mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/WikiEditor
synced 2024-09-25 03:10:18 +00:00
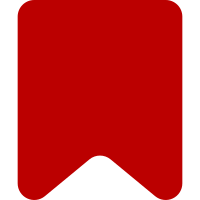
eqeqeq: * Change loose comparisons to strict comparisons where it seems safe to use a strict comparision instead. Mostly comparisons to strings or objects, and comparisons to numbers where the other value is known to be a number, too. E.g. foo == 'string', bar == node, indexOf() != -1. * Add eqeqeq:false to files where there are non-obvious usages left. onevar, quotmark: * Disabled in files with lots of style violations. unused: * Remove unused variables that have no side-effects in their assigned expression. Coding style cleanups on affected lines where trivial. Change-Id: I5db155a632740e24cb52dba2177c7fc35d5aebd5
79 lines
1.9 KiB
JavaScript
79 lines
1.9 KiB
JavaScript
/* Templates Module for wikiEditor */
|
|
/*jshint onevar:false */
|
|
( function ( $ ) { $.wikiEditor.modules.templates = {
|
|
|
|
/**
|
|
* Core Requirements
|
|
*/
|
|
req: [ 'iframe' ],
|
|
/**
|
|
* Object Templates
|
|
*/
|
|
tpl: {
|
|
marker: {
|
|
type: 'template',
|
|
anchor: 'wrap',
|
|
skipDivision: 'realchange',
|
|
afterWrap: function ( node ) {
|
|
$( node ).addClass( 'wikiEditor-template' );
|
|
},
|
|
getAnchor: function ( ca1 ) {
|
|
return $( ca1.parentNode ).is( '.wikiEditor-template' ) ? ca1.parentNode : null;
|
|
}
|
|
}
|
|
},
|
|
/**
|
|
* Event handlers
|
|
*/
|
|
evt: {
|
|
/**
|
|
* @param context
|
|
* @param event
|
|
*/
|
|
mark: function ( context ) {
|
|
// The markers returned by this function are skipped on realchange, so don't regenerate them in that case
|
|
if ( context.modules.highlight.currentScope === 'realchange' ) {
|
|
return;
|
|
}
|
|
// Get references to the markers and tokens from the current context
|
|
var markers = context.modules.highlight.markers;
|
|
var tokens = context.modules.highlight.tokenArray;
|
|
// Use depth-tracking to extract top-level templates from tokens
|
|
var depth = 0, bias, start;
|
|
for ( var i in tokens ) {
|
|
depth += ( bias = tokens[i].label === 'TEMPLATE_BEGIN' ? 1 : ( tokens[i].label === 'TEMPLATE_END' ? -1 : 0 ) );
|
|
if ( bias > 0 && depth === 1 ) {
|
|
// Top-level opening - use offset as start
|
|
start = tokens[i].offset;
|
|
} else if ( bias < 0 && depth === 0 ) {
|
|
// Top-level closing - use offset as end
|
|
markers[markers.length] = $.extend(
|
|
{ context: context, start: start, end: tokens[i].offset },
|
|
$.wikiEditor.modules.templates.tpl.marker
|
|
);
|
|
}
|
|
if ( depth < 0 ) {
|
|
depth = 0;
|
|
}
|
|
}
|
|
}
|
|
},
|
|
exp: [
|
|
{ regex: /{{/, label: 'TEMPLATE_BEGIN' },
|
|
{ regex: /}}/, label: 'TEMPLATE_END', markAfter: true }
|
|
],
|
|
/**
|
|
* Internally used functions
|
|
*/
|
|
fn: {
|
|
/**
|
|
* @param context
|
|
* @param config
|
|
*/
|
|
create: function () {
|
|
// Do some stuff here...
|
|
}
|
|
}
|
|
|
|
}; } ) ( jQuery );
|