mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
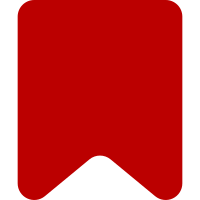
Changed: VisualEditor.i18n.php * Updated Link inspector i18n messages ve.ui.MetaDialog.js -> ve.ui.PagedDialog * Moved paging functionality into Paged dialog ve.ui.EditorPanelLayout -> ve.ui.PagePanelLayout.js * Renamed from EditorPanelLayout to work nicely with the concept of stacks and pages ve.ui.GroupElement.js * Added addItem method and change addItems to use it ve.ui.Dialog.css * Updated classname as per refactor of meta dialog ve.ui.StackPanelLayout.js * Set currentItem property on showItem * In addItems method, show currentItem with class method ** rather display block on element ve.ui.Layout.css * Make editorPanel layout 100% in width. ve.ui.Widget.css * Added CategoryWidget and CategoryPopup styles * Other adjustments ve.ui.PopupWidget.js * Added auto-close on loss of focus * Made friendly with being initialized inside a frame ve.ui.MWLinkTargetInputWidget.js * Mixin ve.ui.PendingInputWidget and remove pending methods * Prevent querying on spaces * Reintroduce i18n messages for menu sections ve.ui.MenuWidget.js * Update cases of $input config property to input New: ve.ui.PagedDialog.js * Refactored base-class for mwMeta dialog (and probably other dialogs too) * Abstracts adding and accessing pages ve.ui.PendingInputWidget.js * Moved pushPending and popPending methods into pending class Change-Id: I29bcd92b7b5641941a4e98e65b2a56424a5263ff
101 lines
2.7 KiB
JavaScript
101 lines
2.7 KiB
JavaScript
/*!
|
|
* VisualEditor user interface PagedDialog class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Paged dialog.
|
|
*
|
|
* A paged dialog has an outline in the left third, and a series of pages in the right two-thirds.
|
|
* Pages can be added using the #addPage method, and later accessed using `this.pages[name]` or
|
|
* through the #getPage method.
|
|
*
|
|
* @class
|
|
* @abstract
|
|
* @extends ve.ui.Dialog
|
|
*
|
|
* @constructor
|
|
* @param {ve.Surface} surface
|
|
*/
|
|
ve.ui.PagedDialog = function VeUiPagedDialog( surface ) {
|
|
// Parent constructor
|
|
ve.ui.Dialog.call( this, surface );
|
|
|
|
// Properties
|
|
this.pages = {};
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
ve.inheritClass( ve.ui.PagedDialog, ve.ui.Dialog );
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Handle frame ready events.
|
|
*
|
|
* @method
|
|
*/
|
|
ve.ui.PagedDialog.prototype.initialize = function () {
|
|
// Call parent method
|
|
ve.ui.Dialog.prototype.initialize.call( this );
|
|
|
|
// Properties
|
|
this.outlinePanel = new ve.ui.PanelLayout( { '$$': this.$$, 'scroll': true } );
|
|
this.pagesPanel = new ve.ui.StackPanelLayout( { '$$': this.$$ } );
|
|
this.layout = new ve.ui.GridLayout(
|
|
[this.outlinePanel, this.pagesPanel], { '$$': this.$$, 'widths': [1, 2] }
|
|
);
|
|
this.outlineWidget = new ve.ui.OutlineWidget( { '$$': this.$$ } );
|
|
|
|
// Events
|
|
this.outlineWidget.on( 'select', ve.bind( function ( item ) {
|
|
if ( item ) {
|
|
this.pagesPanel.showItem( this.pages[item.getData()] );
|
|
}
|
|
}, this ) );
|
|
|
|
// Initialization
|
|
this.outlinePanel.$.append( this.outlineWidget.$ ).addClass( 've-ui-pagedDialog-outlinePanel' );
|
|
this.pagesPanel.$.addClass( 've-ui-pagedDialog-pagesPanel' );
|
|
this.$body.append( this.layout.$ );
|
|
};
|
|
|
|
/**
|
|
* Add a page to the dialog.
|
|
*
|
|
* @method
|
|
* @param {string} name Symbolic name of page
|
|
* @param {jQuery|string} [label] Page label
|
|
* @param {string} [icon] Symbolic name of icon
|
|
* @chainable
|
|
*/
|
|
ve.ui.PagedDialog.prototype.addPage = function ( name, label, icon ) {
|
|
var config = { '$$': this.$$, 'icon': icon, 'label': label || name };
|
|
|
|
// Create and add page panel and outline item
|
|
this.pages[name] = new ve.ui.PagePanelLayout( config );
|
|
this.pagesPanel.addItems( [this.pages[name]] );
|
|
this.outlineWidget.addItems( [ new ve.ui.OutlineItemWidget( name, config ) ] );
|
|
|
|
// Auto-select first item when nothing is selected yet
|
|
if ( !this.outlineWidget.getSelectedItem() ) {
|
|
this.outlineWidget.selectItem( this.outlineWidget.getClosestSelectableItem( 0 ) );
|
|
}
|
|
|
|
return this;
|
|
};
|
|
|
|
/**
|
|
* Get a page by name.
|
|
*
|
|
* @method
|
|
* @param {string} name Symbolic name of page
|
|
* @returns {ve.ui.PagePanelLayout|undefined} Page, if found
|
|
*/
|
|
ve.ui.PagedDialog.prototype.getPage = function ( name ) {
|
|
return this.pages[name];
|
|
};
|