mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-23 18:28:51 +00:00
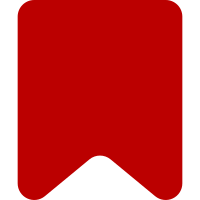
This is not great, but it's a start (and unblocks other pull-throughs). New changes: c401efc98 build: Replace jsduck with jsdoc for documentation 16ba162a0 JSDoc: @mixins -> @mixes 9e0a1f53b JSDoc: Fix complex return types 449b6cc0f Prefer arrow function callbacks 1539af2c8 Remove 'this' bindings in arrow functions b760f3b14 Use arrow functions in OO.ui.Process steps 57c24109e Use arrow functions in jQuery callbacks 9622ccef9 Convert some remaining functions callbacks to arrow functions f6c885021 Remove useless local variable 1cd800020 Clear branch node cache when rebuilding tree Bug: T250843 Bug: T363329 Change-Id: I0f4878ca84b95e3f388b358b943f105637e455f9
179 lines
4.2 KiB
JavaScript
179 lines
4.2 KiB
JavaScript
/*!
|
|
* Grunt file
|
|
*
|
|
* @package VisualEditor
|
|
*/
|
|
|
|
'use strict';
|
|
|
|
module.exports = function ( grunt ) {
|
|
const conf = grunt.file.readJSON( 'extension.json' ),
|
|
screenshotOptions = {
|
|
reporter: 'spec',
|
|
// TODO: Work out how to catch this timeout and continue.
|
|
// For now just make it very long.
|
|
timeout: 5 * 60 * 1000,
|
|
require: [
|
|
function () {
|
|
global.langs = [ grunt.option( 'lang' ) || 'en' ];
|
|
}
|
|
]
|
|
},
|
|
screenshotOptionsAll = {
|
|
reporter: 'spec',
|
|
// TODO: Work out how to catch this timeout and continue.
|
|
// For now just make it very long.
|
|
timeout: 5 * 60 * 1000,
|
|
require: [
|
|
function () {
|
|
global.langs = require( './build/tasks/screenshotLangs.json' ).langs;
|
|
}
|
|
]
|
|
};
|
|
|
|
grunt.loadNpmTasks( 'grunt-banana-checker' );
|
|
grunt.loadNpmTasks( 'grunt-contrib-watch' );
|
|
grunt.loadNpmTasks( 'grunt-eslint' );
|
|
grunt.loadNpmTasks( 'grunt-image' );
|
|
grunt.loadNpmTasks( 'grunt-mocha-test' );
|
|
grunt.loadNpmTasks( 'grunt-stylelint' );
|
|
grunt.loadNpmTasks( 'grunt-tyops' );
|
|
grunt.loadTasks( 'lib/ve/build/tasks' );
|
|
grunt.loadTasks( 'build/tasks' );
|
|
|
|
grunt.initConfig( {
|
|
mochaTest: {
|
|
'screenshots-en': {
|
|
options: screenshotOptions,
|
|
src: [ 'build/screenshots.userGuide.js' ]
|
|
},
|
|
'screenshots-all': {
|
|
options: screenshotOptionsAll,
|
|
src: [ 'build/screenshots.userGuide.js' ]
|
|
},
|
|
'diff-screenshots-en': {
|
|
options: screenshotOptions,
|
|
src: [ 'build/screenshots.diffs.js' ]
|
|
},
|
|
'diff-screenshots-all': {
|
|
options: screenshotOptionsAll,
|
|
src: [ 'build/screenshots.diffs.js' ]
|
|
}
|
|
},
|
|
image: {
|
|
pngs: {
|
|
options: {
|
|
zopflipng: true,
|
|
pngout: true,
|
|
optipng: true,
|
|
advpng: true,
|
|
pngcrush: true
|
|
},
|
|
'screenshots-en': {
|
|
expand: true,
|
|
src: 'screenshots/*-en.png'
|
|
},
|
|
'screenshots-all': {
|
|
expand: true,
|
|
src: 'screenshots/*.png'
|
|
}
|
|
},
|
|
svgs: {
|
|
options: {
|
|
svgo: [
|
|
'--pretty',
|
|
'--enable=removeRasterImages',
|
|
'--enable=sortAttrs',
|
|
'--disable=cleanupIDs',
|
|
'--disable=removeDesc',
|
|
'--disable=removeTitle',
|
|
'--disable=removeViewBox',
|
|
'--disable=removeXMLProcInst'
|
|
]
|
|
},
|
|
expand: true,
|
|
src: 'images/*.svg'
|
|
}
|
|
},
|
|
tyops: {
|
|
options: {
|
|
typos: 'build/typos.json'
|
|
},
|
|
src: [
|
|
'**/*.{js,json,less,css,txt,php,md,sh}',
|
|
'!package-lock.json',
|
|
'!build/typos.json',
|
|
'!**/i18n/**/*.json',
|
|
'**/i18n/**/en.json',
|
|
'**/i18n/**/qqq.json',
|
|
'!lib/**',
|
|
'!{docs,node_modules,vendor}/**',
|
|
'!.git/**'
|
|
]
|
|
},
|
|
eslint: {
|
|
options: {
|
|
cache: true,
|
|
fix: grunt.option( 'fix' )
|
|
},
|
|
all: [ '.' ]
|
|
},
|
|
stylelint: {
|
|
options: {
|
|
reportNeedlessDisables: true
|
|
},
|
|
all: [
|
|
'**/*.{css,less}',
|
|
'!coverage/**',
|
|
'!dist/**',
|
|
'!docs/**',
|
|
'!lib/**',
|
|
'!node_modules/**',
|
|
'!vendor/**'
|
|
]
|
|
},
|
|
banana: conf.MessagesDirs,
|
|
watch: {
|
|
files: [
|
|
'.{stylelintrc,eslintrc}.json',
|
|
'<%= eslint.all %>',
|
|
'<%= stylelint.all %>'
|
|
],
|
|
tasks: 'test'
|
|
}
|
|
} );
|
|
|
|
grunt.registerTask( 'git-status', function () {
|
|
const done = this.async();
|
|
// Are there unstaged changes?
|
|
require( 'child_process' ).exec( 'git ls-files --modified', function ( err, stdout, stderr ) {
|
|
const ret = err || stderr || stdout;
|
|
if ( ret ) {
|
|
grunt.log.error( 'Unstaged changes in these files:' );
|
|
grunt.log.error( ret );
|
|
// Show a condensed diff
|
|
require( 'child_process' ).exec( 'git diff -U1 | tail -n +3', function ( err2, stdout2, stderr2 ) {
|
|
grunt.log.write( err2 || stderr2 || stdout2 );
|
|
done( false );
|
|
} );
|
|
} else {
|
|
grunt.log.ok( 'No unstaged changes.' );
|
|
done();
|
|
}
|
|
} );
|
|
} );
|
|
|
|
grunt.registerTask( 'test', [ 'tyops', 'eslint', 'stylelint', 'banana' ] );
|
|
grunt.registerTask( 'test-ci', [ 'git-status' ] );
|
|
grunt.registerTask( 'screenshots', [ 'mochaTest:screenshots-en', 'image:pngs' ] );
|
|
grunt.registerTask( 'screenshots-all', [ 'mochaTest:screenshots-all', 'image:pngs' ] );
|
|
grunt.registerTask( 'default', 'test' );
|
|
|
|
if ( process.env.JENKINS_HOME ) {
|
|
grunt.renameTask( 'test', 'test-internal' );
|
|
grunt.registerTask( 'test', [ 'test-internal', 'test-ci' ] );
|
|
} else {
|
|
grunt.registerTask( 'ci', [ 'test', 'image:svgs', 'test-ci' ] );
|
|
}
|
|
};
|