mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/WikiEditor
synced 2024-11-12 09:57:16 +00:00
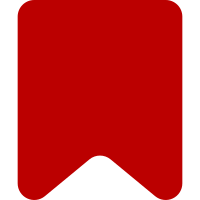
eqeqeq: * Change loose comparisons to strict comparisons where it seems safe to use a strict comparision instead. Mostly comparisons to strings or objects, and comparisons to numbers where the other value is known to be a number, too. E.g. foo == 'string', bar == node, indexOf() != -1. * Add eqeqeq:false to files where there are non-obvious usages left. onevar, quotmark: * Disabled in files with lots of style violations. unused: * Remove unused variables that have no side-effects in their assigned expression. Coding style cleanups on affected lines where trivial. Change-Id: I5db155a632740e24cb52dba2177c7fc35d5aebd5
171 lines
4.7 KiB
JavaScript
171 lines
4.7 KiB
JavaScript
/* Preview module for wikiEditor */
|
|
( function ( $, mw ) {
|
|
/*jshint onevar:false */
|
|
$.wikiEditor.modules.preview = {
|
|
|
|
/**
|
|
* Compatability map
|
|
*/
|
|
browsers: {
|
|
// Left-to-right languages
|
|
ltr: {
|
|
msie: [['>=', 7]],
|
|
firefox: [['>=', 3]],
|
|
opera: [['>=', 9.6]],
|
|
safari: [['>=', 4]]
|
|
},
|
|
// Right-to-left languages
|
|
rtl: {
|
|
msie: [['>=', 8]],
|
|
firefox: [['>=', 3]],
|
|
opera: [['>=', 9.6]],
|
|
safari: [['>=', 4]]
|
|
}
|
|
},
|
|
|
|
/**
|
|
* Internally used functions
|
|
*/
|
|
fn: {
|
|
/**
|
|
* Creates a preview module within a wikiEditor
|
|
* @param context Context object of editor to create module in
|
|
* @param config Configuration object to create module from
|
|
*/
|
|
create: function ( context ) {
|
|
if ( 'initialized' in context.modules.preview ) {
|
|
return;
|
|
}
|
|
context.modules.preview = {
|
|
'initialized': true,
|
|
'previewText': null,
|
|
'changesText': null
|
|
};
|
|
context.modules.preview.$preview = context.fn.addView( {
|
|
'name': 'preview',
|
|
'titleMsg': 'wikieditor-preview-tab',
|
|
'init': function ( context ) {
|
|
// Gets the latest copy of the wikitext
|
|
var wikitext = context.$textarea.textSelection( 'getContents' );
|
|
// Aborts when nothing has changed since the last preview
|
|
if ( context.modules.preview.previewText === wikitext ) {
|
|
return;
|
|
}
|
|
context.modules.preview.$preview.find( '.wikiEditor-preview-contents' ).empty();
|
|
context.modules.preview.$preview.find( '.wikiEditor-preview-loading' ).show();
|
|
$.post(
|
|
mw.util.wikiScript( 'api' ),
|
|
{
|
|
format: 'json',
|
|
action: 'parse',
|
|
title: mw.config.get( 'wgPageName' ),
|
|
text: wikitext,
|
|
prop: 'text',
|
|
pst: ''
|
|
},
|
|
function ( data ) {
|
|
if (
|
|
typeof data.parse === 'undefined' ||
|
|
typeof data.parse.text === 'undefined' ||
|
|
typeof data.parse.text['*'] === 'undefined'
|
|
) {
|
|
return;
|
|
}
|
|
context.modules.preview.previewText = wikitext;
|
|
context.modules.preview.$preview.find( '.wikiEditor-preview-loading' ).hide();
|
|
context.modules.preview.$preview.find( '.wikiEditor-preview-contents' )
|
|
.html( data.parse.text['*'] )
|
|
.find( 'a:not([href^=#])' ).click( false );
|
|
},
|
|
'json'
|
|
);
|
|
}
|
|
} );
|
|
|
|
context.$changesTab = context.fn.addView( {
|
|
'name': 'changes',
|
|
'titleMsg': 'wikieditor-preview-changes-tab',
|
|
'init': function ( context ) {
|
|
// Gets the latest copy of the wikitext
|
|
var wikitext = context.$textarea.textSelection( 'getContents' );
|
|
// Aborts when nothing has changed since the last time
|
|
if ( context.modules.preview.changesText === wikitext ) {
|
|
return;
|
|
}
|
|
context.$changesTab.find( 'table.diff tbody' ).empty();
|
|
context.$changesTab.find( '.wikiEditor-preview-loading' ).show();
|
|
|
|
// Call the API. First PST the input, then diff it
|
|
var postdata = {
|
|
format: 'json',
|
|
action: 'parse',
|
|
onlypst: '',
|
|
text: wikitext
|
|
};
|
|
|
|
$.post( mw.util.wikiScript( 'api' ), postdata, function ( data ) {
|
|
try {
|
|
var postdata2 = {
|
|
format: 'json',
|
|
action: 'query',
|
|
indexpageids: '',
|
|
prop: 'revisions',
|
|
titles: mw.config.get( 'wgPageName' ),
|
|
rvdifftotext: data.parse.text['*'],
|
|
rvprop: ''
|
|
};
|
|
var section = $( '[name="wpSection"]' ).val();
|
|
if ( section !== '' ) {
|
|
postdata2.rvsection = section;
|
|
}
|
|
|
|
$.post( mw.util.wikiScript( 'api' ), postdata2, function ( data ) {
|
|
// Add diff CSS
|
|
mw.loader.load( 'mediawiki.action.history.diff' );
|
|
try {
|
|
var diff = data.query.pages[data.query.pageids[0]]
|
|
.revisions[0].diff['*'];
|
|
context.$changesTab.find( 'table.diff tbody' )
|
|
.html( diff );
|
|
context.$changesTab
|
|
.find( '.wikiEditor-preview-loading' ).hide();
|
|
context.modules.preview.changesText = wikitext;
|
|
} catch ( e ) { } // "blah is undefined" error, ignore
|
|
}, 'json'
|
|
);
|
|
} catch ( e ) { } // "blah is undefined" error, ignore
|
|
}, 'json' );
|
|
}
|
|
} );
|
|
|
|
var loadingMsg = mw.msg( 'wikieditor-preview-loading' );
|
|
context.modules.preview.$preview
|
|
.add( context.$changesTab )
|
|
.append( $( '<div>' )
|
|
.addClass( 'wikiEditor-preview-loading' )
|
|
.append( $( '<img>' )
|
|
.addClass( 'wikiEditor-preview-spinner' )
|
|
.attr( {
|
|
'src': $.wikiEditor.imgPath + 'dialogs/loading.gif',
|
|
'valign': 'absmiddle',
|
|
'alt': loadingMsg,
|
|
'title': loadingMsg
|
|
} )
|
|
)
|
|
.append(
|
|
$( '<span>' ).text( loadingMsg )
|
|
)
|
|
)
|
|
.append( $( '<div>' )
|
|
.addClass( 'wikiEditor-preview-contents' )
|
|
);
|
|
context.$changesTab.find( '.wikiEditor-preview-contents' )
|
|
.html( '<table class="diff"><col class="diff-marker"/><col class="diff-content"/>' +
|
|
'<col class="diff-marker"/><col class="diff-content"/><tbody/></table>' );
|
|
}
|
|
}
|
|
|
|
};
|
|
|
|
}( jQuery, mediaWiki ) );
|