mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-16 10:59:56 +00:00
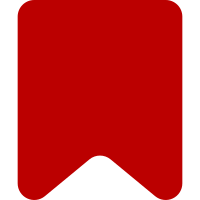
This is really only about the methods name, but doesn't change any behavior. I realized we work with several different definitions of what "empty" means. There are at least two significant definitions: 1. When a parameter's value is the empty string or identical to the default value, the behavior of the template is the same. It will use the default value just as if the user entered it. The auto-value is a meaningful value in this scenario and can't be considered equal to the empty string. 2. The context here is when the user presses the back button. This will destroy all user input. But an auto-value is not user input. It will appear again when the user realizes they made a mistake. Nothing is lost. Personally, I would not use the word "empty" to describe this concept. Things like "containsUserProvidedValue", "isCustomValue", "isMeaningfulValue", … come to mind. These are all still a big vague. A "user provided" value can be identical to the default or auto-value. "Custom" how? I went for "containsValuableData" for now. Bug: T274551 Change-Id: I2912a35556795c867a6b2396cbad291e947f0ed6
64 lines
1.4 KiB
JavaScript
64 lines
1.4 KiB
JavaScript
/*!
|
|
* VisualEditor DataModel MWTransclusionContentModel class.
|
|
*
|
|
* @copyright 2011-2020 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Represents a raw wikitext snippet that is part of an unbalanced sequence of template invocations.
|
|
* Meant to be an item in a {@see ve.dm.MWTransclusionModel}. Holds a back-reference to it's parent.
|
|
*
|
|
* @class
|
|
* @extends ve.dm.MWTransclusionPartModel
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.MWTransclusionModel} transclusion
|
|
* @param {string} [wikitext='']
|
|
*/
|
|
ve.dm.MWTransclusionContentModel = function VeDmMWTransclusionContentModel( transclusion, wikitext ) {
|
|
// Parent constructor
|
|
ve.dm.MWTransclusionContentModel.super.call( this, transclusion );
|
|
|
|
// Properties
|
|
this.wikitext = wikitext || '';
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.dm.MWTransclusionContentModel, ve.dm.MWTransclusionPartModel );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* Emitted when the wikitext changed.
|
|
*
|
|
* @event change
|
|
*/
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* @param {string} wikitext
|
|
*/
|
|
ve.dm.MWTransclusionContentModel.prototype.setWikitext = function ( wikitext ) {
|
|
if ( this.wikitext !== wikitext ) {
|
|
this.wikitext = wikitext;
|
|
this.emit( 'change' );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.dm.MWTransclusionContentModel.prototype.serialize = function () {
|
|
return this.wikitext;
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.dm.MWTransclusionContentModel.prototype.containsValuableData = function () {
|
|
return this.wikitext !== '';
|
|
};
|