mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-12-02 18:06:16 +00:00
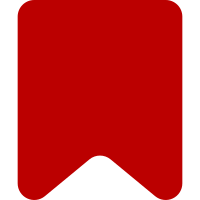
This is needed because the $params array is then passed on to ApiEditPage, so if the hook implementer wants to alter the data used with the edit, it needs to be able to modify the $params. See I494d72a42d9103c28c4d44077cfe0f1269fc7b00 for an example where GrowthExperiments would like to modify the 'tags' parameter for an edit. Depends-On: Idd052281898f99e4f13f241d5633294b59b29329 Bug: T304747 Change-Id: Ia4842a1593028f5fa145de167ccf9b72efa81351
65 lines
1.6 KiB
PHP
65 lines
1.6 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\VisualEditor\Tests;
|
|
|
|
use MediaWiki\Extension\VisualEditor\VisualEditorHookRunner;
|
|
use MediaWiki\HookContainer\HookContainer;
|
|
use MediaWiki\Page\PageIdentityValue;
|
|
use MediaWiki\User\UserIdentityValue;
|
|
use MediaWikiUnitTestCase;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\VisualEditor\VisualEditorHookRunner
|
|
*/
|
|
class VisualEditorHookRunnerTest extends MediaWikiUnitTestCase {
|
|
|
|
public function testPreSaveHook() {
|
|
$container = $this->createNoOpMock( HookContainer::class, [ 'run' ] );
|
|
$container->expects( $this->once() )
|
|
->method( 'run' )
|
|
->with(
|
|
'VisualEditorApiVisualEditorEditPreSave',
|
|
$this->isType( 'array' ),
|
|
[ 'abortable' => true ]
|
|
)
|
|
->willReturn( true );
|
|
$runner = new VisualEditorHookRunner( $container );
|
|
|
|
$apiResponse = [];
|
|
$params = [];
|
|
$result = $runner->onVisualEditorApiVisualEditorEditPreSave(
|
|
PageIdentityValue::localIdentity( 0, 0, 'test' ),
|
|
UserIdentityValue::newAnonymous( '' ),
|
|
'',
|
|
$params,
|
|
[],
|
|
$apiResponse
|
|
);
|
|
$this->assertTrue( $result );
|
|
}
|
|
|
|
public function testPostSaveHook() {
|
|
$container = $this->createNoOpMock( HookContainer::class, [ 'run' ] );
|
|
$container->expects( $this->once() )
|
|
->method( 'run' )
|
|
->with(
|
|
'VisualEditorApiVisualEditorEditPostSave',
|
|
$this->isType( 'array' ),
|
|
[ 'abortable' => false ]
|
|
);
|
|
$runner = new VisualEditorHookRunner( $container );
|
|
|
|
$apiResponse = [];
|
|
$runner->onVisualEditorApiVisualEditorEditPostSave(
|
|
PageIdentityValue::localIdentity( 0, 0, 'test' ),
|
|
UserIdentityValue::newAnonymous( '' ),
|
|
'',
|
|
[],
|
|
[],
|
|
[],
|
|
$apiResponse
|
|
);
|
|
}
|
|
|
|
}
|