mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-14 18:15:19 +00:00
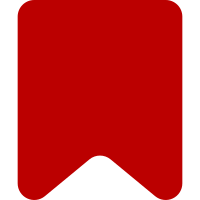
Pages titles with a wikipedia interwiki prefix now load the page from corresponding Wikipedia. Links in a page then stay within the given language. Note that Parsoid currently makes no effort to recognize localized namespaces, so it won't render media files, categories etc correctly. Change-Id: I7bc4102e81a402772ea23231170734d580ea15b9
80 lines
1.6 KiB
JavaScript
80 lines
1.6 KiB
JavaScript
function Title ( key, ns, nskey, env ) {
|
|
this.key = key;
|
|
// Namespace index
|
|
this.ns = new Namespace( ns );
|
|
// the original ns string
|
|
this.nskey = nskey;
|
|
this.env = env;
|
|
}
|
|
|
|
Title.prototype.makeLink = function () {
|
|
// XXX: links always point to the canonical namespace name.
|
|
if ( false && this.nskey ) {
|
|
return this.env.sanitizeURI( this.env.wgScriptPath +
|
|
this.nskey + ':' + this.key );
|
|
} else {
|
|
var l = this.env.wgScriptPath,
|
|
ns = this.ns.getDefaultName();
|
|
|
|
if ( ns ) {
|
|
l += ns + ':';
|
|
}
|
|
return this.env.sanitizeURI( l + this.key );
|
|
}
|
|
};
|
|
|
|
Title.prototype.getPrefixedText = function () {
|
|
// XXX: links always point to the canonical namespace name.
|
|
if ( this.nskey ) {
|
|
return this.env.sanitizeURI( this.nskey + ':' + this.key );
|
|
} else {
|
|
var ns = this.ns.getDefaultName();
|
|
|
|
if ( ns ) {
|
|
ns += ':';
|
|
}
|
|
return this.env.sanitizeURI( ns + this.key );
|
|
}
|
|
};
|
|
|
|
|
|
function Namespace ( id ) {
|
|
this.id = id;
|
|
}
|
|
|
|
Namespace.prototype._defaultNamespaceIDs = {
|
|
file: -2,
|
|
image: -2,
|
|
special: -1,
|
|
main: 0,
|
|
category: 14
|
|
};
|
|
|
|
Namespace.prototype._defaultNamespaceNames = {
|
|
'-2': 'File',
|
|
'-1': 'Special',
|
|
'0': '',
|
|
'14': 'Category'
|
|
};
|
|
|
|
Namespace.prototype.isFile = function ( ) {
|
|
return this.id === this._defaultNamespaceIDs.file;
|
|
};
|
|
Namespace.prototype.isCategory = function ( ) {
|
|
return this.id === this._defaultNamespaceIDs.category;
|
|
};
|
|
|
|
Namespace.prototype.getDefaultName = function ( ) {
|
|
if ( this.id == this._defaultNamespaceIDs.main ) {
|
|
return '';
|
|
} else {
|
|
return this._defaultNamespaceNames[this.id.toString()];
|
|
}
|
|
};
|
|
|
|
|
|
if (typeof module == "object") {
|
|
module.exports.Title = Title;
|
|
module.exports.Namespace = Namespace;
|
|
}
|