mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/WikiEditor
synced 2024-09-24 10:50:17 +00:00
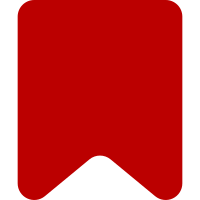
Deprecated as of MediaWiki 1.26, replaced with an empty array. Change-Id: Ic5f308060f796b896f98de34e153bb8bd11f8e6c
174 lines
4.8 KiB
JavaScript
174 lines
4.8 KiB
JavaScript
/* Preview module for wikiEditor */
|
|
( function ( $, mw ) {
|
|
/*jshint onevar:false */
|
|
$.wikiEditor.modules.preview = {
|
|
|
|
/**
|
|
* Compatability map
|
|
*/
|
|
browsers: {
|
|
// Left-to-right languages
|
|
ltr: {
|
|
msie: [['>=', 7]],
|
|
firefox: [['>=', 3]],
|
|
opera: [['>=', 9.6]],
|
|
safari: [['>=', 4]]
|
|
},
|
|
// Right-to-left languages
|
|
rtl: {
|
|
msie: [['>=', 8]],
|
|
firefox: [['>=', 3]],
|
|
opera: [['>=', 9.6]],
|
|
safari: [['>=', 4]]
|
|
}
|
|
},
|
|
|
|
/**
|
|
* Internally used functions
|
|
*/
|
|
fn: {
|
|
/**
|
|
* Creates a preview module within a wikiEditor
|
|
* @param context Context object of editor to create module in
|
|
* @param config Configuration object to create module from
|
|
*/
|
|
create: function ( context ) {
|
|
var api = new mw.Api();
|
|
|
|
if ( 'initialized' in context.modules.preview ) {
|
|
return;
|
|
}
|
|
context.modules.preview = {
|
|
'initialized': true,
|
|
'previewText': null,
|
|
'changesText': null
|
|
};
|
|
context.modules.preview.$preview = context.fn.addView( {
|
|
'name': 'preview',
|
|
'titleMsg': 'wikieditor-preview-tab',
|
|
'init': function ( context ) {
|
|
// Gets the latest copy of the wikitext
|
|
var wikitext = context.$textarea.textSelection( 'getContents' );
|
|
// Aborts when nothing has changed since the last preview
|
|
if ( context.modules.preview.previewText === wikitext ) {
|
|
return;
|
|
}
|
|
context.modules.preview.$preview.find( '.wikiEditor-preview-contents' ).empty();
|
|
context.modules.preview.$preview.find( '.wikiEditor-preview-loading' ).show();
|
|
api.post( {
|
|
action: 'parse',
|
|
title: mw.config.get( 'wgPageName' ),
|
|
text: wikitext,
|
|
prop: 'text|modules',
|
|
pst: ''
|
|
} ).done( function ( data ) {
|
|
if ( !data.parse || !data.parse.text || data.parse.text['*'] === undefined ) {
|
|
return;
|
|
}
|
|
|
|
context.modules.preview.previewText = wikitext;
|
|
context.modules.preview.$preview.find( '.wikiEditor-preview-loading' ).hide();
|
|
context.modules.preview.$preview.find( '.wikiEditor-preview-contents' )
|
|
.html( data.parse.text['*'] )
|
|
.append( '<div class="visualClear"></div>' )
|
|
.find( 'a:not([href^=#])' )
|
|
.click( false );
|
|
|
|
var loadmodules = data.parse.modules.concat(
|
|
data.parse.modulescripts,
|
|
data.parse.modulestyles
|
|
);
|
|
mw.loader.load( loadmodules );
|
|
} );
|
|
}
|
|
} );
|
|
|
|
context.$changesTab = context.fn.addView( {
|
|
'name': 'changes',
|
|
'titleMsg': 'wikieditor-preview-changes-tab',
|
|
'init': function ( context ) {
|
|
// Gets the latest copy of the wikitext
|
|
var wikitext = context.$textarea.textSelection( 'getContents' );
|
|
// Aborts when nothing has changed since the last time
|
|
if ( context.modules.preview.changesText === wikitext ) {
|
|
return;
|
|
}
|
|
context.$changesTab.find( 'table.diff tbody' ).empty();
|
|
context.$changesTab.find( '.wikiEditor-preview-loading' ).show();
|
|
|
|
// Call the API. First PST the input, then diff it
|
|
api.post( {
|
|
action: 'parse',
|
|
title: mw.config.get( 'wgPageName' ),
|
|
onlypst: '',
|
|
text: wikitext
|
|
} ).done( function ( data ) {
|
|
try {
|
|
var postdata2 = {
|
|
action: 'query',
|
|
indexpageids: '',
|
|
prop: 'revisions',
|
|
titles: mw.config.get( 'wgPageName' ),
|
|
rvdifftotext: data.parse.text['*'],
|
|
rvprop: ''
|
|
};
|
|
var section = $( '[name="wpSection"]' ).val();
|
|
if ( section !== '' ) {
|
|
postdata2.rvsection = section;
|
|
}
|
|
|
|
api.post( postdata2 )
|
|
.done( function ( data ) {
|
|
// Add diff CSS
|
|
mw.loader.load( 'mediawiki.action.history.diff' );
|
|
try {
|
|
var diff = data.query.pages[data.query.pageids[0]]
|
|
.revisions[0].diff['*'];
|
|
|
|
context.$changesTab.find( 'table.diff tbody' )
|
|
.html( diff )
|
|
.append( '<div class="visualClear"></div>' );
|
|
context.modules.preview.changesText = wikitext;
|
|
} catch ( e ) {
|
|
// "data.blah is undefined" error, ignore
|
|
}
|
|
context.$changesTab.find( '.wikiEditor-preview-loading' ).hide();
|
|
} );
|
|
} catch ( e ) {
|
|
// "data.blah is undefined" error, ignore
|
|
}
|
|
} );
|
|
}
|
|
} );
|
|
|
|
var loadingMsg = mw.msg( 'wikieditor-preview-loading' );
|
|
context.modules.preview.$preview
|
|
.add( context.$changesTab )
|
|
.append( $( '<div>' )
|
|
.addClass( 'wikiEditor-preview-loading' )
|
|
.append( $( '<img>' )
|
|
.addClass( 'wikiEditor-preview-spinner' )
|
|
.attr( {
|
|
'src': $.wikiEditor.imgPath + 'dialogs/loading.gif',
|
|
'valign': 'absmiddle',
|
|
'alt': loadingMsg,
|
|
'title': loadingMsg
|
|
} )
|
|
)
|
|
.append(
|
|
$( '<span>' ).text( loadingMsg )
|
|
)
|
|
)
|
|
.append( $( '<div>' )
|
|
.addClass( 'wikiEditor-preview-contents' )
|
|
);
|
|
context.$changesTab.find( '.wikiEditor-preview-contents' )
|
|
.html( '<table class="diff"><col class="diff-marker"/><col class="diff-content"/>' +
|
|
'<col class="diff-marker"/><col class="diff-content"/><tbody/></table>' );
|
|
}
|
|
}
|
|
|
|
};
|
|
|
|
}( jQuery, mediaWiki ) );
|