mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-25 19:26:46 +00:00
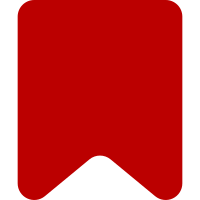
* Replaces c8b4a28936
* Use Object() casting to detect objects instead of .constructor
(or instanceof). Both .constructor and instanceof compare by reference
the type "Object" which means if the object comes from another window
(where there is a different "Object" and "Object.prototype") it will
drop out of the system and go freewack.
Theory: If a variable casted to an object returns true when strictly compared
to the original, the input must be an object.
Which is true. It doesn't change the inheritance, it doesn't make it inherit
from this window's Object if the object is from another window's object. All it
does is cast to an object if not an object already.
So e.g. "Object(5) !== 5" because 5 is a primitive value as opposed to an instance
of Number.
And contrary to "typeof", it doesn't return true for "null".
* .constructor also has the problem that it only works this way if the
input is a plain object. e.g. a simple construtor function that creates
an object also get in the wrong side of the if/else case since it is
an instance of Object, but not directly (rather indirectly via another
constructor).
* Added unit tests for basic getHash usage, as well as regression tests
against the above two mentioned problems (these tests fail before this commit).
* While at it, also improved other utilities a bit.
- Use hasOwnProperty instead of casting to boolean
when checking for presence of native support.
Thanks to Douglas Crockford for that tip.
- Fix documentation for ve.getHash: Parameter is not named "obj".
- Add Object-check to ve.getObjectKeys per ES5 Object.keys spec (to match native behavior)
- Add Object-check to ve.getObjectValues to match ve.getObjectKeys
- Improved performance of ve.getObjectKeys shim. Tried several potential optimizations
and compared with jsperf. Using a "static" reference to hasOwn improves performance
(by not having to look it up 4 scopes up and 3 property levels deep).
Also using [.length] instead of .push() shared off a few ms.
- Added unit tests for ve.getObjectValues
Change-Id: If24d09405321f201c67f7df75d332bb1171c8a36
98 lines
2.7 KiB
PHP
98 lines
2.7 KiB
PHP
<?php
|
|
/**
|
|
* VisualEditor extension hooks
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @copyright 2011-2012 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
class VisualEditorHooks {
|
|
/** List of skins VisualEditor integration supports */
|
|
protected static $supportedSkins = array( 'vector', 'apex', 'monobook' );
|
|
|
|
/**
|
|
* Adds VisualEditor JS to the output if in the correct namespace.
|
|
*
|
|
* This is attached to the MediaWiki 'BeforePageDisplay' hook.
|
|
*
|
|
* @param $output OutputPage
|
|
* @param $skin Skin
|
|
*/
|
|
public static function onBeforePageDisplay( &$output, &$skin ) {
|
|
global $wgTitle;
|
|
if (
|
|
in_array( $skin->getSkinName(), self::$supportedSkins ) &&
|
|
(
|
|
// Article in the VisualEditor namespace
|
|
$wgTitle->getNamespace() === NS_VISUALEDITOR ||
|
|
// Special page action for an article in the VisualEditor namespace
|
|
$skin->getRelevantTitle()->getNamespace() === NS_VISUALEDITOR
|
|
)
|
|
) {
|
|
$output->addModules( array( 'ext.visualEditor.viewPageTarget' ) );
|
|
}
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Adds extra variables to the page config.
|
|
*
|
|
* This is attached to the MediaWiki 'MakeGlobalVariablesScript' hook.
|
|
*/
|
|
public static function onMakeGlobalVariablesScript( &$vars ) {
|
|
global $wgUser, $wgTitle;
|
|
$vars['wgVisualEditor'] = array(
|
|
'isPageWatched' => $wgUser->isWatched( $wgTitle )
|
|
);
|
|
return true;
|
|
}
|
|
|
|
public static function onResourceLoaderTestModules( array &$testModules, ResourceLoader &$resourceLoader ) {
|
|
$testModules['qunit']['ext.visualEditor.test'] = array(
|
|
'scripts' => array(
|
|
// QUnit plugin
|
|
've.qunit.js',
|
|
// VisualEditor Tests
|
|
've.test.js',
|
|
've.example.js',
|
|
've.Document.test.js',
|
|
've.Node.test.js',
|
|
've.BranchNode.test.js',
|
|
've.LeafNode.test.js',
|
|
've.Factory.test.js',
|
|
// VisualEditor DataModel Tests
|
|
'dm/ve.dm.example.js',
|
|
'dm/ve.dm.NodeFactory.test.js',
|
|
'dm/ve.dm.Node.test.js',
|
|
'dm/ve.dm.Converter.test.js',
|
|
'dm/ve.dm.BranchNode.test.js',
|
|
'dm/ve.dm.LeafNode.test.js',
|
|
'dm/nodes/ve.dm.TextNode.test.js',
|
|
'dm/ve.dm.Document.test.js',
|
|
'dm/ve.dm.DocumentSynchronizer.test.js',
|
|
'dm/ve.dm.Transaction.test.js',
|
|
'dm/ve.dm.TransactionProcessor.test.js',
|
|
'dm/ve.dm.Surface.test.js',
|
|
// VisualEditor ContentEditable Tests
|
|
'ce/ve.ce.test.js',
|
|
'ce/ve.ce.Document.test.js',
|
|
'ce/ve.ce.NodeFactory.test.js',
|
|
'ce/ve.ce.Node.test.js',
|
|
'ce/ve.ce.BranchNode.test.js',
|
|
'ce/ve.ce.LeafNode.test.js',
|
|
'ce/nodes/ve.ce.TextNode.test.js',
|
|
),
|
|
'dependencies' => array(
|
|
'ext.visualEditor.core',
|
|
'ext.visualEditor.viewPageTarget',
|
|
),
|
|
'localBasePath' => dirname( __FILE__ ) . '/modules/ve/test',
|
|
'remoteExtPath' => 'VisualEditor/modules/ve/test',
|
|
);
|
|
|
|
return true;
|
|
}
|
|
}
|