mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-26 07:15:32 +00:00
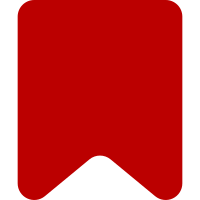
It does not provide any additional information, and it is long enough that it causes the actual template name(s) to not fit on the screen on small mobile sizes. Bug: T209610 Change-Id: I47a995905fef5aa2cabb2b3215111de0b506e7f7
105 lines
2.8 KiB
JavaScript
105 lines
2.8 KiB
JavaScript
/*!
|
|
* VisualEditor MWTransclusionContextItem class.
|
|
*
|
|
* @copyright 2011-2019 VisualEditor Team and others; see http://ve.mit-license.org
|
|
*/
|
|
|
|
/**
|
|
* Context item for a MWTransclusion.
|
|
*
|
|
* @class
|
|
* @extends ve.ui.LinearContextItem
|
|
*
|
|
* @constructor
|
|
* @param {ve.ui.Context} context Context item is in
|
|
* @param {ve.dm.Model} model Model item is related to
|
|
* @param {Object} config Configuration options
|
|
*/
|
|
ve.ui.MWTransclusionContextItem = function VeUiMWTransclusionContextItem() {
|
|
// Parent constructor
|
|
ve.ui.MWTransclusionContextItem.super.apply( this, arguments );
|
|
|
|
// Initialization
|
|
this.$element.addClass( 've-ui-mwTransclusionContextItem' );
|
|
if ( !this.model.isSingleTemplate() ) {
|
|
this.setLabel( ve.msg( 'visualeditor-dialogbutton-transclusion-tooltip' ) );
|
|
}
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ui.MWTransclusionContextItem, ve.ui.LinearContextItem );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.ui.MWTransclusionContextItem.static.name = 'transclusion';
|
|
|
|
ve.ui.MWTransclusionContextItem.static.icon = 'puzzle';
|
|
|
|
ve.ui.MWTransclusionContextItem.static.label =
|
|
OO.ui.deferMsg( 'visualeditor-dialogbutton-template-tooltip' );
|
|
|
|
ve.ui.MWTransclusionContextItem.static.modelClasses = [ ve.dm.MWTransclusionNode ];
|
|
|
|
ve.ui.MWTransclusionContextItem.static.commandName = 'transclusion';
|
|
|
|
/**
|
|
* Only display item for single-template transclusions of these templates.
|
|
*
|
|
* @property {string|string[]|null}
|
|
* @static
|
|
* @inheritable
|
|
*/
|
|
ve.ui.MWTransclusionContextItem.static.template = null;
|
|
|
|
/* Static Methods */
|
|
|
|
/**
|
|
* @static
|
|
* @localdoc Sharing implementation with ve.ui.MWTransclusionDialogTool
|
|
*/
|
|
ve.ui.MWTransclusionContextItem.static.isCompatibleWith =
|
|
ve.ui.MWTransclusionDialogTool.static.isCompatibleWith;
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWTransclusionContextItem.prototype.getDescription = function () {
|
|
return ve.msg(
|
|
'visualeditor-dialog-transclusion-contextitem-description',
|
|
ve.ce.MWTransclusionNode.static.getDescription( this.model ),
|
|
ve.ce.MWTransclusionNode.static.getTemplatePartDescriptions( this.model ).length
|
|
);
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.LinearContextItem.prototype.renderDescription = function () {
|
|
// No "Generated from" prefix in mobile context
|
|
this.$description.text( ve.ce.MWTransclusionNode.static.getDescription( this.model ) );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
ve.ui.MWTransclusionContextItem.prototype.onEditButtonClick = function () {
|
|
var surfaceModel = this.context.getSurface().getModel(),
|
|
selection = surfaceModel.getSelection();
|
|
|
|
if ( selection instanceof ve.dm.TableSelection ) {
|
|
surfaceModel.setLinearSelection( selection.getOuterRanges(
|
|
surfaceModel.getDocument()
|
|
)[ 0 ] );
|
|
}
|
|
|
|
// Parent method
|
|
ve.ui.MWTransclusionContextItem.super.prototype.onEditButtonClick.apply( this, arguments );
|
|
};
|
|
|
|
/* Registration */
|
|
|
|
ve.ui.contextItemFactory.register( ve.ui.MWTransclusionContextItem );
|