mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-16 10:59:56 +00:00
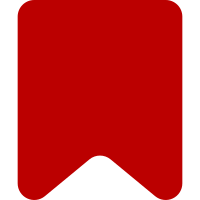
The idea is to possibly rename some of these classes, based on these descriptions. But this should be done in later, separate patches. Change-Id: I7f9e5b2382711b434d6dd618489fa3ed8b7a46b4
100 lines
2.3 KiB
JavaScript
100 lines
2.3 KiB
JavaScript
/*!
|
|
* VisualEditor DataModel MWTransclusionPartModel class.
|
|
*
|
|
* @copyright 2011-2020 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Abstract base class for items in a {@see ve.dm.MWTransclusionModel}. Holds a back-reference to
|
|
* it's parent. Currently used for:
|
|
* - {@see ve.dm.MWTemplateModel} for a single template invocation.
|
|
* - {@see ve.dm.MWTemplatePlaceholderModel} while searching for a template name to be added.
|
|
* - {@see ve.dm.MWTransclusionContentModel} for a raw wikitext snippet.
|
|
*
|
|
* @class
|
|
* @mixins OO.EventEmitter
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.MWTransclusionModel} transclusion Transclusion
|
|
*/
|
|
ve.dm.MWTransclusionPartModel = function VeDmMWTransclusionPartModel( transclusion ) {
|
|
// Mixin constructors
|
|
OO.EventEmitter.call( this );
|
|
|
|
// Properties
|
|
this.transclusion = transclusion;
|
|
this.id = 'part_' + this.transclusion.getUniquePartId();
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.mixinClass( ve.dm.MWTransclusionPartModel, OO.EventEmitter );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* @event change
|
|
*/
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Get transclusion part is in.
|
|
*
|
|
* @return {ve.dm.MWTransclusionModel} Transclusion
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.getTransclusion = function () {
|
|
return this.transclusion;
|
|
};
|
|
|
|
/**
|
|
* Get a unique part ID within the transclusion.
|
|
*
|
|
* @return {string} Unique ID
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.getId = function () {
|
|
return this.id;
|
|
};
|
|
|
|
/**
|
|
* Remove part from transclusion.
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.remove = function () {
|
|
this.transclusion.removePart( this );
|
|
};
|
|
|
|
/**
|
|
* Get serialized representation of transclusion part.
|
|
*
|
|
* @return {Mixed} Serialized representation, or undefined if empty
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.serialize = function () {
|
|
return undefined;
|
|
};
|
|
|
|
/**
|
|
* Get the wikitext for this part.
|
|
*
|
|
* @return {string} Wikitext
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.getWikitext = function () {
|
|
return '';
|
|
};
|
|
|
|
/**
|
|
* Add all non-existing required and suggested parameters, if any.
|
|
*
|
|
* @return {number} Number of parameters added
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.addPromptedParameters = function () {
|
|
return 0;
|
|
};
|
|
|
|
/**
|
|
* @return {boolean} True if there is no user input
|
|
*/
|
|
ve.dm.MWTransclusionPartModel.prototype.isEmpty = function () {
|
|
return true;
|
|
};
|