mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-26 19:56:49 +00:00
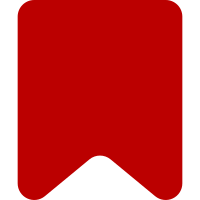
This patch adds ve.track; it provides a means for VisualEditor code to log various changes of state that are of potential analytic interest. This is done without coupling VisualEditor to a particular analytics framework by providing a method, ve.hook.registerHandler, by which event data may be routed to a particular analytic framework for processing and dispatch. ve.track uses a $.Callbacks-like object for tracking analytic events which can remember an arbitrary number of past events. This is done by maintaining an array containing the arguments of past calls and maintaining a counter for each callback indicating its position in the queue (i.e., how many events it has already received.) This ensures handlers are called for each event, including events which were fired before the handler was registered. This allows the load-order of VE and analytics components to remain unspecified. Change-Id: I29740fa7a0ac403e484e0acee6dfcadaf6fc4566
49 lines
1.6 KiB
JavaScript
49 lines
1.6 KiB
JavaScript
/*!
|
|
* VisualEditor tracking methods.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
( function () {
|
|
var callbacks = $.Callbacks( 'memory' ), queue = [];
|
|
|
|
/**
|
|
* Track an analytic event.
|
|
*
|
|
* VisualEditor uses this method internally to track internal changes of state that are of analytic
|
|
* interest, either because they provide data about how users interact with the editor, or because
|
|
* they contain exception info, latency measurements, or other metrics that help gauge performance
|
|
* and reliability. VisualEditor does not transmit these events by default, but it provides a
|
|
* generic interface for routing these events to an analytics framework.
|
|
*
|
|
* @member ve
|
|
* @param {string} name Event name
|
|
* @param {Mixed...} [data] Data to log
|
|
*/
|
|
ve.track = function () {
|
|
queue.push( { context: { timeStamp: ve.now() }, args: arguments } );
|
|
callbacks.fire( queue );
|
|
};
|
|
|
|
/**
|
|
* Register a handler for analytic events.
|
|
*
|
|
* Handlers will be called once for each tracked event, including any events that fired before the
|
|
* handler was registered; 'this' is set to a plain object with a 'timeStamp' property indicating
|
|
* the exact time at which the event fired.
|
|
*
|
|
* @member ve
|
|
* @param {Function} callback
|
|
*/
|
|
ve.trackRegisterHandler = function ( callback ) {
|
|
var invocation, seen = 0;
|
|
callbacks.add( function ( queue ) {
|
|
for ( ; seen < queue.length; seen++ ) {
|
|
invocation = queue[ seen ];
|
|
callback.apply( invocation.context, invocation.args );
|
|
}
|
|
} );
|
|
};
|
|
}() );
|