mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-12-04 18:58:37 +00:00
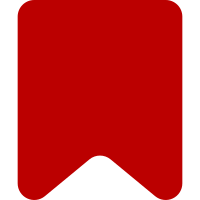
For readability. The current implementation is a sequence of 7 (!) array_…() function calls. It is also not free from bugs. If one of the two inputs (ExtensionRegistry and Config) specifies namespaces by e.g. canonical name, but the other by number, the two are not properly merged. It should be possible to use configuration to disable a namespace that would otherwise be enabled. This currently works only if both use the same array keys. Bug: T291727 Change-Id: I2671f391cdc510da21eda8a1dc5ed4d2513a378a
68 lines
1.7 KiB
PHP
68 lines
1.7 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\VisualEditor\Tests;
|
|
|
|
use ApiVisualEditor;
|
|
use ExtensionRegistry;
|
|
use HashConfig;
|
|
use MediaWikiIntegrationTestCase;
|
|
use Wikimedia\ScopedCallback;
|
|
|
|
/**
|
|
* @covers \ApiVisualEditor
|
|
*/
|
|
class ApiVisualEditorTest extends MediaWikiIntegrationTestCase {
|
|
|
|
/** @var ScopedCallback|null */
|
|
private $scopedCallback;
|
|
|
|
protected function setUp(): void {
|
|
parent::setUp();
|
|
$this->scopedCallback = ExtensionRegistry::getInstance()->setAttributeForTest(
|
|
'VisualEditorAvailableNamespaces',
|
|
[ 'User' => true, 'Template_Talk' => true ]
|
|
);
|
|
}
|
|
|
|
protected function tearDown(): void {
|
|
$this->scopedCallback = null;
|
|
parent::tearDown();
|
|
}
|
|
|
|
public function testIsAllowedNamespace() {
|
|
$config = new HashConfig( [ 'VisualEditorAvailableNamespaces' => [
|
|
0 => true,
|
|
1 => false,
|
|
] ] );
|
|
$this->assertTrue( ApiVisualEditor::isAllowedNamespace( $config, 0 ) );
|
|
$this->assertFalse( ApiVisualEditor::isAllowedNamespace( $config, 1 ) );
|
|
}
|
|
|
|
public function testGetAvailableNamespaceIds() {
|
|
$config = new HashConfig( [ 'VisualEditorAvailableNamespaces' => [
|
|
0 => true,
|
|
1 => false,
|
|
-1 => true,
|
|
999999 => true,
|
|
2 => false,
|
|
'Template' => true,
|
|
'Foobar' => true,
|
|
] ] );
|
|
$this->assertSame(
|
|
[ -1, 0, 10, 11 ],
|
|
ApiVisualEditor::getAvailableNamespaceIds( $config )
|
|
);
|
|
}
|
|
|
|
public function testIsAllowedContentType() {
|
|
$config = new HashConfig( [ 'VisualEditorAvailableContentModels' => [
|
|
'on' => true,
|
|
'off' => false,
|
|
] ] );
|
|
$this->assertTrue( ApiVisualEditor::isAllowedContentType( $config, 'on' ) );
|
|
$this->assertFalse( ApiVisualEditor::isAllowedContentType( $config, 'off' ) );
|
|
$this->assertFalse( ApiVisualEditor::isAllowedContentType( $config, 'unknown' ) );
|
|
}
|
|
|
|
}
|