mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
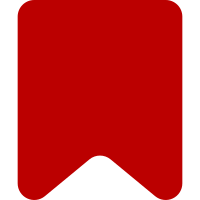
The behavior is now consistant with what would happen when the buttons are triggered. Instead of emitting a button click I directly wired the methods that will by triggerd by the click. This might make it easier to remove the old sidbar later. To avoid movement when the buttons should be disabled, an additional check was added to the onMove method. It's not identical to the more complex check in the outlineControlsWidget, but should be enough for our use case. The onDelete method already just does nothing if nothing is selected. Bug: T300971 Change-Id: I8a278c9657c91fd648944b5a8c1204c9fff75b7e
95 lines
2.9 KiB
JavaScript
95 lines
2.9 KiB
JavaScript
/**
|
|
* Generic button-like widget for items in the template dialog sidebar. See
|
|
* {@see OO.ui.ButtonWidget} for inspiration.
|
|
*
|
|
* @class
|
|
* @extends OO.ui.OptionWidget
|
|
*
|
|
* @constructor
|
|
* @param {Object} config
|
|
* @cfg {string} [icon=''] Symbolic name of an icon, e.g. "puzzle" or "wikiText"
|
|
* @cfg {string} label
|
|
*/
|
|
ve.ui.MWTransclusionOutlineButtonWidget = function VeUiMWTransclusionOutlineButtonWidget( config ) {
|
|
// Parent constructor
|
|
ve.ui.MWTransclusionOutlineButtonWidget.super.call( this, ve.extendObject( config, {
|
|
classes: [ 've-ui-mwTransclusionOutlineButtonWidget' ]
|
|
} ) );
|
|
|
|
// Mixin constructors
|
|
OO.ui.mixin.ButtonElement.call( this, {
|
|
// FIXME semantically this could be a <legend> and the surrounding OutlinePartWidget a <fieldset>
|
|
$button: $( '<span>' ),
|
|
framed: false
|
|
} );
|
|
OO.ui.mixin.IconElement.call( this, config );
|
|
OO.ui.mixin.TabIndexedElement.call( this, ve.extendObject( {
|
|
$tabIndexed: this.$button
|
|
}, config ) );
|
|
ve.ui.MWAriaDescribe.call( this, {
|
|
ariaLabel: config.label,
|
|
$describedElement: this.$button
|
|
} );
|
|
|
|
// FIXME hack for screen readers to understand the selection state
|
|
this.$button.attr( {
|
|
role: 'gridcell',
|
|
'aria-selected': 'false'
|
|
} );
|
|
|
|
this.$element
|
|
.append( this.$button.append( this.$icon, this.$label ) );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWTransclusionOutlineButtonWidget, OO.ui.OptionWidget );
|
|
OO.mixinClass( ve.ui.MWTransclusionOutlineButtonWidget, OO.ui.mixin.ButtonElement );
|
|
OO.mixinClass( ve.ui.MWTransclusionOutlineButtonWidget, OO.ui.mixin.IconElement );
|
|
OO.mixinClass( ve.ui.MWTransclusionOutlineButtonWidget, OO.ui.mixin.TabIndexedElement );
|
|
OO.mixinClass( ve.ui.MWTransclusionOutlineButtonWidget, ve.ui.MWAriaDescribe );
|
|
|
|
ve.ui.MWTransclusionOutlineButtonWidget.static.highlightable = false;
|
|
ve.ui.MWTransclusionOutlineButtonWidget.static.pressable = false;
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* @event keyPressed
|
|
* @param {number} key Typically one of the {@see OO.ui.Keys} constants
|
|
*/
|
|
|
|
/**
|
|
* @inheritDoc OO.ui.mixin.ButtonElement
|
|
* @param {jQuery.Event} e
|
|
* @fires keyPressed
|
|
*/
|
|
ve.ui.MWTransclusionOutlineButtonWidget.prototype.onKeyDown = function ( e ) {
|
|
var isMac = ve.getSystemPlatform() === 'mac';
|
|
var withMetaKey = isMac ? e.metaKey : e.ctrlKey;
|
|
|
|
if ( e.which === OO.ui.Keys.SPACE &&
|
|
!withMetaKey && !e.shiftKey && !e.altKey
|
|
) {
|
|
// We know we can only select another part, so don't even try to unselect this one
|
|
if ( !this.isSelected() ) {
|
|
this.emit( 'keyPressed', e.which );
|
|
}
|
|
// The default behavior of pressing space is to scroll down
|
|
e.preventDefault();
|
|
return;
|
|
}
|
|
|
|
return OO.ui.mixin.ButtonElement.prototype.onKeyDown.call( this, e );
|
|
};
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
ve.ui.MWTransclusionOutlineButtonWidget.prototype.setSelected = function ( state ) {
|
|
if ( this.$button ) {
|
|
this.$button.attr( 'aria-selected', state.toString() );
|
|
}
|
|
return ve.ui.MWTransclusionOutlineButtonWidget.super.prototype.setSelected.call( this, state );
|
|
};
|