mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/MinervaNeue
synced 2024-11-16 18:58:45 +00:00
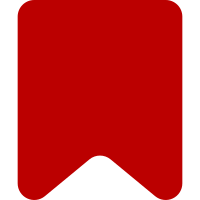
For skins, all templates should be in the same folder. This is for security reasons, to limit access of templates to files that are not templates. This also adds consistency with other skins where templates are all in the same subdirectory. It also allows us to reduce the creation of TemplateParser instances. Note: All styles and scripts should be in the resources folder but this is not rectified by this patchset. Will be done in follow ups following more discussion. This begins this work in the least disruptive way possible and drops the README note to avoid this pattern growing. Bug: T292558 Change-Id: I4c2e115451c0a76c742734730712814c1f1d838d
99 lines
2.9 KiB
PHP
99 lines
2.9 KiB
PHP
<?php
|
|
/**
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*
|
|
* @file
|
|
*/
|
|
namespace MediaWiki\Minerva\Menu\PageActions;
|
|
|
|
use MessageLocalizer;
|
|
use MinervaUI;
|
|
use MWException;
|
|
|
|
/**
|
|
* Director responsible for building Page Actions menu.
|
|
* This class is stateless.
|
|
*/
|
|
final class PageActionsDirector {
|
|
|
|
/**
|
|
* @var ToolbarBuilder
|
|
*/
|
|
private $toolbarBuilder;
|
|
|
|
/**
|
|
* @var IOverflowBuilder
|
|
*/
|
|
private $overflowBuilder;
|
|
|
|
/**
|
|
* @var MessageLocalizer
|
|
*/
|
|
private $messageLocalizer;
|
|
|
|
/**
|
|
* Director responsible for Page Actions menu building
|
|
*
|
|
* @param ToolbarBuilder $toolbarBuilder The toolbar builder
|
|
* @param IOverflowBuilder $overflowBuilder The overflow menu builder
|
|
* @param MessageLocalizer $messageLocalizer Message localizer used to translate texts
|
|
*/
|
|
public function __construct(
|
|
ToolbarBuilder $toolbarBuilder,
|
|
IOverflowBuilder $overflowBuilder,
|
|
MessageLocalizer $messageLocalizer
|
|
) {
|
|
$this->toolbarBuilder = $toolbarBuilder;
|
|
$this->overflowBuilder = $overflowBuilder;
|
|
$this->messageLocalizer = $messageLocalizer;
|
|
}
|
|
|
|
/**
|
|
* Build the menu data array that can be passed to views/javascript
|
|
* @param array $toolbox An array of common toolbox items from the sidebar menu
|
|
* @param array $actions An array of actions usually bucketed under the more menu
|
|
* @return array
|
|
* @throws MWException
|
|
*/
|
|
public function buildMenu( array $toolbox, array $actions ): array {
|
|
$toolbar = $this->toolbarBuilder->getGroup();
|
|
$overflowMenu = $this->overflowBuilder->getGroup( $toolbox, $actions );
|
|
|
|
$menu = [
|
|
'toolbar' => $toolbar->getEntries()
|
|
];
|
|
if ( $overflowMenu->hasEntries() ) {
|
|
// See includes/Skins/components/ToggleList.
|
|
$menu[ 'overflowMenu' ] = [
|
|
'item-id' => 'page-actions-overflow',
|
|
'checkboxID' => 'page-actions-overflow-checkbox',
|
|
'toggleID' => 'page-actions-overflow-toggle',
|
|
'listID' => $overflowMenu->getId(),
|
|
'toggleClass' => MinervaUI::iconClass(
|
|
'ellipsis',
|
|
'element',
|
|
'mw-ui-icon-with-label-desktop' ),
|
|
'listClass' => 'page-actions-overflow-list toggle-list__list--drop-down',
|
|
'text' => $this->messageLocalizer->msg( 'minerva-page-actions-overflow' ),
|
|
'analyticsEventName' => 'ui.overflowmenu',
|
|
'items' => $overflowMenu->getEntries()
|
|
];
|
|
}
|
|
return $menu;
|
|
}
|
|
|
|
}
|