mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/MinervaNeue
synced 2024-11-15 02:13:49 +00:00
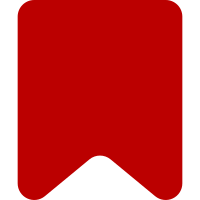
The MobileFrontend dependency in Minerva is problematic. Code that Minerva needs should live in core. MobileFrontend should load code on all skins when they operate on a mobile domain. This eslint check reminds developers of this in a hope it encourages more upstreaming to core when possible. Of course disabling is also an option, but this check will at least make us aware of when we are moving further away from the goal. Change-Id: I62183c9aefc81053e4ad81fb746decef2dd24b44
110 lines
3.2 KiB
JavaScript
110 lines
3.2 KiB
JavaScript
( function ( M ) {
|
|
var
|
|
mobile = M.require( 'mobile.startup' ),
|
|
ToggleList = require( '../../components/ToggleList/ToggleList.js' ),
|
|
downloadPageAction = require( './downloadPageAction.js' ).downloadPageAction,
|
|
Icon = mobile.Icon,
|
|
page = mobile.currentPage(),
|
|
/** The top level menu. */
|
|
toolbarSelector = '.page-actions-menu',
|
|
/** The secondary overflow submenu component container. */
|
|
overflowSubmenuSelector = '#page-actions-overflow',
|
|
overflowListSelector = '.toggle-list__list';
|
|
|
|
/**
|
|
* @param {Window} window
|
|
* @param {Element} toolbar
|
|
* @return {void}
|
|
*/
|
|
function bind( window, toolbar ) {
|
|
var overflowSubmenu = toolbar.querySelector( overflowSubmenuSelector );
|
|
if ( overflowSubmenu ) {
|
|
ToggleList.bind( window, overflowSubmenu );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @param {Window} window
|
|
* @param {Element} toolbar
|
|
* @return {void}
|
|
*/
|
|
function render( window, toolbar ) {
|
|
var overflowList = toolbar.querySelector( overflowListSelector );
|
|
renderEditButton();
|
|
renderDownloadButton( window, overflowList );
|
|
}
|
|
|
|
/**
|
|
* Initialize page edit action link (#ca-edit)
|
|
*
|
|
* Mark the edit link as disabled if the user is not actually able to edit the page for some
|
|
* reason (e.g. page is protected or user is blocked).
|
|
*
|
|
* Note that the link is still clickable, but clicking it will probably open a view-source
|
|
* form or display an error message, rather than open an edit form.
|
|
*
|
|
* FIXME: Review this code as part of T206262
|
|
*
|
|
* @ignore
|
|
*/
|
|
function renderEditButton() {
|
|
var
|
|
// FIXME: create a utility method to generate class names instead of
|
|
// constructing temporary objects. This affects disabledEditIcon,
|
|
// enabledEditIcon, enabledEditIcon, and disabledClass and
|
|
// a number of other places in the code base.
|
|
disabledEditIcon = new Icon( {
|
|
name: 'edit',
|
|
glyphPrefix: 'minerva'
|
|
} ),
|
|
enabledEditIcon = new Icon( {
|
|
name: 'edit-enabled',
|
|
glyphPrefix: 'minerva'
|
|
} ),
|
|
enabledClass = enabledEditIcon.getGlyphClassName(),
|
|
disabledClass = disabledEditIcon.getGlyphClassName();
|
|
|
|
if ( mw.config.get( 'wgMinervaReadOnly' ) ) {
|
|
// eslint-disable-next-line no-jquery/no-global-selector
|
|
$( '#ca-edit' )
|
|
.removeClass( enabledClass )
|
|
.addClass( disabledClass );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Initialize and inject the download button
|
|
*
|
|
* There are many restrictions when we can show the download button, this function should handle
|
|
* all device/os/operating system related checks and if device supports printing it will inject
|
|
* the Download icon
|
|
* @param {Window} window
|
|
* @param {Element|null} overflowList
|
|
* @return {void}
|
|
*/
|
|
function renderDownloadButton( window, overflowList ) {
|
|
var $downloadAction = downloadPageAction( page,
|
|
mw.config.get( 'wgMinervaDownloadNamespaces', [] ), window, !!overflowList );
|
|
|
|
if ( $downloadAction ) {
|
|
if ( overflowList ) {
|
|
$downloadAction.appendTo( overflowList );
|
|
} else {
|
|
$downloadAction.insertAfter( '.page-actions-menu__list-item:first-child' );
|
|
}
|
|
|
|
mw.track( 'minerva.downloadAsPDF', {
|
|
action: 'buttonVisible'
|
|
} );
|
|
}
|
|
}
|
|
|
|
module.exports = {
|
|
selector: toolbarSelector,
|
|
bind: bind,
|
|
render: render
|
|
};
|
|
|
|
// eslint-disable-next-line no-restricted-properties
|
|
}( mw.mobileFrontend ) );
|