mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
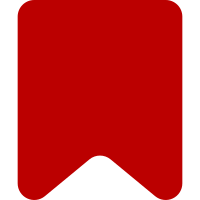
Us grouping the inheritable static properties that way is an implementation detail that is polluting the index and makes it harder to refer to individual identifiers. It also causes problems under JSDuck 5 because that version is more strict about defining properties (Foo.static.bar) of which the parent is not defined in the index (Foo.static), we'd have to add a sea of `@static @property {Object} this.static` all over the place. Might as well hide this implementation detail and just consider them static properties (just like we already do for "private" properties). Change-Id: Ibf2ebf7752aabc2b75b6ac6fa00e2284a181a600
66 lines
1.8 KiB
JavaScript
66 lines
1.8 KiB
JavaScript
/*!
|
|
* VisualEditor ContentEditable Annotation class.
|
|
*
|
|
* @copyright 2011-2013 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license The MIT License (MIT); see LICENSE.txt
|
|
*/
|
|
|
|
/**
|
|
* Generic ContentEditable annotation.
|
|
*
|
|
* This is an abstract class, annotations should extend this and call this constructor from their
|
|
* constructor. You should not instantiate this class directly.
|
|
*
|
|
* Subclasses of ve.dm.Annotation should have a corresponding subclass here that controls rendering.
|
|
*
|
|
* @abstract
|
|
* @extends ve.ce.View
|
|
*
|
|
* @constructor
|
|
* @param {ve.dm.Annotation} model Model to observe
|
|
* @param {ve.ce.ContentBranchNode} [parentNode] Node rendering this annotation
|
|
* @param {Object} [config] Configuration options
|
|
*/
|
|
ve.ce.Annotation = function VeCeAnnotation( model, parentNode, config ) {
|
|
// Parent constructor
|
|
ve.ce.View.call( this, model, config );
|
|
|
|
// Properties
|
|
this.parentNode = parentNode || null;
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( ve.ce.Annotation, ve.ce.View );
|
|
|
|
/* Static Properties */
|
|
|
|
ve.ce.Annotation.static.tagName = 'span';
|
|
|
|
/**
|
|
* Whether this annotation's continuation (or lack thereof) needs to be forced.
|
|
*
|
|
* This should be set to true only for annotations that aren't continued by browsers but are in DM,
|
|
* or the other way around, or those where behavior is inconsistent between browsers.
|
|
*
|
|
* @static
|
|
* @property
|
|
* @inheritable
|
|
*/
|
|
ve.ce.Annotation.static.forceContinuation = false;
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Get the content branch node this annotation is rendered in, if any.
|
|
* @returns {ve.ce.ContentBranchNode|null} Content branch node or null if none
|
|
*/
|
|
ve.ce.Annotation.prototype.getParentNode = function () {
|
|
return this.parentNode;
|
|
};
|
|
|
|
/** */
|
|
ve.ce.Annotation.prototype.getModelHtmlDocument = function () {
|
|
return this.parentNode && this.parentNode.getModelHtmlDocument();
|
|
};
|