mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/VisualEditor
synced 2024-11-15 18:39:52 +00:00
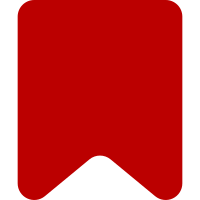
What: Add a hook that runs before a save attempt is made in ApiVisualEditorEdit. The hook receives the same data available in ApiVisualEditorEdit, and implementations of the hook can modify the API response. Why: VE plugins may send additional data when saving an edit, and extensions might want to prevent the save from taking place based on that additional data. See for example the AddLink plugin in Ic8225933c9, where the save is blocked if link suggestions don't exist in the database at save time. Bug: T283109 Change-Id: I6b81ea318f52ec47661086d85b5cc242a3fcd0e4
75 lines
1.5 KiB
PHP
75 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\VisualEditor;
|
|
|
|
/**
|
|
* VisualEditorHookRunner
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @copyright 2011-2021 VisualEditor Team and others; see AUTHORS.txt
|
|
* @license MIT
|
|
*/
|
|
|
|
use MediaWiki\HookContainer\HookContainer;
|
|
use MediaWiki\Page\ProperPageIdentity;
|
|
use MediaWiki\User\UserIdentity;
|
|
|
|
class VisualEditorHookRunner implements
|
|
VisualEditorApiVisualEditorEditPreSaveHook,
|
|
VisualEditorApiVisualEditorEditPostSaveHook
|
|
{
|
|
|
|
public const SERVICE_NAME = 'VisualEditorHookRunner';
|
|
|
|
/** @var HookContainer */
|
|
private $hookContainer;
|
|
|
|
/**
|
|
* @param HookContainer $hookContainer
|
|
*/
|
|
public function __construct( HookContainer $hookContainer ) {
|
|
$this->hookContainer = $hookContainer;
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function onVisualEditorApiVisualEditorEditPreSave(
|
|
ProperPageIdentity $page,
|
|
UserIdentity $user,
|
|
string $wikitext,
|
|
array $params,
|
|
array $pluginData,
|
|
array &$apiResponse
|
|
) {
|
|
return $this->hookContainer->run( 'VisualEditorApiVisualEditorEditPreSave', [
|
|
$page,
|
|
$user,
|
|
$wikitext,
|
|
$params,
|
|
$pluginData,
|
|
&$apiResponse
|
|
], [ 'abortable' => true ] );
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function onVisualEditorApiVisualEditorEditPostSave(
|
|
ProperPageIdentity $page,
|
|
UserIdentity $user,
|
|
string $wikitext,
|
|
array $params,
|
|
array $pluginData,
|
|
array $saveResult,
|
|
array &$apiResponse
|
|
): void {
|
|
$this->hookContainer->run( 'VisualEditorApiVisualEditorEditPostSave', [
|
|
$page,
|
|
$user,
|
|
$wikitext,
|
|
$params,
|
|
$pluginData,
|
|
$saveResult,
|
|
&$apiResponse
|
|
], [ 'abortable' => false ] );
|
|
}
|
|
}
|