mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/WikiEditor
synced 2024-11-27 17:50:44 +00:00
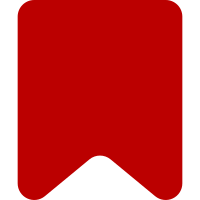
Add following logging events: * preview-realtime-error-stopped: triggered when realtime preview auto-load is stopped. * preview-realtime-reload-error: triggered when realtime preview pane shows an error message and the reload button shown in this message is clicked. * preview-realtime-reload-hover: triggered when the reload button that shows on hover in the realtime preview pane is clicked. * preview-realtime-reload-manual: triggered when the reload button in the realtime preview pane manual bar is clicked. Bug: T306176 Change-Id: I0785b0a8e88125a9bb30ff5c64c8a7c50b556c63
65 lines
2.1 KiB
JavaScript
65 lines
2.1 KiB
JavaScript
/* global RealtimePreview */
|
|
/**
|
|
* @class
|
|
* @constructor
|
|
* @param {RealtimePreview} realtimePreview
|
|
* @param {OO.ui.ButtonWidget} reloadHoverButton
|
|
*/
|
|
function ManualWidget( realtimePreview, reloadHoverButton ) {
|
|
var config = {
|
|
classes: [ 'ext-WikiEditor-ManualWidget' ],
|
|
$element: $( '<a>' )
|
|
};
|
|
ManualWidget.super.call( this, config );
|
|
|
|
// Mixins.
|
|
OO.ui.mixin.AccessKeyedElement.call( this, {} );
|
|
OO.ui.mixin.ButtonElement.call( this, $.extend( {
|
|
$button: this.$element
|
|
}, config ) );
|
|
OO.ui.mixin.IconElement.call( this, { icon: 'reload' } );
|
|
OO.ui.mixin.TitledElement.call( this, {
|
|
title: mw.msg( 'wikieditor-realtimepreview-reload-title' )
|
|
} );
|
|
|
|
this.reloadHoverButton = reloadHoverButton;
|
|
|
|
// UI elements.
|
|
var $reloadLabel = $( '<span>' )
|
|
.text( mw.msg( 'wikieditor-realtimepreview-manual' ) );
|
|
var $reloadButton = $( '<span>' )
|
|
.addClass( 'ext-WikiEditor-realtimepreview-manual-reload' )
|
|
.text( mw.msg( 'wikieditor-realtimepreview-reload' ) );
|
|
this.connect( realtimePreview, {
|
|
click: function () {
|
|
if ( !this.isScreenWideEnough() ) {
|
|
// Do nothing if realtime preview is not visible.
|
|
return;
|
|
}
|
|
// Only refresh the preview if we're enabled.
|
|
if ( this.enabled ) {
|
|
this.doRealtimePreview();
|
|
}
|
|
mw.hook( 'ext.WikiEditor.realtimepreview.reloadManual' ).fire( this );
|
|
}.bind( realtimePreview )
|
|
} );
|
|
this.$element.append( this.$icon, $reloadLabel, $reloadButton );
|
|
}
|
|
|
|
OO.inheritClass( ManualWidget, OO.ui.Widget );
|
|
OO.mixinClass( ManualWidget, OO.ui.mixin.AccessKeyedElement );
|
|
OO.mixinClass( ManualWidget, OO.ui.mixin.ButtonElement );
|
|
OO.mixinClass( ManualWidget, OO.ui.mixin.IconElement );
|
|
OO.mixinClass( ManualWidget, OO.ui.mixin.TitledElement );
|
|
|
|
ManualWidget.prototype.toggle = function ( show ) {
|
|
ManualWidget.parent.prototype.toggle.call( this, show );
|
|
if ( show ) {
|
|
this.reloadHoverButton.$element.remove();
|
|
// Use the same access key as the hover reload button, because this won't ever be displayed at the same time as that.
|
|
this.setAccessKey( mw.msg( 'accesskey-wikieditor-realtimepreview' ) );
|
|
}
|
|
};
|
|
|
|
module.exports = ManualWidget;
|