mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
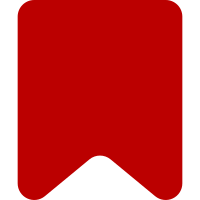
Technically the old BookletLayout sidebar is still there. But it's never visible, effectively dead, and meant to be removed. This patch just empties the OutlineItems of all template dialog related pages without actually disabling the old sidebar. Note this partly reverts Ie57f462. Bug: T310859 Bug: T310866 Change-Id: Ic0b7d703f369045ed342426563f8eeb3e47046db
192 lines
5.8 KiB
JavaScript
192 lines
5.8 KiB
JavaScript
/*!
|
|
* VisualEditor user interface MWTemplatePlaceholderPage class.
|
|
*
|
|
* @copyright 2011-2020 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* MediaWiki transclusion dialog placeholder page.
|
|
*
|
|
* @class
|
|
* @extends OO.ui.PageLayout
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.MWTemplatePlaceholderModel} placeholder Template placeholder
|
|
* @param {string} name Unique symbolic name of page
|
|
* @param {Object} [config] Configuration options
|
|
* @cfg {jQuery} [$overlay] Overlay to render dropdowns in
|
|
*/
|
|
ve.ui.MWTemplatePlaceholderPage = function VeUiMWTemplatePlaceholderPage( placeholder, name, config ) {
|
|
var veConfig = mw.config.get( 'wgVisualEditorConfig' );
|
|
|
|
// Configuration initialization
|
|
config = ve.extendObject( {
|
|
scrollable: false
|
|
}, config );
|
|
|
|
// Parent constructor
|
|
ve.ui.MWTemplatePlaceholderPage.super.call( this, name, config );
|
|
|
|
// Properties
|
|
this.placeholder = placeholder;
|
|
|
|
this.addTemplateInput = new ve.ui.MWTemplateTitleInputWidget( {
|
|
$overlay: config.$overlay,
|
|
showDescriptions: true,
|
|
api: ve.init.target.getContentApi()
|
|
} )
|
|
.connect( this, {
|
|
change: 'onTemplateInputChange',
|
|
enter: 'onAddTemplate'
|
|
} );
|
|
|
|
this.addTemplateInput.getLookupMenu().connect( this, {
|
|
choose: 'onAddTemplate'
|
|
} );
|
|
|
|
this.addTemplateInput.$input.attr( 'aria-label', ve.msg( 'visualeditor-dialog-transclusion-add-template' ) );
|
|
|
|
this.addTemplateButton = new OO.ui.ButtonWidget( {
|
|
label: ve.msg( 'visualeditor-dialog-transclusion-add-template' ),
|
|
flags: [ 'progressive' ],
|
|
classes: [ 've-ui-mwTransclusionDialog-addButton' ],
|
|
disabled: true
|
|
} )
|
|
.connect( this, { click: 'onAddTemplate' } );
|
|
|
|
var addTemplateActionFieldLayout = new OO.ui.ActionFieldLayout(
|
|
this.addTemplateInput,
|
|
this.addTemplateButton,
|
|
{ align: 'top' }
|
|
);
|
|
|
|
var addTemplateFieldsetConfig = {
|
|
label: ve.msg( 'visualeditor-dialog-transclusion-placeholder' ),
|
|
icon: 'puzzle',
|
|
classes: [ 've-ui-mwTransclusionDialog-addTemplateFieldset' ],
|
|
items: [ addTemplateActionFieldLayout ]
|
|
};
|
|
|
|
var dialogTitle = this.placeholder.getTransclusion().isSingleTemplate() ?
|
|
'visualeditor-dialog-transclusion-template-search' :
|
|
'visualeditor-dialog-transclusion-add-template';
|
|
|
|
// TODO: Remove `mw.storage.remove` after a few months, let's say December 2022.
|
|
mw.storage.remove( 'mwe-visualeditor-hide-visualeditor-dialog-transclusion-feedback-message' );
|
|
|
|
addTemplateFieldsetConfig = ve.extendObject( addTemplateFieldsetConfig, {
|
|
// The following messages are used here:
|
|
// * visualeditor-dialog-transclusion-template-search
|
|
// * visualeditor-dialog-transclusion-add-template
|
|
label: ve.msg( dialogTitle ),
|
|
help: ve.msg( 'visualeditor-dialog-transclusion-template-search-help' ),
|
|
helpInline: true
|
|
} );
|
|
this.addTemplateFieldset = new OO.ui.FieldsetLayout( addTemplateFieldsetConfig );
|
|
|
|
// Initialization
|
|
this.$element
|
|
.addClass( 've-ui-mwTemplatePlaceholderPage' )
|
|
.append( this.addTemplateFieldset.$element );
|
|
|
|
if ( !veConfig.transclusionDialogNewSidebar ) {
|
|
this.removeButton = new OO.ui.ButtonWidget( {
|
|
framed: false,
|
|
icon: 'trash',
|
|
title: ve.msg( 'visualeditor-dialog-transclusion-remove-template' ),
|
|
flags: [ 'destructive' ],
|
|
classes: [ 've-ui-mwTransclusionDialog-removeButton' ]
|
|
} )
|
|
.connect( this, { click: 'onRemoveButtonClick' } );
|
|
|
|
if ( this.placeholder.getTransclusion().isSingleTemplate() ) {
|
|
this.removeButton.toggle( false );
|
|
}
|
|
this.$element.append( this.removeButton.$element );
|
|
}
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWTemplatePlaceholderPage, OO.ui.PageLayout );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWTemplatePlaceholderPage.prototype.setupOutlineItem = function () {
|
|
this.outlineItem
|
|
// Basic properties to make the OO.ui.OutlineControlsWidget buttons behave sane
|
|
.setMovable( true )
|
|
.setRemovable( true );
|
|
};
|
|
|
|
/**
|
|
* @inheritDoc OO.ui.PanelLayout
|
|
*/
|
|
ve.ui.MWTemplatePlaceholderPage.prototype.focus = function () {
|
|
// The parent method would focus the first element, which might be the message widget
|
|
this.addTemplateInput.focus();
|
|
|
|
// HACK: Set the width of the lookupMenu to the width of the input
|
|
// TODO: This should be handled upstream in OOUI
|
|
this.addTemplateInput.lookupMenu.width = this.addTemplateInput.$input[ 0 ].clientWidth;
|
|
};
|
|
|
|
/**
|
|
* @private
|
|
*/
|
|
ve.ui.MWTemplatePlaceholderPage.prototype.onAddTemplate = function () {
|
|
var transclusion = this.placeholder.getTransclusion(),
|
|
menu = this.addTemplateInput.getLookupMenu();
|
|
|
|
if ( menu.isVisible() ) {
|
|
menu.chooseItem( menu.findSelectedItem() );
|
|
}
|
|
var name = this.addTemplateInput.getMWTitle();
|
|
if ( !name ) {
|
|
// Invalid titles return null, so abort here.
|
|
return;
|
|
}
|
|
|
|
// TODO tracking will only be implemented temporarily to answer questions on
|
|
// template usage for the Technical Wishes topic area see T258917
|
|
var event = {
|
|
action: 'add-template',
|
|
// eslint-disable-next-line camelcase
|
|
template_names: [ name.getPrefixedText() ]
|
|
};
|
|
var editCountBucket = mw.config.get( 'wgUserEditCountBucket' );
|
|
if ( editCountBucket !== null ) {
|
|
// eslint-disable-next-line camelcase
|
|
event.user_edit_count_bucket = editCountBucket;
|
|
}
|
|
mw.track( 'event.VisualEditorTemplateDialogUse', event );
|
|
|
|
var part = ve.dm.MWTemplateModel.newFromName( transclusion, name );
|
|
transclusion.replacePart( this.placeholder, part );
|
|
this.addTemplateInput.pushPending();
|
|
// abort pending lookups, also, so the menu can't appear after we've left the page
|
|
this.addTemplateInput.closeLookupMenu();
|
|
this.addTemplateButton.setDisabled( true );
|
|
if ( this.removeButton ) {
|
|
this.removeButton.setDisabled( true );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* @private
|
|
*/
|
|
ve.ui.MWTemplatePlaceholderPage.prototype.onTemplateInputChange = function () {
|
|
this.addTemplateButton.setDisabled( this.addTemplateInput.getMWTitle() === null );
|
|
};
|
|
|
|
/**
|
|
* @private
|
|
*/
|
|
ve.ui.MWTemplatePlaceholderPage.prototype.onRemoveButtonClick = function () {
|
|
this.placeholder.remove();
|
|
};
|