mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-09-23 18:28:51 +00:00
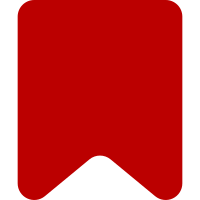
This is needed because the $params array is then passed on to ApiEditPage, so if the hook implementer wants to alter the data used with the edit, it needs to be able to modify the $params. See I494d72a42d9103c28c4d44077cfe0f1269fc7b00 for an example where GrowthExperiments would like to modify the 'tags' parameter for an edit. Depends-On: Idd052281898f99e4f13f241d5633294b59b29329 Bug: T304747 Change-Id: Ia4842a1593028f5fa145de167ccf9b72efa81351
89 lines
1.8 KiB
PHP
89 lines
1.8 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\VisualEditor;
|
|
|
|
/**
|
|
* VisualEditorHookRunner
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @copyright 2011-2021 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license MIT
|
|
*/
|
|
|
|
use MediaWiki\HookContainer\HookContainer;
|
|
use MediaWiki\Page\ProperPageIdentity;
|
|
use MediaWiki\User\UserIdentity;
|
|
use OutputPage;
|
|
use Skin;
|
|
|
|
class VisualEditorHookRunner implements
|
|
VisualEditorApiVisualEditorEditPreSaveHook,
|
|
VisualEditorApiVisualEditorEditPostSaveHook,
|
|
VisualEditorBeforeEditorHook
|
|
{
|
|
|
|
public const SERVICE_NAME = 'VisualEditorHookRunner';
|
|
|
|
/** @var HookContainer */
|
|
private $hookContainer;
|
|
|
|
/**
|
|
* @param HookContainer $hookContainer
|
|
*/
|
|
public function __construct( HookContainer $hookContainer ) {
|
|
$this->hookContainer = $hookContainer;
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function onVisualEditorApiVisualEditorEditPreSave(
|
|
ProperPageIdentity $page,
|
|
UserIdentity $user,
|
|
string $wikitext,
|
|
array &$params,
|
|
array $pluginData,
|
|
array &$apiResponse
|
|
) {
|
|
return $this->hookContainer->run( 'VisualEditorApiVisualEditorEditPreSave', [
|
|
$page,
|
|
$user,
|
|
$wikitext,
|
|
&$params,
|
|
$pluginData,
|
|
&$apiResponse
|
|
], [ 'abortable' => true ] );
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function onVisualEditorApiVisualEditorEditPostSave(
|
|
ProperPageIdentity $page,
|
|
UserIdentity $user,
|
|
string $wikitext,
|
|
array $params,
|
|
array $pluginData,
|
|
array $saveResult,
|
|
array &$apiResponse
|
|
): void {
|
|
$this->hookContainer->run( 'VisualEditorApiVisualEditorEditPostSave', [
|
|
$page,
|
|
$user,
|
|
$wikitext,
|
|
$params,
|
|
$pluginData,
|
|
$saveResult,
|
|
&$apiResponse
|
|
], [ 'abortable' => false ] );
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function onVisualEditorBeforeEditor(
|
|
OutputPage $output,
|
|
Skin $skin
|
|
): bool {
|
|
return $this->hookContainer->run( 'VisualEditorBeforeEditor', [
|
|
$output,
|
|
$skin
|
|
], [ 'abortable' => true ] );
|
|
}
|
|
}
|