mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-27 20:26:46 +00:00
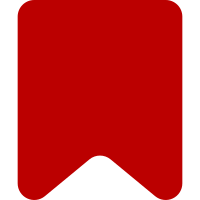
The bubbling update events are not needed with ce or dm, but were once upon a time useful for es, this just eliminates some unused cruft that was costing extra function call overhead. Change-Id: Ia16d0f4cd74c84cded5caecada33ee83d0882f30
87 lines
2.3 KiB
JavaScript
87 lines
2.3 KiB
JavaScript
/**
|
|
* ContentEditable node that can have branch or leaf children.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
* @constructor
|
|
* @extends {ve.BranchNode}
|
|
* @extends {ve.ce.Node}
|
|
* @param model {ve.dm.BranchNode} Model to observe
|
|
* @param {jQuery} [$element] Element to use as a container
|
|
*/
|
|
ve.ce.BranchNode = function( model, $element ) {
|
|
// Inheritance
|
|
ve.BranchNode.call( this );
|
|
ve.ce.Node.call( this, model, $element );
|
|
|
|
// Events
|
|
this.model.addListenerMethod( this, 'splice', 'onSplice' );
|
|
|
|
// Initialization
|
|
if ( model.getChildren().length ) {
|
|
this.onSplice.apply( this, [0, 0].concat( model.getChildren() ) );
|
|
}
|
|
};
|
|
|
|
/* Methods */
|
|
|
|
ve.ce.BranchNode.prototype.replaceDomWrapper = function( $element ) {
|
|
// Copy classes
|
|
$element.attr( 'class', this.$.attr( 'class' ) );
|
|
// Move contents
|
|
$element.append( this.$.contents() );
|
|
// Swap elements
|
|
this.$.replaceWith( $element );
|
|
// Use new element from now on
|
|
this.$ = $element;
|
|
};
|
|
|
|
/**
|
|
* Responds to splice events on a ve.dm.BranchNode.
|
|
*
|
|
* ve.ce.Node objects are generated from the inserted ve.dm.Node objects, producing a view that's a
|
|
* mirror of it's model.
|
|
*
|
|
* @method
|
|
* @param {Integer} index Index to remove and or insert nodes at
|
|
* @param {Integer} howmany Number of nodes to remove
|
|
* @param {ve.dm.BranchNode} [...] Variadic list of nodes to insert
|
|
*/
|
|
ve.ce.BranchNode.prototype.onSplice = function( index, howmany ) {
|
|
var i,
|
|
length,
|
|
args = Array.prototype.slice.call( arguments, 0 );
|
|
// Convert models to views and attach them to this node
|
|
if ( args.length >= 3 ) {
|
|
for ( i = 2, length = args.length; i < length; i++ ) {
|
|
args[i] = ve.ce.factory.createNode( args[i].getType(), args[i] );
|
|
}
|
|
}
|
|
var removals = this.children.splice.apply( this.children, args );
|
|
for ( i = 0, length = removals.length; i < length; i++ ) {
|
|
removals[i].detach();
|
|
// Update DOM
|
|
removals[i].$.detach();
|
|
}
|
|
if ( args.length >= 3 ) {
|
|
var $target;
|
|
if ( index ) {
|
|
// Get the element before the insertion point
|
|
$anchor = this.$.children().eq( index - 1 );
|
|
}
|
|
for ( i = args.length - 1; i >= 2; i-- ) {
|
|
args[i].attach( this );
|
|
if ( index ) {
|
|
$anchor.after( args[i].$ );
|
|
} else {
|
|
this.$.prepend( args[i].$ );
|
|
}
|
|
}
|
|
}
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.extendClass( ve.ce.BranchNode, ve.BranchNode );
|
|
ve.extendClass( ve.ce.BranchNode, ve.ce.Node );
|