mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
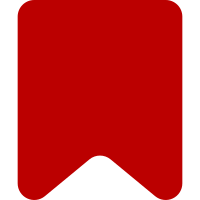
* Use TitleInputWidget for wiki-page-name and wiki-template-name parameters * Use UserInputWidget for wiki-user-name parameters * Use a custom hacky CheckboxInputWidget child class for boolean parameters * Borrow some ve.ui.MWExternalLinkAnnotationWidget.prototype.createInputWidget code for url parameters * Use a TextInputWidget with multiline disabled for line parameters Not dealt with in this commit, so fallback to existing behaviour: * string * number * unknown * content * unbalanced-wikitext * date * wiki-file-name Bug: T55613 Bug: T124734 Bug: T124736 Change-Id: If04944d64303d959e8dd605e75a175895932b788 Depends-On: I87699a93ca1b34c6d248456fcc060f584623d158 Depends-On: I5e97604f0fc24176d5e89899bf0505dc442a1a7e
73 lines
1.8 KiB
JavaScript
73 lines
1.8 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface MWExternalLinkAnnotationWidget class.
|
|
*
|
|
* @copyright 2011-2016 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Creates an ve.ui.MWExternalLinkAnnotationWidget object.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.LinkAnnotationWidget
|
|
*
|
|
* @constructor
|
|
* @param {Object} [config] Configuration options
|
|
*/
|
|
ve.ui.MWExternalLinkAnnotationWidget = function VeUiMWExternalLinkAnnotationWidget() {
|
|
// Parent constructor
|
|
ve.ui.MWExternalLinkAnnotationWidget.super.apply( this, arguments );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWExternalLinkAnnotationWidget, ve.ui.LinkAnnotationWidget );
|
|
|
|
/* Static Methods */
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWExternalLinkAnnotationWidget.static.getAnnotationFromText = function ( value ) {
|
|
var href = value.trim();
|
|
|
|
// Keep annotation in sync with value
|
|
if ( href === '' ) {
|
|
return null;
|
|
} else {
|
|
return new ve.dm.MWExternalLinkAnnotation( {
|
|
type: 'link/mwExternal',
|
|
attributes: {
|
|
href: href
|
|
}
|
|
} );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Create an external link input widget.
|
|
*
|
|
* @param {Object} [config] Configuration options
|
|
* @return {OO.ui.TextInputWidget} Text input widget
|
|
*/
|
|
ve.ui.MWExternalLinkAnnotationWidget.static.createExternalLinkInputWidget = function ( config ) {
|
|
return new OO.ui.TextInputWidget( $.extend( {}, config, {
|
|
icon: 'linkExternal',
|
|
validate: function ( text ) {
|
|
return !!ve.init.platform.getExternalLinkUrlProtocolsRegExp().exec( text.trim() );
|
|
}
|
|
} ) );
|
|
};
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Create a text input widget to be used by the annotation widget
|
|
*
|
|
* @param {Object} [config] Configuration options
|
|
* @return {OO.ui.TextInputWidget} Text input widget
|
|
*/
|
|
ve.ui.MWExternalLinkAnnotationWidget.prototype.createInputWidget = function ( config ) {
|
|
return this.constructor.static.createExternalLinkInputWidget( config );
|
|
};
|