mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-05 22:22:54 +00:00
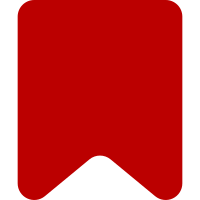
Objectives: * Split ve.Surface into ve.Editor and ve.ui.Surface * Move actions, triggers and commands to ve.ui * Move toolbar wrapping, floating, shadow and actions functionality to configurable options of ve.ui.Toolbar * Make ve.ce.Surface and ve.ui.Surface inherit ve.Element and use this.$$ for iframe friendliness * Make the toolbar separately initialized so it's possible to have a surface without one, as well as control where the toolbar is Some change notes: VisualEditor.php * Added standalone module for mediawiki integrated unit testing ve.ce.Surface.js * Remove requirement to pass in an attached container to construct object * Inherit ve.Element and use this.$$ instead of $ * Make getSelectionRect iframe friendly * Move most of the initialize stuff to a new initialize method to be called after the surface is attached to the DOM ve.init.mw.ViewPageTarget.js * Merge toolbar functions into setup/teardown methods * Add toolbar manually (since it's not added by the surface anymore) ve.init.sa.Target.js * Update new init procedure for editor, surface and toolbar separately * Move toolbar floating stuff to ve.Toolbar Change-Id: If91a9d6e76a8be8d1b5a2566394765a37d29a8a7
111 lines
3 KiB
JavaScript
111 lines
3 KiB
JavaScript
/*!
|
|
* VisualEditor UserInterface AnnotationAction class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Annotation action.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.Action
|
|
* @constructor
|
|
* @param {ve.ui.Surface} surface Surface to act on
|
|
*/
|
|
ve.ui.AnnotationAction = function VeUiAnnotationAction( surface ) {
|
|
// Parent constructor
|
|
ve.ui.Action.call( this, surface );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.AnnotationAction, ve.ui.Action );
|
|
|
|
/* Static Properties */
|
|
|
|
/**
|
|
* List of allowed methods for the action.
|
|
*
|
|
* @static
|
|
* @property
|
|
*/
|
|
ve.ui.AnnotationAction.static.methods = ['set', 'clear', 'toggle', 'clearAll'];
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Set an annotation.
|
|
*
|
|
* @method
|
|
* @param {string} name Annotation name, for example: 'textStyle/bold'
|
|
* @param {Object} [data] Additional annotation data
|
|
*/
|
|
ve.ui.AnnotationAction.prototype.set = function ( name, data ) {
|
|
this.surface.getModel().getFragment().annotateContent( 'set', name, data );
|
|
};
|
|
|
|
/**
|
|
* Clear an annotation.
|
|
*
|
|
* @method
|
|
* @param {string} name Annotation name, for example: 'textStyle/bold'
|
|
* @param {Object} [data] Additional annotation data
|
|
*/
|
|
ve.ui.AnnotationAction.prototype.clear = function ( name, data ) {
|
|
this.surface.getModel().getFragment().annotateContent( 'clear', name, data );
|
|
};
|
|
|
|
/**
|
|
* Toggle an annotation.
|
|
*
|
|
* If the selected text is completely covered with the annotation already the annotation will be
|
|
* cleared. Otherwise the annotation will be set.
|
|
*
|
|
* @method
|
|
* @param {string} name Annotation name, for example: 'textStyle/bold'
|
|
* @param {Object} [data] Additional annotation data
|
|
*/
|
|
ve.ui.AnnotationAction.prototype.toggle = function ( name, data ) {
|
|
var existingAnnotations,
|
|
surfaceModel = this.surface.getModel(),
|
|
fragment = surfaceModel.getFragment(),
|
|
annotation = ve.dm.annotationFactory.create( name, data );
|
|
|
|
if ( !fragment.getRange().isCollapsed() ) {
|
|
fragment.annotateContent(
|
|
fragment.getAnnotations().containsComparable( annotation ) ? 'clear' : 'set', name, data
|
|
);
|
|
} else {
|
|
existingAnnotations = surfaceModel
|
|
.getInsertionAnnotations().getComparableAnnotations( annotation );
|
|
if ( existingAnnotations.isEmpty() ) {
|
|
surfaceModel.addInsertionAnnotations( annotation );
|
|
} else {
|
|
surfaceModel.removeInsertionAnnotations( existingAnnotations );
|
|
}
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Clear all annotations.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.AnnotationAction.prototype.clearAll = function () {
|
|
var i, len, arr,
|
|
fragment = this.surface.getModel().getFragment(),
|
|
annotations = fragment.getAnnotations( true );
|
|
|
|
arr = annotations.get();
|
|
// TODO: Allow multiple annotations to be set or cleared by ve.dm.SurfaceFragment, probably
|
|
// using an annotation set and ideally building a single transaction
|
|
for ( i = 0, len = arr.length; i < len; i++ ) {
|
|
fragment.annotateContent( 'clear', arr[i].name, arr[i].data );
|
|
}
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.ui.actionFactory.register( 'annotation', ve.ui.AnnotationAction );
|