mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 10:35:48 +00:00
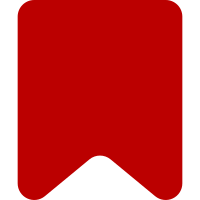
There was a remaining issue when the window was made very narrow in desktop mode (smaller than 500px). This patch doesn't aim to really "fix" the dialog's design in this case. The goal is to make the popup window appear less broken, so the text can stil be read and the buttons clicked. That's all. This patch should not have any effect in: a) mobile mode, b) desktop mode when the window is wider than 500px. Bug: T294839 Change-Id: I3171dbb991533b91eaadba63b78d0ff40aa486dc
71 lines
1.8 KiB
JavaScript
71 lines
1.8 KiB
JavaScript
/*!
|
|
* VisualEditor user interface MWFloatingHelpDialog class.
|
|
*
|
|
* @copyright 2011-2021 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Dialog for the floating help element.
|
|
*
|
|
* @class
|
|
* @extends OO.ui.ProcessDialog
|
|
*
|
|
* @constructor
|
|
* @param {Object} [config] Configuration options
|
|
* @cfg {string} label
|
|
* @cfg {jQuery} $message
|
|
*/
|
|
ve.ui.MWFloatingHelpDialog = function VeUiMWFloatingHelpDialog( config ) {
|
|
// Parent constructor
|
|
ve.ui.MWFloatingHelpDialog.super.call( this, config );
|
|
|
|
this.label = config.label;
|
|
this.$message = config.$message;
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWFloatingHelpDialog, OO.ui.ProcessDialog );
|
|
|
|
/* Static properties */
|
|
|
|
ve.ui.MWFloatingHelpDialog.static.name = 'floatingHelp';
|
|
|
|
ve.ui.MWFloatingHelpDialog.static.actions = [
|
|
{
|
|
label: OO.ui.deferMsg( 'visualeditor-dialog-action-cancel' ),
|
|
flags: [ 'safe', 'close' ]
|
|
}
|
|
];
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWFloatingHelpDialog.prototype.initialize = function () {
|
|
ve.ui.MWFloatingHelpDialog.super.prototype.initialize.call( this );
|
|
var content = new OO.ui.PanelLayout( { padded: true, expanded: false } );
|
|
content.$element.append( this.$message );
|
|
this.$body.append( content.$element );
|
|
this.$foot.remove();
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWFloatingHelpDialog.prototype.getSetupProcess = function ( data ) {
|
|
return ve.ui.MWFloatingHelpDialog.super.prototype.getSetupProcess.call( this, data ).next( function () {
|
|
this.title.setLabel( this.label );
|
|
}, this );
|
|
};
|
|
|
|
ve.ui.MWFloatingHelpDialog.prototype.getSizeProperties = function () {
|
|
var sizeProps = ve.ui.MWFloatingHelpDialog.super.prototype.getSizeProperties.call( this );
|
|
if ( !OO.ui.isMobile() ) {
|
|
return ve.extendObject( {}, sizeProps, { width: '350px', maxHeight: '50%' } );
|
|
}
|
|
return sizeProps;
|
|
};
|