
|
class SkinUserPageHelper {
|
|
/**
|
|
* @var Title
|
|
*/
|
|
private $title;
|
|
/**
|
|
* @var bool
|
|
*/
|
|
private $fetchedData = false;
|
|
|
|
/**
|
|
* @var User
|
|
*/
|
|
private $pageUser;
|
|
|
|
/**
|
|
* SkinMinervaUserPageHelper constructor.
|
|
* @param IContextSource $context
|
|
*/
|
|
public function __construct( IContextSource $context ) {
|
|
$this->title = $context->getTitle();
|
|
}
|
|
|
|
/**
|
|
* Fetch user data and store locally for perfomance improvement
|
|
*/
|
|
private function fetchData() {
|
|
if ( $this->fetchedData === false ) {
|
|
if ( $this->title->inNamespace( NS_USER ) && !$this->title->isSubpage() ) {
|
|
$pageUserId = User::idFromName( $this->title->getText() );
|
|
if ( $pageUserId ) {
|
|
$this->pageUser = User::newFromId( $pageUserId );
|
|
}
|
|
}
|
|
$this->fetchedData = true;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @return User|null
|
|
*/
|
|
public function getPageUser() {
|
|
$this->fetchData();
|
|
return $this->pageUser;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function isUserPage() {
|
|
$this->fetchData();
|
|
return $this->pageUser !== null;
|
|
}
|
|
}
|