mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
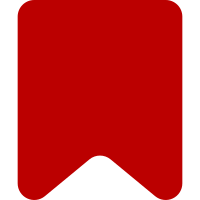
In this commit several methods (child node add/remove and parent/root modification) were also moved to ve.dm.BranchNode ve.dm.Node respectively. ve.Node and ve.BranchNode are immutable. ve.dm.Node and ve.dm.BranchNode are mutable. Other subclasses of ve.Node and ve.BranchNode should implement functionality to mimic changes made to a data model. Change-Id: Ia9ff78764f8f50f99fc8f9f9593657c0a0bf287e
67 lines
1.5 KiB
JavaScript
67 lines
1.5 KiB
JavaScript
/**
|
|
* Creates an ve.Node object.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
* @constructor
|
|
* @extends {ve.EventEmitter}
|
|
* @param {String} type Symbolic name of node type
|
|
*/
|
|
ve.Node = function( type ) {
|
|
// Inheritance
|
|
ve.EventEmitter.call( this );
|
|
|
|
// Properties
|
|
this.type = type;
|
|
this.parent = null;
|
|
this.root = this;
|
|
|
|
// Convenience function for emitting update events
|
|
// Has this bound to the closure scope, so can be passed as a callback
|
|
var _this = this;
|
|
this.emitUpdate = function() {
|
|
_this.emit( 'update' );
|
|
};
|
|
};
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Checks if this node can have child nodes.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @returns {Boolean} Whether this node can have children
|
|
*/
|
|
ve.Node.prototype.canHaveChildren = function() {
|
|
throw 've.Node.canHaveChildren not implemented in this subclass:' + this.constructor;
|
|
};
|
|
|
|
/**
|
|
* Checks if this node can have child nodes that can have child nodes.
|
|
*
|
|
* If this function returns false, only nodes that can't have children can be attached to this node.
|
|
* If canHaveChildren() returns false, this method must also return false.
|
|
*
|
|
* @method
|
|
* @abstract
|
|
* @returns {Boolean} Whether this node can have grandchildren
|
|
*/
|
|
ve.Node.prototype.canHaveGrandchildren = function() {
|
|
throw 've.Node.canHaveGrandchildren not implemented in this subclass:' + this.constructor;
|
|
};
|
|
|
|
/**
|
|
* Gets the symbolic node type name.
|
|
*
|
|
* @method
|
|
* @returns {String} Symbolic name of element type
|
|
*/
|
|
ve.Node.prototype.getType = function() {
|
|
return this.type;
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.extendClass( ve.Node, ve.EventEmitter );
|