mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-12 09:09:25 +00:00
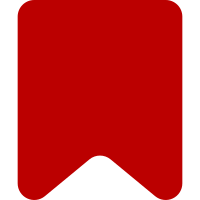
ve.ui.Inspector * Removed disabled state and interfaces - this isn't needed * Renamed prepareSelection to onInitialize * Using event emitter to run onInitialize, onOpen and onClose methods * Left removal up to the child class to handle in the onClose method * Replaced calls on context to close inspector to calling close directly * Renamed prepareSelection stub to onInitialize * Emitting initialize event from within the open method * Added recursion guarding to close method * Changed the close method's argument to be remove instead of accept - the more common case is to save changes, and the only time you wouldn't save changes is if you were to remove the annotation * Moved focus restore to close method ve.ui.Context * Moved the majority of the code in openInspector and closeInspector to event handlers for onInspectorOpen and onInspectorClose * Updated calls to closeInspector re: accept->remove argument change ve.ui.LinkInspector * Renamed prepareSelection to onInitialize and rewrote logic and documentation * Removed unused onLocationInputChange method * Moved restore focus (now it's in the inspector base class) ve.dm.SurfaceFragment * Added word mode for expandRange ve.dm.Surface * Added locking/unlocking while processing transactions - this was not an issue before because this was effectively being done manually throughout ce (which needs to be cleaned up) but once we started using the content action to insert content dm and ce started playing off each other and inserting in a loop - we already do this for undo/redo so it makes sense to do it here as well ve.InspectorAction * Updated arguments re: close method's accept->remove argument change Change-Id: I38995d4101fda71bfb2e6fe516603507ce820937
215 lines
5.2 KiB
JavaScript
215 lines
5.2 KiB
JavaScript
/**
|
|
* VisualEditor user interface Inspector class.
|
|
*
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Creates an ve.ui.Inspector object.
|
|
*
|
|
* @class
|
|
* @constructor
|
|
* @extends {ve.EventEmitter}
|
|
* @param {ve.ui.Context} context
|
|
*/
|
|
ve.ui.Inspector = function VeUiInspector( context ) {
|
|
// Inheritance
|
|
ve.EventEmitter.call( this );
|
|
|
|
// Properties
|
|
this.context = context;
|
|
this.initialSelection = null;
|
|
this.closing = false;
|
|
this.frame = context.getFrame();
|
|
this.$ = $( '<div class="ve-ui-inspector"></div>' );
|
|
this.$form = this.frame.$$( '<form></form>' );
|
|
this.$title = this.frame.$$( '<div class="ve-ui-inspector-title"></div>' )
|
|
.text( ve.msg( this.constructor.static.titleMessage ) );
|
|
this.$titleIcon = this.frame.$$( '<div class="ve-ui-inspector-titleIcon"></div>' )
|
|
.addClass( 've-ui-icon-' + this.constructor.static.icon );
|
|
this.$closeButton = this.frame.$$(
|
|
'<div class="ve-ui-inspector-button ve-ui-inspector-closeButton ve-ui-icon-close"></div>'
|
|
);
|
|
this.$removeButton = this.frame.$$(
|
|
'<div class="ve-ui-inspector-button ve-ui-inspector-removeButton ve-ui-icon-remove"></div>'
|
|
);
|
|
|
|
// Events
|
|
this.$closeButton.on( {
|
|
'click': ve.bind( this.onCloseButtonClick, this ),
|
|
} );
|
|
this.$removeButton.on( {
|
|
'click': ve.bind( this.onRemoveButtonClick, this ),
|
|
} );
|
|
this.$form.on( {
|
|
'submit': ve.bind( this.onFormSubmit, this ),
|
|
'keydown': ve.bind( this.onFormKeyDown, this )
|
|
} );
|
|
this.addListenerMethods( this, {
|
|
'initialize': 'onInitialize',
|
|
'open': 'onOpen',
|
|
'close': 'onClose'
|
|
} );
|
|
|
|
// Initialization
|
|
this.$.append(
|
|
this.$closeButton,
|
|
this.$titleIcon,
|
|
this.$title,
|
|
this.$removeButton,
|
|
this.$form
|
|
);
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.Inspector, ve.EventEmitter );
|
|
|
|
/* Static Members */
|
|
|
|
ve.ui.Inspector.static.icon = 'inspector';
|
|
|
|
ve.ui.Inspector.static.titleMessage = 'visualeditor-inspector-title';
|
|
|
|
ve.ui.Inspector.static.typePattern = new RegExp();
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Responds to close button click events.
|
|
*
|
|
* @method
|
|
* @param {jQuery.Event} e Click event
|
|
*/
|
|
ve.ui.Inspector.prototype.onCloseButtonClick = function () {
|
|
this.close();
|
|
};
|
|
|
|
/**
|
|
* Responds to remove button click events.
|
|
*
|
|
* @method
|
|
* @param {jQuery.Event} e Click event
|
|
* @emits 'remove'
|
|
*/
|
|
ve.ui.Inspector.prototype.onRemoveButtonClick = function() {
|
|
this.close( true );
|
|
};
|
|
|
|
/**
|
|
* Responds to form submission events.
|
|
*
|
|
* @method
|
|
* @param {jQuery.Event} e Submit event
|
|
*/
|
|
ve.ui.Inspector.prototype.onFormSubmit = function ( e ) {
|
|
this.close();
|
|
e.preventDefault();
|
|
return false;
|
|
};
|
|
|
|
/**
|
|
* Responds to form keydown events.
|
|
*
|
|
* @method
|
|
* @param {jQuery.Event} e Keydown event
|
|
*/
|
|
ve.ui.Inspector.prototype.onFormKeyDown = function ( e ) {
|
|
// Escape
|
|
if ( e.which === 27 ) {
|
|
this.close();
|
|
e.preventDefault();
|
|
return false;
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Responds to the inspector being initialized.
|
|
*
|
|
* This gives an inspector an opportunity to make selection and annotation changes prior to the
|
|
* inspector being opened.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Inspector.prototype.onInitialize = function () {
|
|
// This is a stub, override functionality in child classes
|
|
};
|
|
|
|
/**
|
|
* Responds to the inspector being opened.
|
|
*
|
|
* This is when an inspector would initialize it's form with data from the selection.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Inspector.prototype.onOpen = function () {
|
|
// This is a stub, override functionality in child classes
|
|
};
|
|
|
|
/**
|
|
* Responds to the inspector being closed.
|
|
*
|
|
* This is when an inspector would apply any changes made in the form to the selection.
|
|
*
|
|
* @method
|
|
* @param {Boolean} accept Changes to the form should be applied
|
|
*/
|
|
ve.ui.Inspector.prototype.onClose = function () {
|
|
// This is a stub, override functionality in child classes
|
|
};
|
|
|
|
/**
|
|
* Responds to the annotation being inspected being removed.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.Inspector.prototype.onRemove = function () {
|
|
// This is a stub, override functionality in child classes
|
|
};
|
|
|
|
/**
|
|
* Gets a list of matching annotations in selection.
|
|
*
|
|
* @method
|
|
* @param {ve.dm.SurfaceFragment} fragment Fragment to get matching annotations within
|
|
* @returns {ve.AnnotationSet} Matching annotations
|
|
*/
|
|
ve.ui.Inspector.prototype.getMatchingAnnotations = function ( fragment ) {
|
|
return fragment.getAnnotations().getAnnotationsByName( this.constructor.static.typePattern );
|
|
};
|
|
|
|
/**
|
|
* Opens inspector.
|
|
*
|
|
* @method
|
|
* @emits 'initialize'
|
|
* @emits 'open'
|
|
*/
|
|
ve.ui.Inspector.prototype.open = function () {
|
|
this.$.show();
|
|
this.emit( 'initialize' );
|
|
this.initialSelection = this.context.getSurface().getModel().getSelection();
|
|
this.emit( 'open' );
|
|
};
|
|
|
|
/**
|
|
* Closes inspector.
|
|
*
|
|
* This method guards against recursive calling internally. Recursion on this method is caused by
|
|
* changes to the document occuring in a close handler which in turn produce document model change
|
|
* events, which in turn cause the context to close the inspector again, and so on.
|
|
*
|
|
* @method
|
|
* @emits 'close' (remove)
|
|
*/
|
|
ve.ui.Inspector.prototype.close = function ( remove ) {
|
|
if ( !this.closing ) {
|
|
this.closing = true;
|
|
this.$.hide();
|
|
this.emit( 'close', remove );
|
|
this.context.getSurface().getView().getDocument().getDocumentNode().$.focus();
|
|
this.closing = false;
|
|
}
|
|
};
|