mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/MinervaNeue
synced 2024-11-17 03:08:12 +00:00
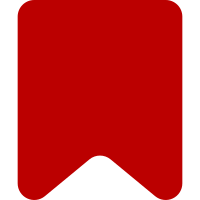
Add a skin-night-mode-page-disabled class to the HTML element when a page was disabled by the new MinervaNightModeOptions configuration flag. Bug: T356653 Change-Id: I7a6582ef8f66e78cc6f07da06bc4d2a3277cfcf0
101 lines
2.6 KiB
JavaScript
101 lines
2.6 KiB
JavaScript
'use strict';
|
|
const reportIfNightModeWasDisabledOnPage = require(
|
|
'../../../resources/skins.minerva.scripts/reportIfNightModeWasDisabledOnPage.js'
|
|
);
|
|
const nightModeDisabledDoc = document.createElement( 'html' );
|
|
nightModeDisabledDoc.setAttribute( 'class', 'skin-night-mode-page-disabled' );
|
|
|
|
const userOptionsEnabled = new Map();
|
|
userOptionsEnabled.set( 'minerva-night-mode', '1' );
|
|
const userOptionsDisabled = new Map();
|
|
userOptionsDisabled.set( 'minerva-night-mode', '0' );
|
|
const userOptionsAutomatic = new Map();
|
|
userOptionsAutomatic.set( 'minerva-night-mode', '2' );
|
|
|
|
const notify = () => {};
|
|
const msg = () => {};
|
|
|
|
describe( 'reportIfNightModeWasDisabledOnPage.js', () => {
|
|
it( 'returns false if no skin-night-mode-page-disabled class is on the document element', () => {
|
|
global.mw = {};
|
|
expect( reportIfNightModeWasDisabledOnPage( document.createElement( 'html' ) ) ).toBe( false );
|
|
} );
|
|
|
|
it( 'shows notification for logged in users with night mode preferred, reading from options', () => {
|
|
global.mw = {
|
|
msg,
|
|
notify,
|
|
user: {
|
|
isNamed: () => true,
|
|
options: userOptionsEnabled
|
|
}
|
|
};
|
|
expect( reportIfNightModeWasDisabledOnPage( nightModeDisabledDoc ) ).toBe( true );
|
|
} );
|
|
|
|
it( 'shows no notification for logged in users with night mode disabled, reading from options', () => {
|
|
global.mw = {
|
|
msg,
|
|
notify,
|
|
user: {
|
|
isNamed: () => true,
|
|
options: userOptionsDisabled
|
|
}
|
|
};
|
|
expect( reportIfNightModeWasDisabledOnPage( nightModeDisabledDoc ) ).toBe( false );
|
|
} );
|
|
|
|
it( 'respects automatic mode when displaying a notification for logged in users', () => {
|
|
global.mw = {
|
|
msg,
|
|
notify,
|
|
user: {
|
|
isNamed: () => true,
|
|
options: userOptionsAutomatic
|
|
}
|
|
};
|
|
window.matchMedia = () => {
|
|
return {
|
|
matches: true
|
|
};
|
|
};
|
|
expect( reportIfNightModeWasDisabledOnPage( nightModeDisabledDoc ) ).toBe( true );
|
|
window.matchMedia = () => {
|
|
return {
|
|
matches: false
|
|
};
|
|
};
|
|
expect( reportIfNightModeWasDisabledOnPage( nightModeDisabledDoc ) ).toBe( false );
|
|
} );
|
|
|
|
it( 'reads from cookie for anons', () => {
|
|
global.mw = {
|
|
msg,
|
|
notify,
|
|
cookie: {
|
|
get: () => 'skin-night-mode-clientpref-1'
|
|
},
|
|
user: {
|
|
isNamed: () => false,
|
|
options: userOptionsDisabled
|
|
}
|
|
};
|
|
expect( reportIfNightModeWasDisabledOnPage( nightModeDisabledDoc ) ).toBe( true );
|
|
} );
|
|
|
|
it( 'reads from cookie for anons, can handle unset cookie', () => {
|
|
global.mw = {
|
|
msg,
|
|
notify,
|
|
cookie: {
|
|
get: () => null
|
|
},
|
|
user: {
|
|
isNamed: () => false,
|
|
options: userOptionsDisabled
|
|
}
|
|
};
|
|
expect( reportIfNightModeWasDisabledOnPage( nightModeDisabledDoc ) ).toBe( false );
|
|
} );
|
|
} );
|