mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/MinervaNeue
synced 2024-11-15 02:13:49 +00:00
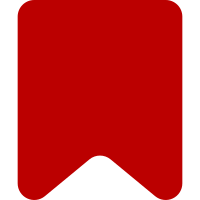
The $hasLangauges and $hasVariants checks were used in couple places, which lead to the same code used in many places. Following the DRY rule, let's implement a Service that can do that check, and use that service everywhere in code. Bug: T224735 Change-Id: I46d58758356e870c408a74b2c087a42d6ad0ddea
80 lines
2.1 KiB
PHP
80 lines
2.1 KiB
PHP
<?php
|
|
|
|
namespace Tests\MediaWiki\Minerva;
|
|
|
|
use MediaWiki\Minerva\LanguagesHelper;
|
|
use PHPUnit\Framework\MockObject\Invocation;
|
|
|
|
/**
|
|
* Class SkinMinervaTest
|
|
* @package Tests\MediaWiki\Minerva
|
|
* @group MinervaNeue
|
|
* @coversDefaultClass \MediaWiki\Minerva\LanguagesHelper
|
|
*/
|
|
class LanguagesHelperTest extends \MediaWikiUnitTestCase {
|
|
|
|
/**
|
|
* Build test Output object
|
|
* @param array $langLinks
|
|
* @return \OutputPage
|
|
*/
|
|
private function getOutput( array $langLinks ) {
|
|
$out = $this->getMock(
|
|
\OutputPage::class,
|
|
[ 'getLanguageLinks' ],
|
|
[],
|
|
'',
|
|
false
|
|
);
|
|
$out->expects( $this->once() )
|
|
->method( 'getLanguageLinks' )
|
|
->willReturn( $langLinks );
|
|
|
|
return $out;
|
|
}
|
|
|
|
/**
|
|
* Build test Title object
|
|
* @param $hasVariants
|
|
* @param Invocation|null $matcher
|
|
* @return \Title
|
|
*/
|
|
private function getTitle( $hasVariants, Invocation $matcher = null ) {
|
|
$languageMock = $this->getMock( \Language::class, [ 'hasVariants' ], [], '', false );
|
|
$languageMock->expects( $matcher ?? $this->any() )
|
|
->method( 'hasVariants' )
|
|
->willReturn( $hasVariants );
|
|
|
|
$title = $this->getMock( \Title::class, [ 'getPageLanguage' ] );
|
|
$title->expects( $matcher ?? $this->any() )
|
|
->method( 'getPageLanguage' )
|
|
->willReturn( $languageMock );
|
|
|
|
return $title;
|
|
}
|
|
|
|
/**
|
|
* @covers ::__construct
|
|
* @covers ::doesTitleHasLanguagesOrVariants
|
|
*/
|
|
public function testReturnsWhenOutputPageHasLangLinks() {
|
|
$helper = new LanguagesHelper( $this->getOutput( [ 'pl:StronaTestowa', 'en:TestPage' ] ) );
|
|
|
|
$this->assertTrue( $helper->doesTitleHasLanguagesOrVariants( $this->getTitle( false ) ) );
|
|
$this->assertTrue( $helper->doesTitleHasLanguagesOrVariants( $this->getTitle( true ) ) );
|
|
}
|
|
|
|
/**
|
|
* @covers ::__construct
|
|
* @covers ::doesTitleHasLanguagesOrVariants
|
|
*/
|
|
public function testReturnsWhenOutputDoesNotHaveLangLinks() {
|
|
$helper = new LanguagesHelper( $this->getOutput( [] ) );
|
|
|
|
$this->assertFalse( $helper->doesTitleHasLanguagesOrVariants(
|
|
$this->getTitle( false ), $this->once() ) );
|
|
$this->assertTrue( $helper->doesTitleHasLanguagesOrVariants(
|
|
$this->getTitle( true ), $this->once() ) );
|
|
}
|
|
}
|