mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
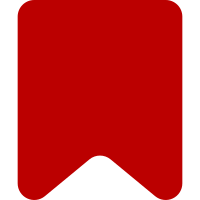
This is really only about the methods name, but doesn't change any behavior. I realized we work with several different definitions of what "empty" means. There are at least two significant definitions: 1. When a parameter's value is the empty string or identical to the default value, the behavior of the template is the same. It will use the default value just as if the user entered it. The auto-value is a meaningful value in this scenario and can't be considered equal to the empty string. 2. The context here is when the user presses the back button. This will destroy all user input. But an auto-value is not user input. It will appear again when the user realizes they made a mistake. Nothing is lost. Personally, I would not use the word "empty" to describe this concept. Things like "containsUserProvidedValue", "isCustomValue", "isMeaningfulValue", … come to mind. These are all still a big vague. A "user provided" value can be identical to the default or auto-value. "Custom" how? I went for "containsValuableData" for now. Bug: T274551 Change-Id: I2912a35556795c867a6b2396cbad291e947f0ed6
97 lines
2.6 KiB
JavaScript
97 lines
2.6 KiB
JavaScript
/*!
|
|
* VisualEditor DataModel MWTransclusionPartModel class.
|
|
*
|
|
* @copyright 2011-2020 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Abstract base class for items in a {@see ve.dm.MWTransclusionModel}. Holds a back-reference to
|
|
* it's parent. Currently used for:
|
|
* - {@see ve.dm.MWTemplateModel} for a single template invocation.
|
|
* - {@see ve.dm.MWTemplatePlaceholderModel} while searching for a template name to be added.
|
|
* - {@see ve.dm.MWTransclusionContentModel} for a raw wikitext snippet.
|
|
*
|
|
* @class
|
|
* @mixins OO.EventEmitter
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.MWTransclusionModel} transclusion
|
|
*/
|
|
ve.dm.MWTransclusionPartModel = function VeDmMWTransclusionPartModel( transclusion ) {
|
|
// Mixin constructors
|
|
OO.EventEmitter.call( this );
|
|
|
|
// Properties
|
|
this.transclusion = transclusion;
|
|
this.id = 'part_' + this.transclusion.getUniquePartId();
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.mixinClass( ve.dm.MWTransclusionPartModel, OO.EventEmitter );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* Emitted when anything changed in the content the part represents, e.g. a parameter was added to a
|
|
* template, or a value edited.
|
|
*
|
|
* @event change
|
|
*/
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Get transclusion part is in.
|
|
*
|
|
* @return {ve.dm.MWTransclusionModel} Transclusion
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.getTransclusion = function () {
|
|
return this.transclusion;
|
|
};
|
|
|
|
/**
|
|
* Get a unique part ID within the transclusion.
|
|
*
|
|
* @return {string} Unique ID
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.getId = function () {
|
|
return this.id;
|
|
};
|
|
|
|
/**
|
|
* Remove part from transclusion.
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.remove = function () {
|
|
this.transclusion.removePart( this );
|
|
};
|
|
|
|
/**
|
|
* Create a serialized representation of this part. Contains all information needed to recreate the
|
|
* original wikitext, including extra whitespace. Used in
|
|
* {@see ve.dm.MWTransclusionModel.getPlainObject}. The corresponding deserializer is in
|
|
* {@see ve.dm.MWTransclusionNode.static.getWikitext}.
|
|
*
|
|
* @return {Object|string|undefined} Serialized representation, raw wikitext, or undefined if empty
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.serialize = function () {
|
|
return undefined;
|
|
};
|
|
|
|
/**
|
|
* Add all non-existing required and suggested parameters, if any.
|
|
*
|
|
* @return {number} Number of parameters added
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.addPromptedParameters = function () {
|
|
return 0;
|
|
};
|
|
|
|
/**
|
|
* @return {boolean} True if there is meaningful user input that was not e.g. auto-generated
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.containsValuableData = function () {
|
|
return false;
|
|
};
|