mirror of
https://gerrit.wikimedia.org/r/mediawiki/skins/MinervaNeue
synced 2024-12-03 18:26:16 +00:00
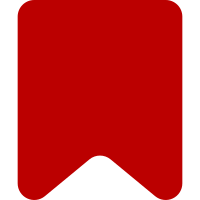
Placing the text overflow on the span instead of the anchor element prevents issues like T287522#7272991 from occurring. Additionally: * Uses the `text-overflow` mixin on every toggle list item label instead of just the user link item as it simplifies the logic and presumably we'd want any text that overflows the menu to be handled the same. * Changes the anchor element to use flexbox/align-items to vertically center instead of relying on `vertical-align` both the span and the icon. Eventually, this should be put into core (see I029f97ba9d5e7f46c8aa175d9a6bbb45ef9615df) but we have to remove all the overrides that use vertical-align first. Bug: T287522 Change-Id: Ie0fbff9dfaf8444c76125df52931a687730b4ad1
94 lines
2.3 KiB
PHP
94 lines
2.3 KiB
PHP
<?php
|
|
/**
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
namespace MediaWiki\Minerva\Menu\Entries;
|
|
|
|
use MinervaUI;
|
|
use Title;
|
|
use User;
|
|
|
|
final class ProfileMenuEntry implements IProfileMenuEntry {
|
|
/**
|
|
* @var User
|
|
*/
|
|
private $user;
|
|
|
|
/**
|
|
* Code used to track clicks on the link to profile page
|
|
* @var string|null
|
|
*/
|
|
private $profileTrackingCode = null;
|
|
|
|
/**
|
|
* Custom profile URL, can be used to override where the profile link href
|
|
* @var string|null
|
|
*/
|
|
private $customProfileURL = null;
|
|
|
|
/**
|
|
* Custom profile label, can be used to override the profile label
|
|
* @var string|null
|
|
*/
|
|
private $customProfileLabel = null;
|
|
|
|
/**
|
|
* @param User $user Currently logged in user/anon
|
|
*/
|
|
public function __construct( User $user ) {
|
|
$this->user = $user;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getName() {
|
|
return 'profile';
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function overrideProfileURL( $customURL, $customLabel = null, $trackingCode = null ) {
|
|
$this->customProfileURL = $customURL;
|
|
$this->customProfileLabel = $customLabel;
|
|
$this->profileTrackingCode = $trackingCode;
|
|
return $this;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getCSSClasses(): array {
|
|
return [];
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getComponents(): array {
|
|
$username = $this->user->getName();
|
|
return [ [
|
|
'text' => $this->customProfileLabel ?? $username,
|
|
'href' => $this->customProfileURL ?? Title::newFromText( $username, NS_USER )->getLocalURL(),
|
|
'class' => MinervaUI::iconClass( 'userAvatar-base20',
|
|
'before', 'primary-action', 'wikimedia' ),
|
|
'data-event-name' => 'menu.' . (
|
|
$this->profileTrackingCode ?? self::DEFAULT_PROFILE_TRACKING_CODE )
|
|
] ];
|
|
}
|
|
}
|