mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-05 22:22:54 +00:00
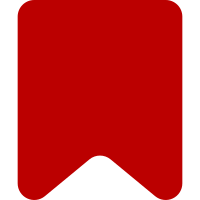
Since format dropdown tool is the only tool where we actually lose focus to the documentNode, we must restore focus so that firefox and chrome will display a cursor after converting content branches. Bug: 50338 Change-Id: I4059b2688565570e0efc21078035775b7aed49e1
82 lines
2.3 KiB
JavaScript
82 lines
2.3 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface FormatAction class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Format action.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.Action
|
|
* @constructor
|
|
* @param {ve.ui.Surface} surface Surface to act on
|
|
*/
|
|
ve.ui.FormatAction = function VeUiFormatAction( surface ) {
|
|
// Parent constructor
|
|
ve.ui.Action.call( this, surface );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.FormatAction, ve.ui.Action );
|
|
|
|
/* Static Properties */
|
|
|
|
/**
|
|
* List of allowed methods for this action.
|
|
*
|
|
* @static
|
|
* @property
|
|
*/
|
|
ve.ui.FormatAction.static.methods = ['convert'];
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Convert the format of content.
|
|
*
|
|
* Conversion splits and unwraps all lists and replaces content branch nodes.
|
|
*
|
|
* TODO: Refactor functionality into {ve.dm.SurfaceFragment}.
|
|
*
|
|
* @param {string} type
|
|
* @param {Object} attributes
|
|
*/
|
|
ve.ui.FormatAction.prototype.convert = function ( type, attributes ) {
|
|
var selected, i, length, contentBranch, txs,
|
|
surfaceModel = this.surface.getModel(),
|
|
selection = surfaceModel.getSelection(),
|
|
fragmentForSelection = surfaceModel.getFragment( selection, true ),
|
|
doc = surfaceModel.getDocument(),
|
|
fragments = [];
|
|
|
|
// We can't have headings or pre's in a list, so if we're trying to convert
|
|
// things that are in lists to a heading or a pre, split the list
|
|
selected = doc.selectNodes( selection, 'leaves' );
|
|
for ( i = 0, length = selected.length; i < length; i++ ) {
|
|
contentBranch = selected[i].node.isContent() ?
|
|
selected[i].node.getParent() :
|
|
selected[i].node;
|
|
|
|
fragments.push( surfaceModel.getFragment( contentBranch.getOuterRange(), true ) );
|
|
}
|
|
|
|
for ( i = 0, length = fragments.length; i < length; i++ ) {
|
|
fragments[i].isolateAndUnwrap( type );
|
|
}
|
|
selection = fragmentForSelection.getRange();
|
|
|
|
// Since format dropdown tool is a focusable menu, documentNode has lost focus.
|
|
// Restore focus to documentNode so that firefox will display the cursor after conversion.
|
|
this.surface.view.documentView.documentNode.$[0].focus();
|
|
|
|
txs = ve.dm.Transaction.newFromContentBranchConversion( doc, selection, type, attributes );
|
|
surfaceModel.change( txs, selection );
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.ui.actionFactory.register( 'format', ve.ui.FormatAction );
|